Extending Spark SQL with Custom Data Sources
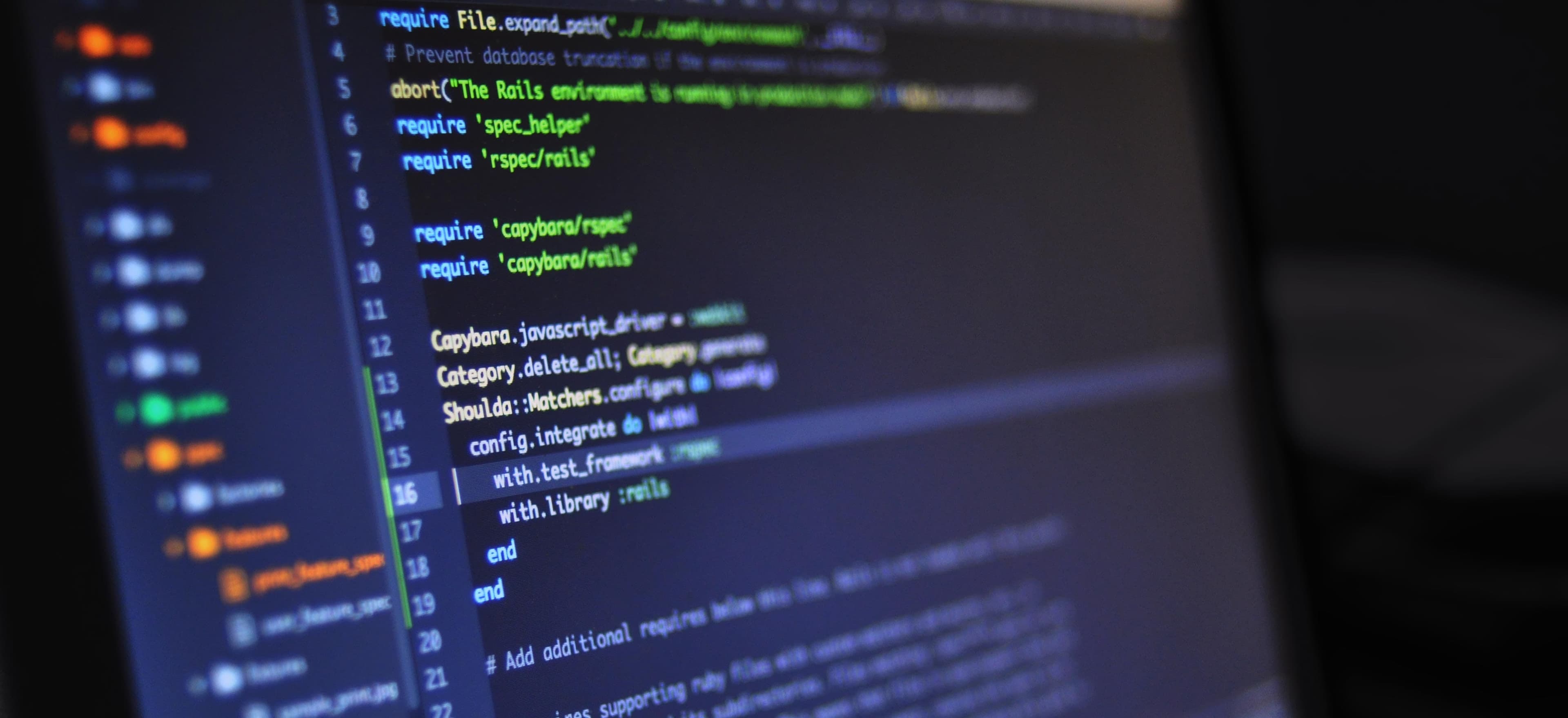
- Published on
Extending Spark SQL with Custom Data Sources
Apache Spark is a powerful open-source distributed computing system known for its speed and ease of use. It provides a unified analytics engine for big data processing with built-in modules for SQL, streaming, machine learning, and graph processing. One of the key components of Spark is Spark SQL, which allows querying structured data inside Spark programs.
In this blog post, we will explore how to extend Spark SQL with custom data sources. We will walk through the process of creating a custom data source using Java, integrating it with Spark SQL, and performing basic operations to demonstrate its functionality.
Why Extend Spark SQL with Custom Data Sources?
Spark SQL comes with built-in support for various data formats and storage systems such as Parquet, JSON, CSV, Apache Hive, and more. However, there are cases where you may need to work with data from a custom source that is not natively supported by Spark SQL.
