Troubleshooting Common Issues in Migrating from Spring Security 5 to Spring Security 6
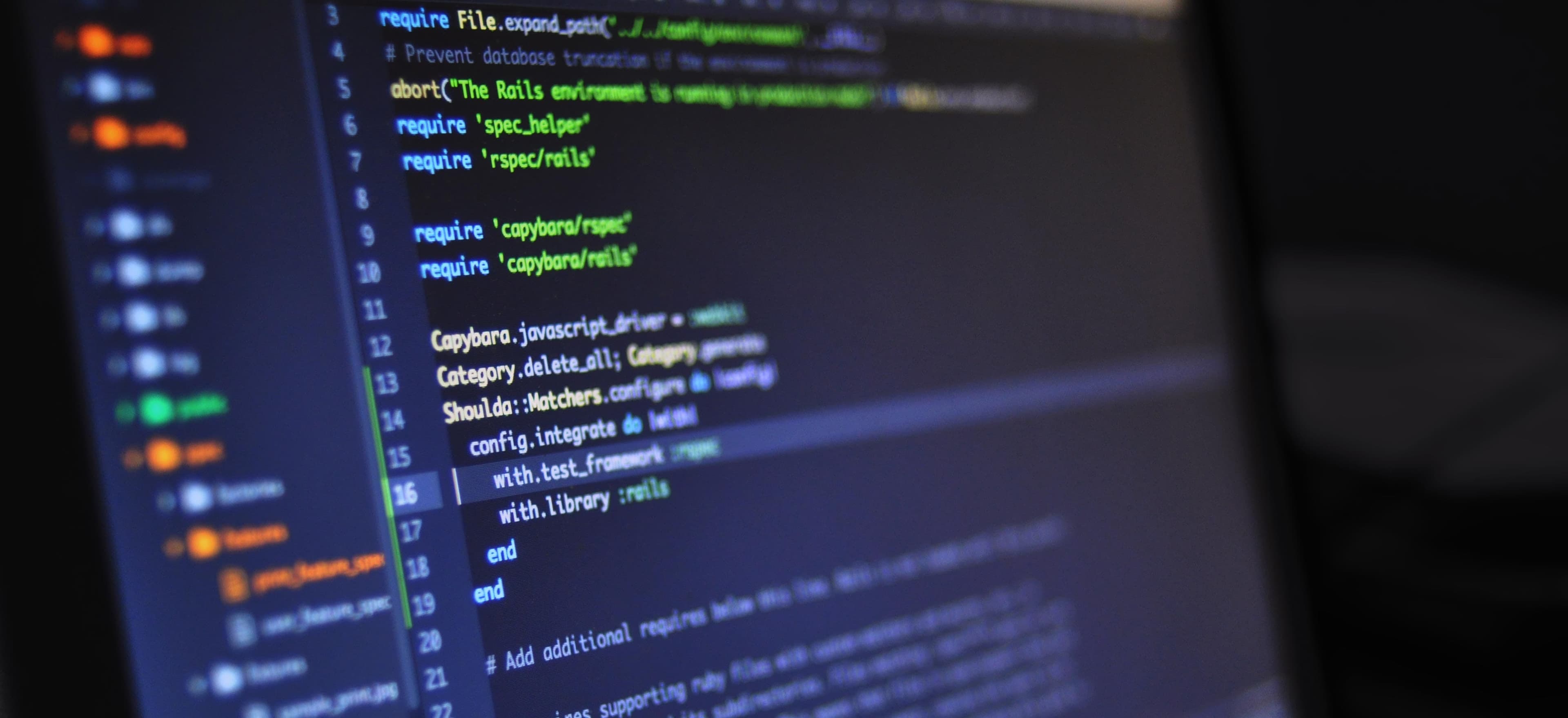
- Published on
Troubleshooting Common Issues in Migrating from Spring Security 5 to Spring Security 6
Spring Security is a powerful and highly customizable authentication and access control framework for Java applications. It provides comprehensive security solutions for Java EE-based enterprise software applications. Spring Security 6 brings in various new features and improvements, making it an attractive option for many developers.
