Scaling Challenges in Microservice Architecture
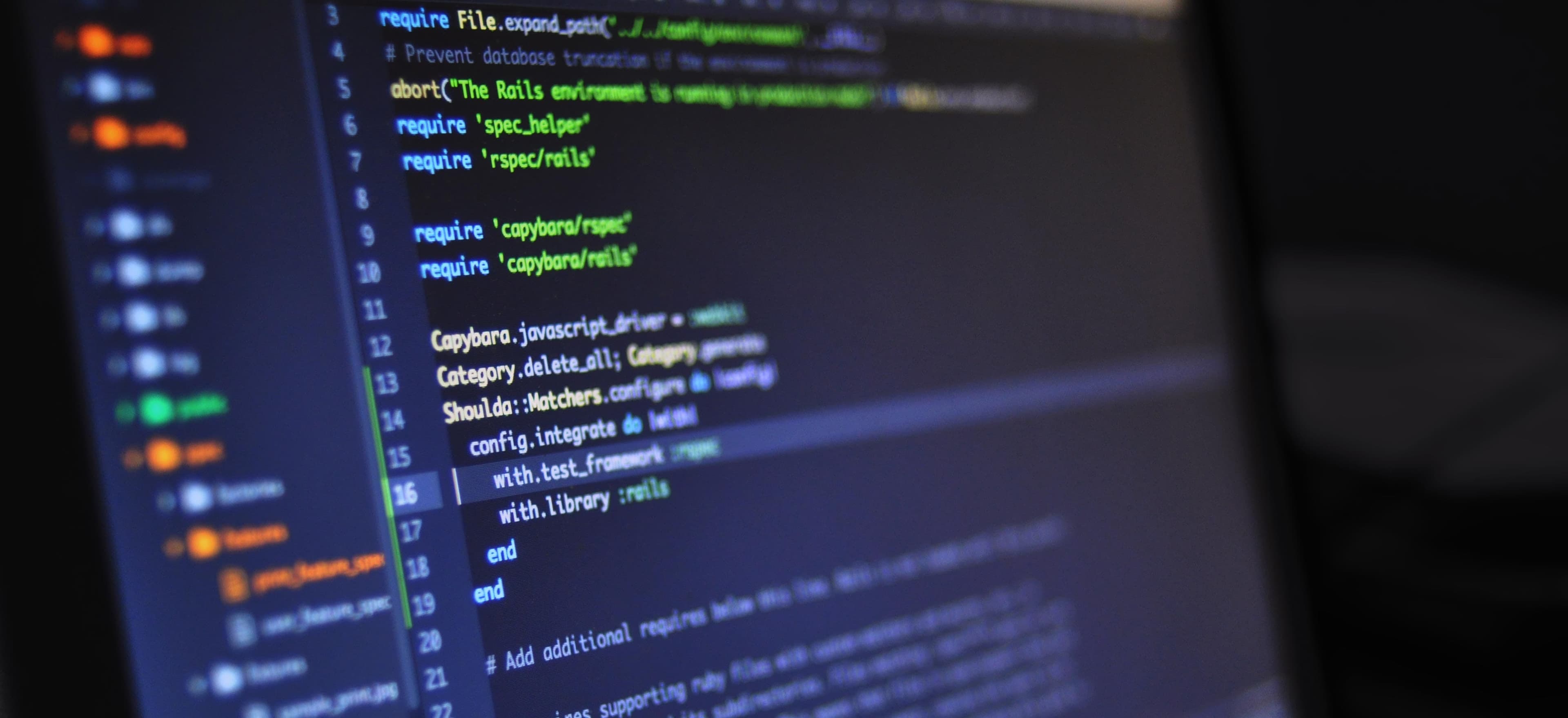
- Published on
Understanding Scaling Challenges in Microservice Architecture
Microservice architecture has gained immense popularity in recent years due to its ability to break down complex applications into smaller, easily manageable services. However, as the number of microservices grows, so do the challenges associated with scaling the architecture. In this blog post, we'll explore the scaling challenges in microservice architecture, discuss strategies to address them, and delve into how Java can be used to mitigate these challenges effectively.
