Breaking Down Amdahl's Law: Overcoming Serial Bottlenecks
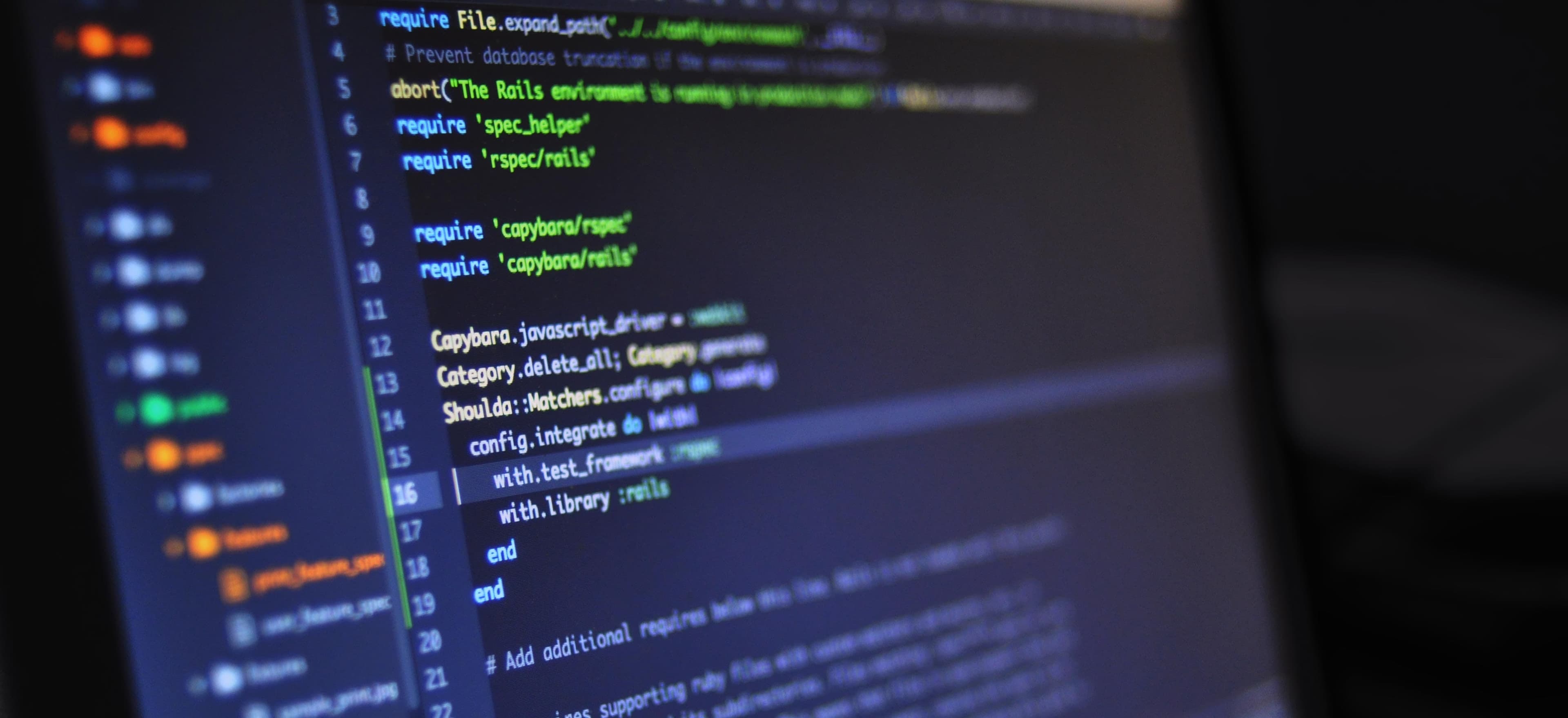
- Published on
Breaking Down Amdahl's Law: Overcoming Serial Bottlenecks
In the world of software development, the scalability and efficiency of code are paramount. It's not uncommon to encounter bottlenecks that hinder the overall performance of an application. Amdahl's Law addresses this very issue, offering a theoretical framework for understanding the potential performance improvements when addressing bottlenecks in parallel computing.
