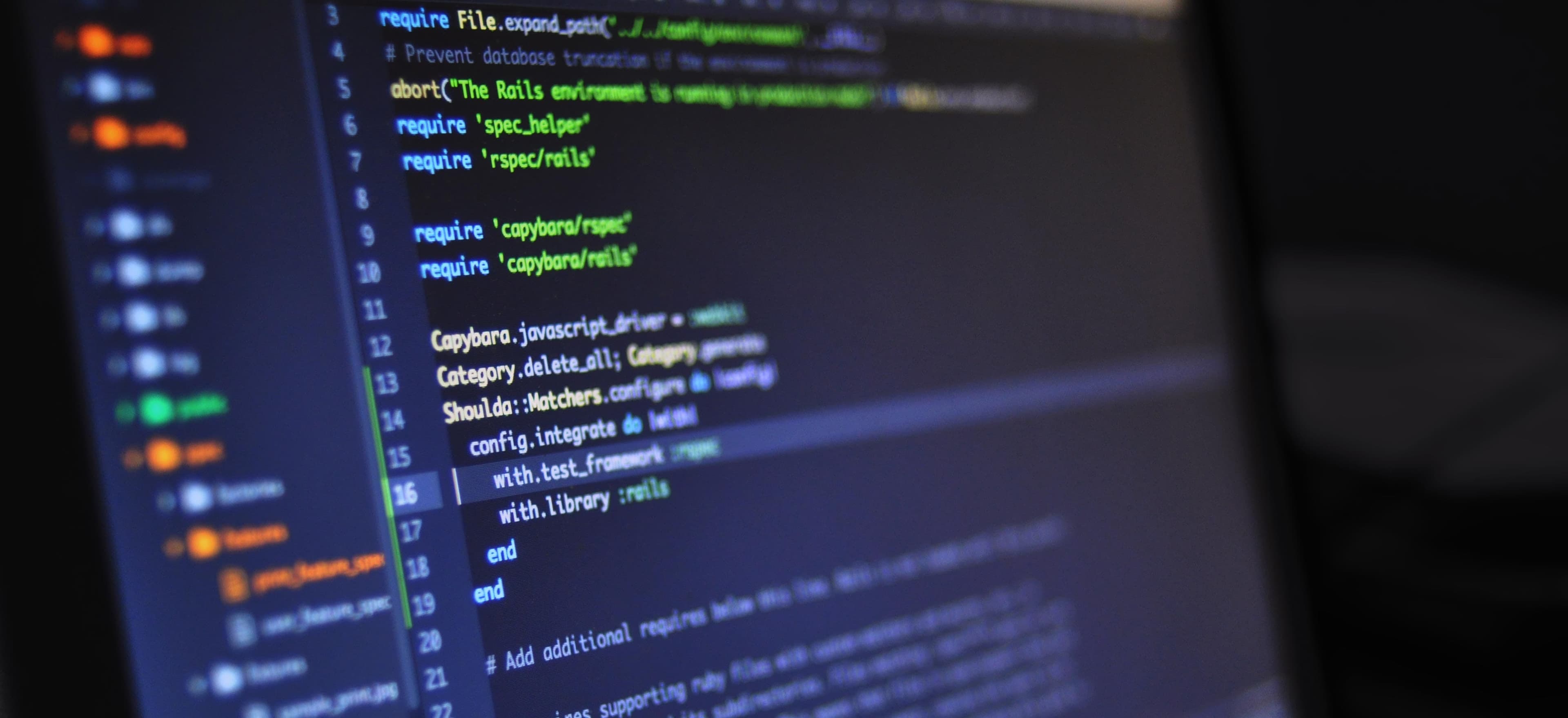
- Published on
Mastering Android UI Views: A Complete Guide
When it comes to building robust and visually appealing Android apps, mastering the various UI views is essential. In this comprehensive guide, we'll delve into the intricacies of UI views in Android, covering everything from the fundamentals to advanced techniques. By the end of this article, you'll have a solid understanding of how to leverage UI views effectively to create stunning and user-friendly Android applications.
Understanding Views and ViewGroups
At the core of Android UI development are Views and ViewGroups. Views represent the basic building blocks of UI components such as buttons, text fields, and images, while ViewGroups are containers that hold Views and other ViewGroups. Understanding the hierarchy and interaction between Views and ViewGroups is crucial for crafting a well-structured and responsive UI.
