Optional in Java 8: Handling Null Values efficiently
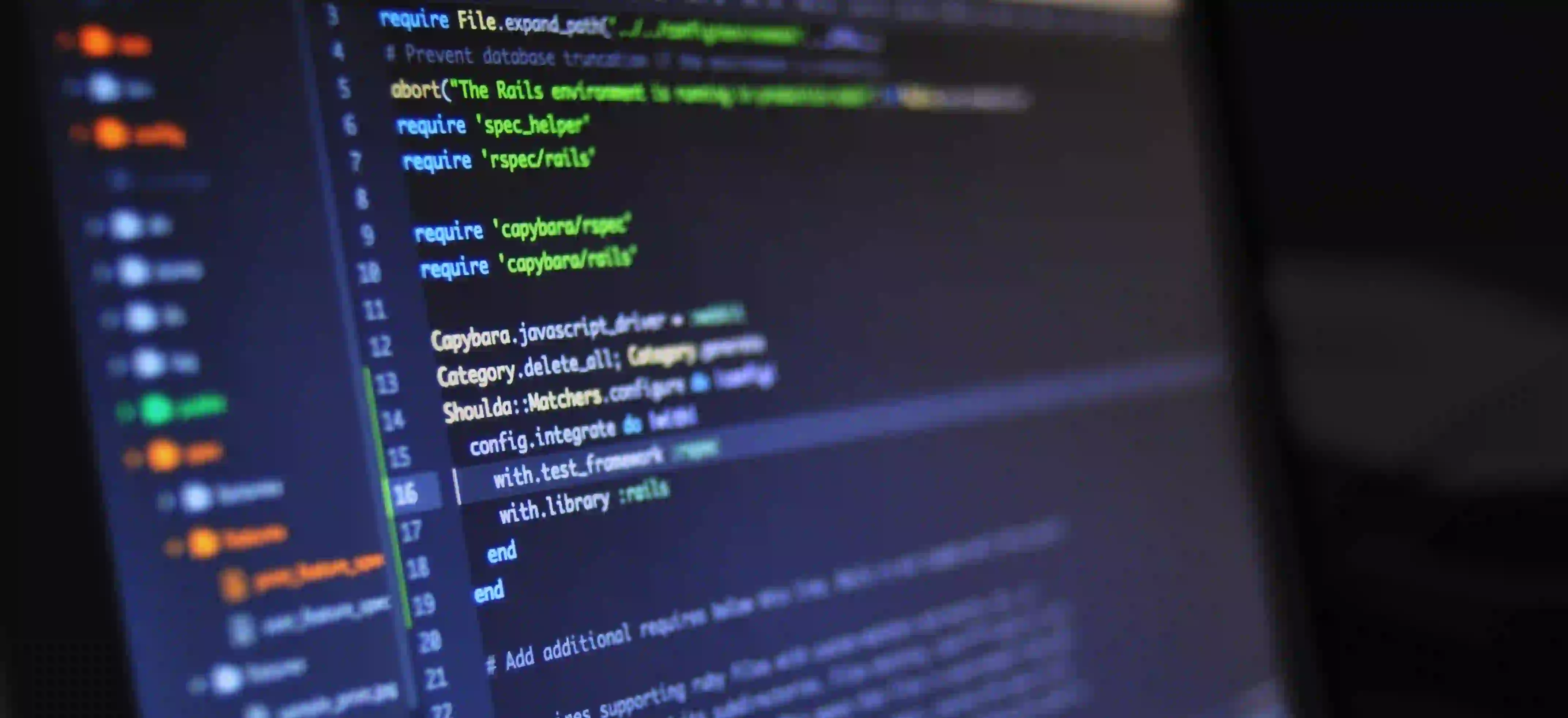
In Java programming, null values are a common source of bugs and errors. This is why Java 8 introduced the Optional class, which provides a more streamlined way to handle null values, reducing the likelihood of encountering NullPointerExceptions. In this post, we will delve into the nuances of handling null values effectively using Optional in Java 8, and demonstrate its practical applications with code examples.