By extending Spark SQL with custom data sources, you can seamlessly integrate external data systems or formats and leverage the full power of Spark SQL's optimization and execution engine for processing and analyzing custom data.
Creating a Custom Data Source in Java
To create a custom data source for Spark SQL in Java, we need to implement the DataSourceV2 interface provided by Apache Spark. This interface allows us to define a custom data source that can be used with Spark SQL.
Let's start by creating a simple custom data source that generates a sequence of numbers. We will define a schema for the data source, implement the necessary interfaces, and then use it with Spark SQL.
Step 1: Define the Schema
We'll start by defining the schema for our custom data source. In this example, our data source will have a single column named "value" of type Integer.
import org.apache.spark.sql.connector.catalog.Table;
import org.apache.spark.sql.connector.catalog.TableCapability;
import org.apache.spark.sql.connector.catalog.SupportsRead;
import org.apache.spark.sql.connector.read.Scan;
import org.apache.spark.sql.connector.read.InputPartition;
import org.apache.spark.sql.connector.read.InputPartitionReader;
import org.apache.spark.sql.types.StructType;
public class NumberDataSourceTable implements Table, SupportsRead {
@Override
public StructType schema() {
return new StructType().add("value", "int");
}
// Other Table and SupportsRead methods implementation...
}
In the above code, we define a class NumberDataSourceTable that implements the Table and SupportsRead interfaces. We define the schema using the schema() method, which returns a StructType representing the schema of our custom data source.
Step 2: Implement the Data Source
Next, we'll implement the actual data source logic. We'll create a custom data source reader that generates a sequence of numbers as rows.
import java.util.List;
import org.apache.spark.sql.connector.read.PartitionReaderFactory;
import org.apache.spark.sql.connector.read.ScanBuilder;
import org.apache.spark.sql.connector.read.SupportsReportPartitioning;
import org.apache.spark.sql.sources.DataSourceRegister;
import org.apache.spark.sql.types.StructType;
public class NumberDataSource implements DataSourceV2, DataSourceRegister {
@Override
public StructType readSchema() {
return new StructType().add("value", "int");
}
// Other DataSourceV2 and DataSourceRegister methods implementation...
}
In the above code, we define a class NumberDataSource that implements the DataSourceV2 and DataSourceRegister interfaces. We define the schema using the readSchema() method, which returns the schema of our custom data source.
Step 3: Register the Data Source
Finally, we need to register our custom data source so that Spark SQL can recognize and use it.
import org.apache.spark.sql.connector.catalog.TableProvider;
import org.apache.spark.sql.sources.DataSourceRegister;
import java.util.Map;
public class NumberDataSourceProvider implements TableProvider, DataSourceRegister {
@Override
public StructType inferSchema(Map<String, String> options) {
return new StructType().add("value", "int");
}
// Other TableProvider and DataSourceRegister methods implementation...
}
In the above code, we define a class NumberDataSourceProvider that implements the TableProvider and DataSourceRegister interfaces. We define the schema using the inferSchema() method, which returns the schema of our custom data source.
Integrating Custom Data Source with Spark SQL
Now that we have created our custom data source, let's integrate it with Spark SQL and perform some basic operations to demonstrate its functionality.
Step 1: Register the Custom Data Source
We need to register our custom data source so that Spark SQL can use it to read and write data.
import org.apache.spark.sql.SparkSession;
public class Main {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("CustomDataSourceExample")
.master("local")
.getOrCreate();
spark.conf().set("spark.sql.sources.default", "custom-number-datasource");
// Other data source registration and operations...
}
}
In the above code, we register our custom data source with the name "custom-number-datasource" using the SparkSession configuration.
Step 2: Read Data from the Custom Data Source
Now, let's use our custom data source to read data into a DataFrame and perform some operations.
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
public class Main {
public static void main(String[] args) {
// SparkSession initialization...
// Read data from the custom data source
Dataset<Row> numbers = spark.read()
.format("custom-number-datasource")
.load();
numbers.show();
// Other data source operations...
}
}
In the above code, we use the "custom-number-datasource" format to load data into a DataFrame. We then display the contents of the DataFrame using the show() method.
Key Takeaways
In this blog post, we have explored the process of extending Spark SQL with custom data sources using Java. We created a simple custom data source, integrated it with Spark SQL, and performed basic operations to demonstrate its functionality.
By extending Spark SQL with custom data sources, you can seamlessly work with data from external systems or formats that are not natively supported by Spark SQL, thereby unlocking the full potential of Spark for your data processing needs.
For further information on extending Spark SQL with custom data sources, you can refer to the official documentation and explore more advanced use cases and optimizations.
Happy coding with Spark SQL custom data sources!