// Example of creating a TextView programmatically
TextView textView = new TextView(context);
textView.setText("Hello, World!");
In the code snippet above, we create a TextView
dynamically and set its text. This demonstrates the flexibility of Views in Android, as they can be instantiated and configured both programmatically and through XML layout files.
Leveraging XML Layouts for UI Design
XML layouts play a pivotal role in defining the structure and appearance of Android UIs. They offer a declarative way to design UI components, allowing developers to define Views and their properties in a clear and organized manner. By utilizing XML layouts, developers can achieve consistency across different screen sizes and device orientations.
<!-- Example of a simple XML layout defining a TextView -->
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, XML Layouts!"
android:textSize="24sp"/>
</LinearLayout>
The above XML layout code snippet showcases a LinearLayout
containing a TextView
with specified layout attributes. This approach provides a clear representation of the UI structure and properties.
Custom Views for Specialized UI Elements
In certain scenarios, the standard set of Android Views might not fully meet the requirements of a particular UI element. This is where custom Views come into play. By extending existing Views or implementing custom drawing logic, developers can create specialized UI components tailored to their specific needs.
// Example of a custom View with overridden onDraw method for custom drawing
public class CustomView extends View {
// Constructor and other methods omitted for brevity
@Override
protected void onDraw(Canvas canvas) {
// Custom drawing logic using Canvas
}
}
The custom View illustrated above demonstrates the potential for creating unique UI elements in Android. Whether it's a custom progress indicator or a complex visualization, custom Views empower developers to craft bespoke UI components.
Handling User Interaction with Views
User interaction is a crucial aspect of any Android app, and Views play a central role in facilitating such interaction. Whether it's responding to button clicks, touch gestures, or text input, handling user interaction effectively is essential for delivering a seamless user experience.
// Example of attaching a click listener to a Button
Button button = findViewById(R.id.my_button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button click
}
});
In the code snippet above, we set a click listener for a Button
to execute specific actions when the button is clicked. This exemplifies the fundamental approach to handling user interaction in Android.
Advanced View Techniques: Custom Drawing and Animations
Beyond the standard use cases, advanced UI scenarios often call for custom drawing and animations. By harnessing the drawing capabilities of the Android Canvas and leveraging animation APIs, developers can create visually captivating and dynamic user interfaces.
// Example of animating a View using ObjectAnimator
ObjectAnimator animator = ObjectAnimator.ofFloat(view, "translationX", 0f, 100f);
animator.setDuration(1000);
animator.start();
The code snippet above demonstrates the use of ObjectAnimator
to animate the translation of a View. This kind of animation can breathe life into UI elements and enhance the overall user experience.
Optimizing Performance and Responsiveness
As the complexity of Android UIs grows, ensuring optimal performance and responsiveness becomes increasingly important. Techniques such as optimizing layout hierarchies, recycling Views in RecyclerViews, and leveraging hardware acceleration are crucial for delivering smooth and efficient UI interactions.
// Example of optimizing RecyclerView performance with ViewHolder pattern
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> {
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_layout, parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
// Bind data to Views
}
static class ViewHolder extends RecyclerView.ViewHolder {
ViewHolder(View itemView) {
super(itemView);
// Initialize Views
}
}
}
In the above snippet, the ViewHolder pattern is employed to optimize the performance of a RecyclerView by reusing Views efficiently. This approach minimizes unnecessary View recreation and contributes to a smoother scrolling experience.
Embracing Material Design and Adaptive UI
With the evolution of Android app design, adhering to Google's Material Design guidelines and embracing adaptive UI principles has become paramount. Leveraging Material Components and designing adaptive layouts ensures that apps not only look modern but also provide a consistent and intuitive user experience across diverse Android devices.
<!-- Example of using Material Components in XML layout -->
<com.google.android.material.button.MaterialButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Material Button"/>
The use of MaterialButton
in the above XML layout aligns with the Material Design principles, offering a visually cohesive and interactive UI element.
Lessons Learned
Mastering Android UI Views is integral to delivering captivating and user-friendly apps. From understanding the fundamentals of Views and ViewGroups to incorporating advanced techniques like custom drawing and animations, a solid grasp of UI views is indispensable for Android developers. By optimizing performance, embracing modern design principles, and prioritizing user interaction, developers can create engaging and seamless Android apps that resonate with users.
In conclusion, honing your skills in Android UI Views empowers you to craft remarkable user experiences and set your apps apart in the competitive Android ecosystem.
For further reading and exploration, you can refer to the official Android Developer documentation on UI and Material Design to deepen your understanding of Android UI development and design principles.