The Scaling Challenge
1. Service Discovery and Load Balancing
With an increasing number of microservices, the task of service discovery and load balancing becomes more complex. Each microservice needs to discover the network locations of other microservices it depends on and distribute the incoming traffic efficiently across multiple instances of those services. This requires a robust service discovery and load balancing mechanism.
2. Data Consistency
Maintaining data consistency across multiple microservices is another major challenge. As microservices are autonomous and can have their own databases, ensuring that data remains consistent across services becomes crucial. In a scaled environment, achieving data consistency while maintaining high availability and partition tolerance (CAP theorem) becomes even more challenging.
3. Monitoring and Logging
As the number of microservices grows, monitoring and logging become essential for maintaining system health and diagnosing issues. However, managing logs and monitoring data from a large number of services and instances can be overwhelming. It's important to have a centralized monitoring and logging solution that can handle the scale of microservices effectively.
Addressing the Challenges with Java
1. Service Discovery and Load Balancing
Using Netflix Eureka
Netflix Eureka is a popular tool for implementing service discovery in a microservices architecture. It allows services to register themselves and discover other services via a REST API. In Java, you can easily integrate Eureka into your microservices using Spring Cloud, which provides seamless support for service registration and discovery.
Exemplary Code Snippet:
// Service registration with Eureka using Spring Cloud
@EnableEurekaClient
@SpringBootApplication
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
}
In this code snippet, the @EnableEurekaClient
annotation enables the microservice to register with Eureka, allowing other services to discover it.
2. Data Consistency
Implementing Distributed Transactions with Sagas
Sagas are a pattern for managing distributed transactions within a microservices architecture. In Java, you can leverage frameworks like Axon or Eventuate to implement sagas, which orchestrate the sequence of local transactions in each microservice to ensure data consistency across the system.
Exemplary Code Snippet:
// Saga orchestration using Axon framework
@Saga
public class OrderProcessSaga {
@StartSaga
@SagaEventHandler(associationProperty = "orderId")
public void handle(OrderCreatedEvent event) {
// Start the saga and initiate local transactions
}
// More saga event handlers to manage the transaction flow
}
By using the Axon framework, you can define sagas to coordinate the local transactions of each microservice involved in a distributed transaction, ensuring eventual data consistency.
3. Monitoring and Logging
Leveraging Prometheus and Grafana
Prometheus is a popular open-source monitoring and alerting tool, and Grafana is a widely used analytics and visualization platform. In a Java-based microservices environment, you can instrument your services with Prometheus client libraries and set up Grafana dashboards to monitor and visualize the system metrics effectively.
Exemplary Code Snippet:
// Instrumenting a microservice with Prometheus client
public class OrderService {
private static final Counter ordersProcessed = Counter.build()
.name("orders_processed_total")
.help("Total number of processed orders")
.register();
public void processOrder(Order order) {
// Process the order
ordersProcessed.inc();
}
}
By using the Prometheus client library in Java, you can expose custom metrics like the total number of processed orders, which can be visualized in Grafana dashboards for monitoring.
Closing the Chapter
Scaling a microservice architecture presents various challenges, including service discovery and load balancing, data consistency, and monitoring/logging. However, by leveraging the right tools and frameworks in Java, such as Netflix Eureka for service discovery, sagas for data consistency, and Prometheus/Grafana for monitoring, these challenges can be effectively addressed.
In conclusion, understanding the scaling challenges in microservice architecture and adopting appropriate strategies in Java is crucial for building resilient and scalable microservices-based systems.
Remember, as your microservice architecture scales, so should your approach to addressing these challenges - with the right tools and strategies in place, you can ensure a robust and scalable system.
Checkout our other articles