However, migrating from Spring Security 5 to Spring Security 6 can sometimes lead to unexpected issues and challenges. In this blog post, we will discuss some common problems encountered during this migration process and how to troubleshoot them effectively.
Problem 1: Deprecated Classes and Methods
One of the most common issues when migrating from Spring Security 5 to Spring Security 6 is the presence of deprecated classes and methods. This can lead to compilation errors and runtime issues in the application.
Solution:
To resolve this issue, carefully review the migration guide, which provides a comprehensive list of deprecated classes and methods. Replace the deprecated classes and methods with their recommended alternatives as per the migration guide.
Here's an example of how to replace a deprecated method:
// Deprecated method in Spring Security 5
http.csrf().disable();
// Recommended alternative in Spring Security 6
http.csrf(csrf -> csrf.disable());
By following the migration guide and replacing deprecated classes and methods, you can ensure a smooth transition from Spring Security 5 to Spring Security 6.
Problem 2: Changes in Configuration Syntax
In Spring Security 6, there have been changes in the configuration syntax, leading to errors when using the old syntax from Spring Security 5.
Solution:
Review the official documentation for migrating from Spring Security 5 to Spring Security 6 to understand the changes in configuration syntax. Update the configuration files, such as SecurityConfig.java
, to use the new syntax as per the documentation.
For example, in Spring Security 5, the configuration to permit all requests might look like this:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/**").permitAll();
}
In Spring Security 6, the same configuration will use a slightly different syntax:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests(authorize -> authorize
.antMatchers("/**").permitAll()
);
}
By updating the configuration syntax as per the documentation, you can avoid errors and ensure the proper functioning of the security configurations in Spring Security 6.
Problem 3: Custom Authentication Provider Issues
If your application uses a custom authentication provider in Spring Security 5, you may encounter issues when migrating to Spring Security 6 due to changes in authentication provider interfaces or methods.
Solution:
Carefully review the authentication provider documentation for migrating from Spring Security 5 to Spring Security 6. Update your custom authentication provider implementation to adhere to the new interfaces and methods introduced in Spring Security 6.
For example, if your custom authentication provider implements the AuthenticationProvider
interface in Spring Security 5, you may need to update it to implement the AbstractUserDetailsAuthenticationProvider
interface in Spring Security 6.
// Spring Security 5
public class CustomAuthenticationProvider implements AuthenticationProvider {
// Implementation
}
// Spring Security 6
public class CustomAuthenticationProvider extends AbstractUserDetailsAuthenticationProvider {
// Updated implementation
}
By making the necessary changes to your custom authentication provider, you can ensure seamless integration with Spring Security 6.
Problem 4: Deprecation of SecurityContextHolder
In Spring Security 6, the SecurityContextHolder
class has been deprecated, and its usage may lead to warnings and potential issues in the application.
Solution:
Update the code that relies on the SecurityContextHolder
class to utilize the new approach recommended in Spring Security 6. Instead of directly accessing SecurityContextHolder
, consider using the SecurityContext
and Authentication
interfaces to access security-related information.
For example, in Spring Security 5, you might have used SecurityContextHolder.getContext().getAuthentication()
to retrieve the current authentication object. In Spring Security 6, you should use the following approach:
SecurityContext context = SecurityContextHolder.createEmptyContext();
Authentication authentication = context.getAuthentication();
By updating the code to replace direct usage of SecurityContextHolder
with the recommended approach, you can mitigate potential issues arising from the deprecation of SecurityContextHolder
in Spring Security 6.
A Final Look
Migrating from Spring Security 5 to Spring Security 6 can be a challenging task, especially when dealing with deprecated classes, changes in configuration syntax, custom authentication provider issues, and the deprecation of SecurityContextHolder
. By carefully reviewing the migration guides and documentation provided by Spring Security, and making the necessary updates to your codebase, you can successfully troubleshoot common issues and ensure a smooth transition to Spring Security 6.
Remember to always refer to the official documentation, utilize the migration guides, and stay updated with the latest best practices to make the most of Spring Security 6 and its new features.
Happy coding with Spring Security 6!