Understanding Amdahl's Law
In 1967, Gene Amdahl, a prominent computer architect, introduced Amdahl's Law as a formula to calculate the maximum expected improvement to an overall system when only part of the system is improved. The law revolves around the concept of parallel computing and aims to provide insights into the potential gains from parallelizing a computing task.
According to Amdahl's Law, the speedup of a program using multiple processors in parallel computing is limited by the sequential fraction of the program. In simpler terms, it highlights that even with an infinite number of processors, the overall speedup of a program will be constrained by the parts of the program that cannot be parallelized.
The Formula
Amdahl's Law is encapsulated in the following formula:
Speedup(S) = 1 / [(1 - P) + (P / N)]
Where:
- S = Speedup
- P = Percentage of the program that can be parallelized
- N = Number of processors
This formula serves as a guide to understand the limitations and potential gains of implementing parallel computing for a specific program or algorithm.
Insightful Code Implementation
To better grasp the practical implications of Amdahl's Law, consider the following Java code snippet:
public class AmdahlsLaw {
public static void main(String[] args) {
double parallelizableFraction = 0.8; // 80% of the program can be parallelized
int numProcessors = 4; // Number of processors
double speedup = 1 / ((1 - parallelizableFraction) + (parallelizableFraction / numProcessors));
System.out.println("Speedup: " + speedup);
}
}
This code snippet calculates the speedup based on the percentage of the program that can be parallelized and the number of processors. Running this code with different values for parallelizableFraction
and numProcessors
allows understanding the impact of these parameters on the speedup.
Overcoming Bottlenecks Through Parallelization
Amdahl's Law underscores the importance of identifying and addressing bottlenecks in a program to achieve optimal performance. While it emphasizes the limitations imposed by sequential portions of a program, it also sheds light on the potential gains from parallelization.
In practical terms, overcoming bottlenecks through parallelization involves dissecting the program to identify parts that can be executed in parallel. This often involves leveraging multithreading or distributed computing to distribute the workload across multiple processors or cores.
Let’s dive into a real-world example. Suppose we have a computationally intensive task, such as image processing, where certain parts of the algorithm can be executed independently. By identifying these parallelizable sections and leveraging parallel computing techniques, we can significantly improve the overall performance of the image processing task.
Leveraging Java for Parallel Computing
Java offers robust support for parallel computing through its java.util.concurrent
package, which provides high-level concurrency utilities. The package includes features such as Executor Framework, Fork/Join Framework, and concurrent data structures that facilitate parallel execution of tasks.
The following example demonstrates the usage of Java's Executor Framework to parallelize a set of tasks:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelComputingExample {
public static void main(String[] args) {
int numTasks = 10;
ExecutorService executor = Executors.newFixedThreadPool(4); // Creating a thread pool with 4 threads
for (int i = 0; i < numTasks; i++) {
executor.execute(new Task(i)); // Executing tasks in parallel
}
executor.shutdown();
}
}
class Task implements Runnable {
private int taskId;
public Task(int taskId) {
this.taskId = taskId;
}
public void run() {
System.out.println("Task " + taskId + " is being processed.");
}
}
In this example, a pool of threads is created using Executors.newFixedThreadPool
, and tasks are executed in parallel using the execute
method. This showcases how Java's concurrency utilities can be utilized to parallelize tasks, thereby effectively addressing bottlenecks and improving overall performance.
Bringing It All Together
Amdahl's Law serves as a valuable tool for understanding the limitations of parallel computing and the potential impacts of addressing bottlenecks in a program. By identifying the sequential fraction of a program and leveraging parallelization techniques, developers can work towards optimizing the performance of their software.
In essence, Amdahl's Law highlights the significance of efficient parallelization in achieving performance improvements. It urges developers to scrutinize their code, identify opportunities for parallel execution, and leverage appropriate technologies, such as Java's concurrency utilities, to overcome serial bottlenecks and unlock the full potential of their applications.