Maximizing Test Coverage for Mobile Software
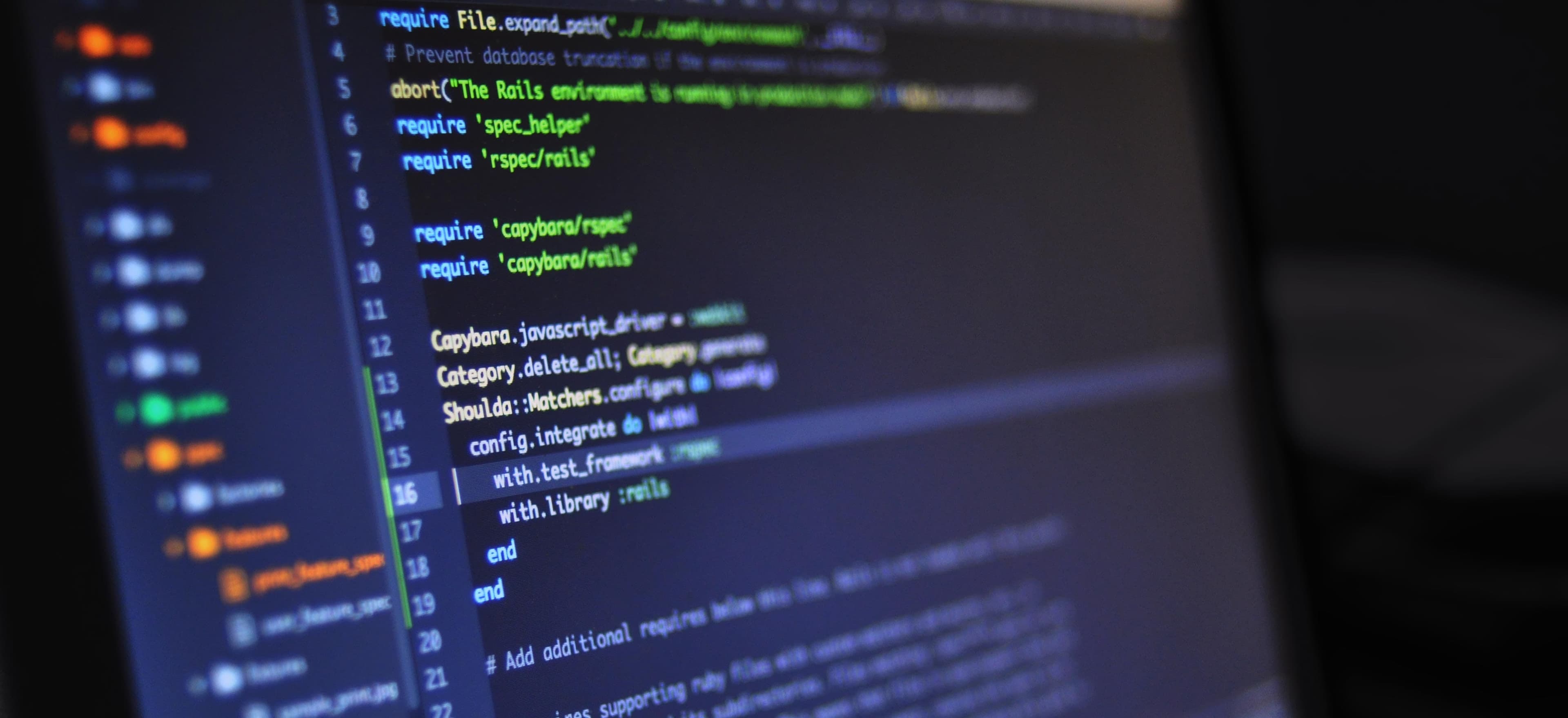
- Published on
Maximizing Test Coverage for Mobile Software
Testing is a critical aspect of the software development lifecycle and is particularly crucial in the mobile application domain. With an abundance of mobile devices, manufacturers, and operating system versions, ensuring comprehensive test coverage is challenging yet essential for delivering a high-quality mobile software product. In this article, we’ll explore strategies for maximizing test coverage for mobile software, with a specific focus on Java-based mobile applications.
Importance of Test Coverage
Test coverage measures the extent to which the source code of a software program has been tested. It is a critical metric that indicates the effectiveness of testing and helps identify areas that require additional attention. For mobile software, comprehensive test coverage is imperative due to the following reasons:
Diverse Device Landscape
The market is flooded with a wide array of mobile devices with varying screen sizes, resolutions, processing power, and hardware configurations. Testing on a diverse set of devices ensures that the application behaves consistently across different platforms.