Understanding the Problem
In traditional Java programming, dealing with null values often involves numerous null checks, making the code cluttered and difficult to read. Consider the following example:
String result = null;
if (obj != null && obj.getField() != null) {
result = obj.getField().getValue();
}
This code snippet demonstrates the rigorous checking required to avoid null pointer exceptions when accessing nested fields or methods of objects. With Optional, however, this can be streamlined and made more readable.
Introducing Optional
Optional is a container object that may or may not contain a non-null value. It is designed to provide a more expressive way to represent the absence of a value, thereby mitigating the need for explicit null checks. Let's revisit the previous example using Optional:
Optional<Object> optionalObj = Optional.ofNullable(obj);
String result = optionalObj.flatMap(o -> Optional.ofNullable(o.getField()))
.map(Field::getValue)
.orElse("default");
In this refactored code, the Optional instance is used to map and chain the field access and value retrieval operations. This concise representation enhances readability and eliminates the need for explicit null checks.
Benefits of Optional
Using Optional offers several advantages, including:
-
Preventing NullPointerExceptions: Optional encapsulates the possibility of a null value, making it easier to handle and less prone to causing null pointer exceptions.
-
Enhanced Readability: By chaining methods and using functional interfaces, Optional facilitates a more fluent and understandable style of coding.
-
Forcing Explicit Handling: When working with Optional, developers are encouraged to explicitly handle both the presence and absence of a value, leading to more robust and predictable code.
Now that we have established the advantages of Optional, let's explore some practical scenarios where Optional can be effectively utilized.
Applying Optional in Practice
1. Retrieving a Value
Consider a scenario where you need to retrieve a value from an object that may be null. With Optional, you can elegantly handle this situation:
Optional<String> optionalValue = Optional.ofNullable(obj.getValue());
String result = optionalValue.orElse("default");
In this example, the orElse method is used to provide a default value if the original value is null. This succinct approach is more expressive than traditional null checks.
2. Chaining Operations
Optional's flatMap and map methods allow for chaining operations on potentially null values. This is particularly useful when dealing with nested objects or method calls. Let's illustrate this with an example:
Optional<Result> optionalResult = Optional.ofNullable(obj)
.flatMap(Obj::getNestedObj)
.map(NestedObj::getResult);
This chaining permits streamlined access to deeply nested properties, reducing verbosity and enhancing code clarity.
3. Conditional Processing
Optional also supports conditional processing using the ifPresent method, which allows for executing a specific action only when a non-null value is present:
Optional.ofNullable(obj)
.ifPresent(o -> System.out.println("Value: " + o));
By leveraging Optional, you can handle null values in a more concise and declarative manner, improving code quality and maintainability.
Best Practices and Considerations
While Optional provides a powerful tool for handling null values, it is essential to apply it judiciously. Consider the following best practices when using Optional:
-
Limited Use: It is advisable to use Optional primarily for return types as opposed to method parameters or collections. Overusing Optional can lead to unnecessary complexity.
-
Avoid Nesting: Excessive nesting of Optional operations should be avoided to maintain code clarity. Instead, consider extracting complex logic into separate methods.
-
Performance Considerations: As with any abstraction, be mindful of performance implications when using Optional, especially in performance-critical code sections.
By adhering to these best practices, developers can maximize the benefits of Optional while minimizing potential drawbacks.
Key Takeaways
In Java 8, Optional provides a compelling solution for handling null values, offering a more concise and expressive approach compared to traditional null checks. By leveraging Optional, developers can enhance the robustness, readability, and maintainability of their code while mitigating the risk of null pointer exceptions.
In conclusion, Optional is a valuable addition to the Java language that empowers developers to write cleaner, more resilient code. Incorporating Optional into your coding practices can lead to more predictable and maintainable codebases, ultimately benefiting both developers and end users.