Dynamic Entity Graphs: Fine-tuning Lazy Loading
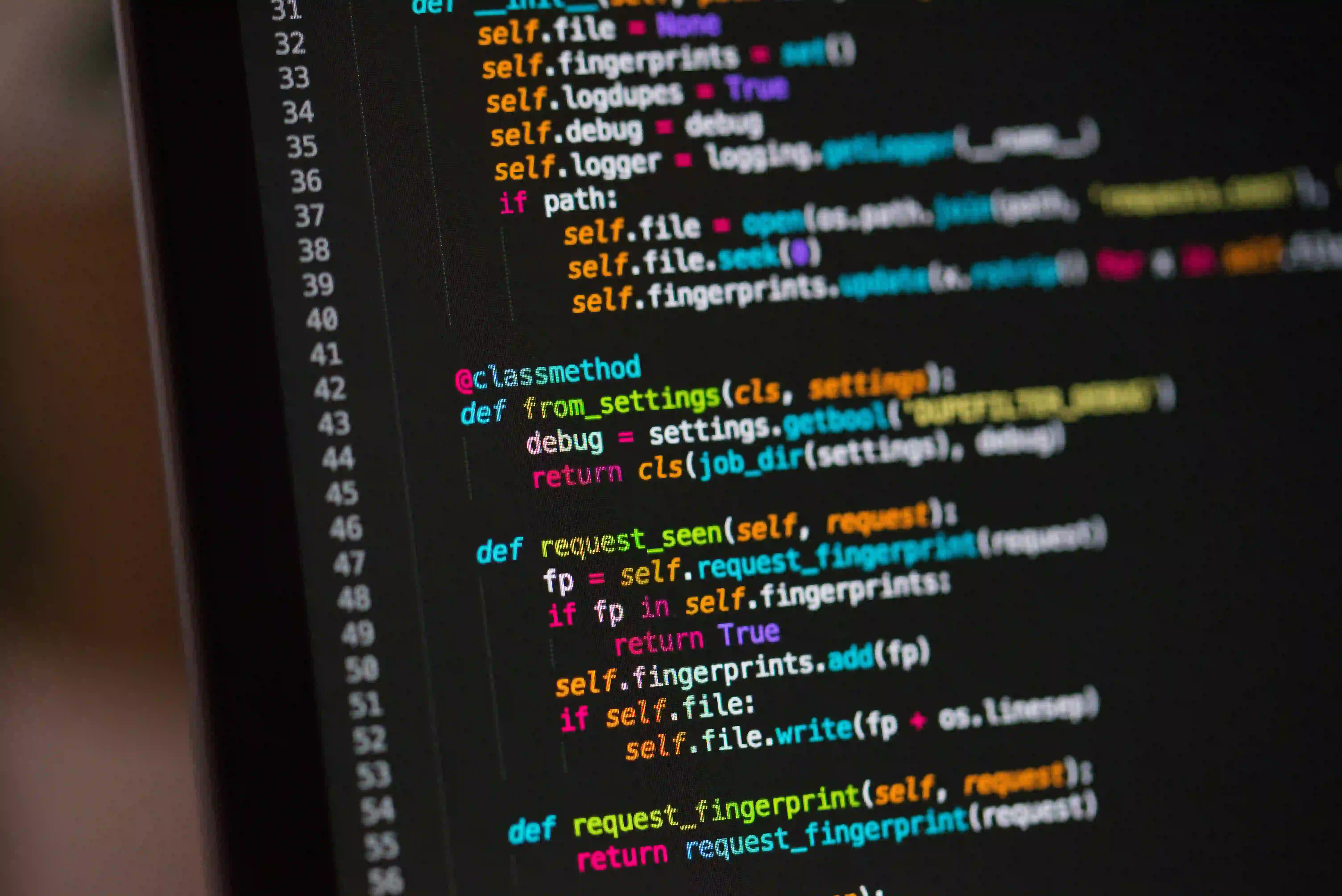
Dynamic Entity Graphs: Fine-tuning Lazy Loading
In the realm of Java Persistence, the ability to optimize lazy loading promises remarkable performance improvements. By employing dynamic entity graphs, developers can selectively load only the necessary data, streamlining the application and enhancing its overall efficiency.
Understanding Lazy Loading
Lazy loading is a popular optimization technique within Java Persistence frameworks such as Hibernate and JPA. It defers the loading of an entity's related data until the point when that data is explicitly accessed. This strategy is particularly beneficial when dealing with complex, interconnected data models.
Consider a scenario where you have an Order
entity associated with multiple OrderItem
entities. Without lazy loading, all associated OrderItem
data would be fetched whenever an Order
object is retrieved, potentially resulting in unnecessary database calls and inflated memory consumption.
The Role of Entity Graphs
Entity graphs, introduced in JPA 2.1, enable developers to define precisely which attributes and associations of an entity should be loaded. This capability eliminates the need for default eager or lazy loading settings, providing fine-grained control over data retrieval.
Implementing Dynamic Entity Graphs
The process of implementing dynamic entity graphs involves creating entity graph instances at runtime, tailored to the specific requirements of the application. Let's consider an example of how this can be achieved using code snippets.
Defining the Entity
@Entity
public class Order implements Serializable {
@Id
private Long id;
private String customerName;
@OneToMany(mappedBy = "order", cascade = CascadeType.ALL)
private List<OrderItem> items;
// Getters and setters
}
Fetching Data with a Dynamic Entity Graph
EntityGraph<Order> graph = entityManager.createEntityGraph(Order.class);
Subgraph<OrderItem> itemGraph = graph.addSubgraph("items");
TypedQuery<Order> query = entityManager.createQuery("SELECT o FROM Order o WHERE o.id = :orderId", Order.class);
query.setParameter("orderId", 123L);
query.setHint("javax.persistence.fetchgraph", graph);
Order order = query.getSingleResult();
In this example, we create an entity graph at runtime and use it to fetch an Order
object with its associated OrderItem
data. By specifying the entity graph as a hint to the query, we direct the persistence provider to load only the attributes and associations mapped within the graph.
The Benefits of Dynamic Entity Graphs
Dynamic entity graphs offer several advantages:
- Reduced Data Overhead: By loading only the required attributes and associations, unnecessary data retrieval is avoided, leading to improved performance.
- Fine-tuned Control: Developers can precisely define which parts of the entity graph should be loaded based on the specific use case, enhancing flexibility.
- Improved Maintainability: As entity graphs are defined programmatically, modifications can be made without altering the entity mappings, facilitating maintenance.
Final Considerations
Dynamic entity graphs present a compelling solution for fine-tuning lazy loading in Java Persistence. By leveraging this capability, developers can optimize data retrieval, minimize overhead, and enhance the overall performance of their applications. Incorporating dynamic entity graphs into the development workflow empowers teams to take control of data loading strategies, resulting in more efficient and maintainable solutions.
To delve deeper into dynamic entity graphs and the opportunities they present, explore the official Java Persistence documentation. Additionally, for practical insights and real-world examples, refer to the insightful blog posts on Baeldung that discuss the practical implementations and benefits of dynamic entity graphs in Java applications.