Operating System Fragmentation
The Android ecosystem, in particular, is highly fragmented with numerous OS versions and customizations by device manufacturers. Testing across multiple OS versions is essential to uncover compatibility issues and ensure a smooth user experience.
User Experience and Expectations
Mobile users have high expectations regarding the performance, usability, and reliability of applications. Comprehensive testing is crucial for meeting or exceeding these expectations and retaining user satisfaction.
Strategies for Maximizing Test Coverage
Automated Testing
Automated testing is a cornerstone of maximizing test coverage. It enables the execution of test scenarios across a wide range of devices and OS versions, significantly enhancing the breadth of test coverage.
@Test
public void loginTest() {
// Test logic for user login
// Assertions for successful login
}
In the above example, a basic unit test for a login functionality is demonstrated. By automating such test cases, developers can ensure consistent behavior across different devices and OS versions.
Embracing Test-Driven Development (TDD)
Test-Driven Development (TDD) promotes writing tests before the actual code implementation. By following this approach, developers can ensure that every line of code has a corresponding test, effectively increasing test coverage.
User Interface Testing
Mobile applications often have complex user interfaces that require thorough testing. Utilizing frameworks like Appium for automated UI testing ensures comprehensive coverage of the application’s visual components and user interactions.
Utilizing Cloud-Based Testing Platforms
Cloud-based testing platforms such as AWS Device Farm and Firebase Test Lab provide access to a vast library of real mobile devices for automated testing. Leveraging these platforms enables testing across a broad spectrum of devices, thereby enhancing test coverage.
@Test
public void cartCheckoutTest() {
// Automated test for the checkout process
// Run on multiple devices using cloud-based testing platform
// Assertions for successful checkout
}
By running tests on diverse real devices through cloud-based platforms, developers can address compatibility issues and ensure a consistent user experience across devices.
Exploratory Testing
In addition to automated testing, exploratory testing conducted by QA professionals helps uncover unforeseen issues and edge cases that might be missed by automated test scenarios. This approach complements automated testing and contributes to comprehensive test coverage.
Leveraging Java for Mobile Test Coverage
Java, with its robust ecosystem and mature testing frameworks, is well-suited for maximizing test coverage in the mobile software domain. Notable tools and frameworks include:
Appium
Appium is an open-source automation tool for testing native, hybrid, and mobile web applications. It supports multiple programming languages, including Java, and enables testing across various mobile platforms.
// Example Appium test in Java
WebElement element = driver.findElement(By.id("loginButton"));
element.click();
// Assertions for login functionality
Using Appium with Java allows developers to write concise and reliable tests for mobile applications, contributing to comprehensive test coverage.
Espresso
Espresso is a testing framework for Android that is specifically designed to make UI testing smooth and reliable. It provides APIs for writing concise and readable UI tests in Java.
// Example Espresso test in Java
onView(withId(R.id.loginButton)).perform(click());
// Assertions for login functionality
By leveraging Espresso, developers can ensure robust test coverage of the user interface aspects of their Android applications.
JUnit and TestNG
JUnit and TestNG are popular Java testing frameworks that enable the creation of unit tests and integration tests for mobile applications. These frameworks provide a structured approach to test development and execution.
@Test
public void addToCartTest() {
// Test logic to add items to the cart
// Assertions for successful addition
}
By utilizing JUnit and TestNG, developers can create comprehensive test suites for their mobile applications, covering various functional aspects.
Closing Remarks
Maximizing test coverage for mobile software is a multifaceted endeavor that demands a strategic combination of automated testing, manual testing, and the right set of tools and frameworks. In the Java ecosystem, leveraging robust testing frameworks such as JUnit, TestNG, Appium, and Espresso is instrumental in achieving comprehensive test coverage for mobile applications. By embracing a holistic approach to testing and incorporating diverse strategies, developers can ensure the delivery of high-quality mobile software that meets the dynamic demands of the modern mobile landscape.
In conclusion, investing in test coverage is not just about identifying bugs - it's about building trust and delivering an exceptional user experience.
By implementing comprehensive test coverage strategies, mobile software developers stand poised to deliver high-quality applications in a competitive and dynamic landscape.