Project Jigsaw: Solving Java 9's Modularity Issue
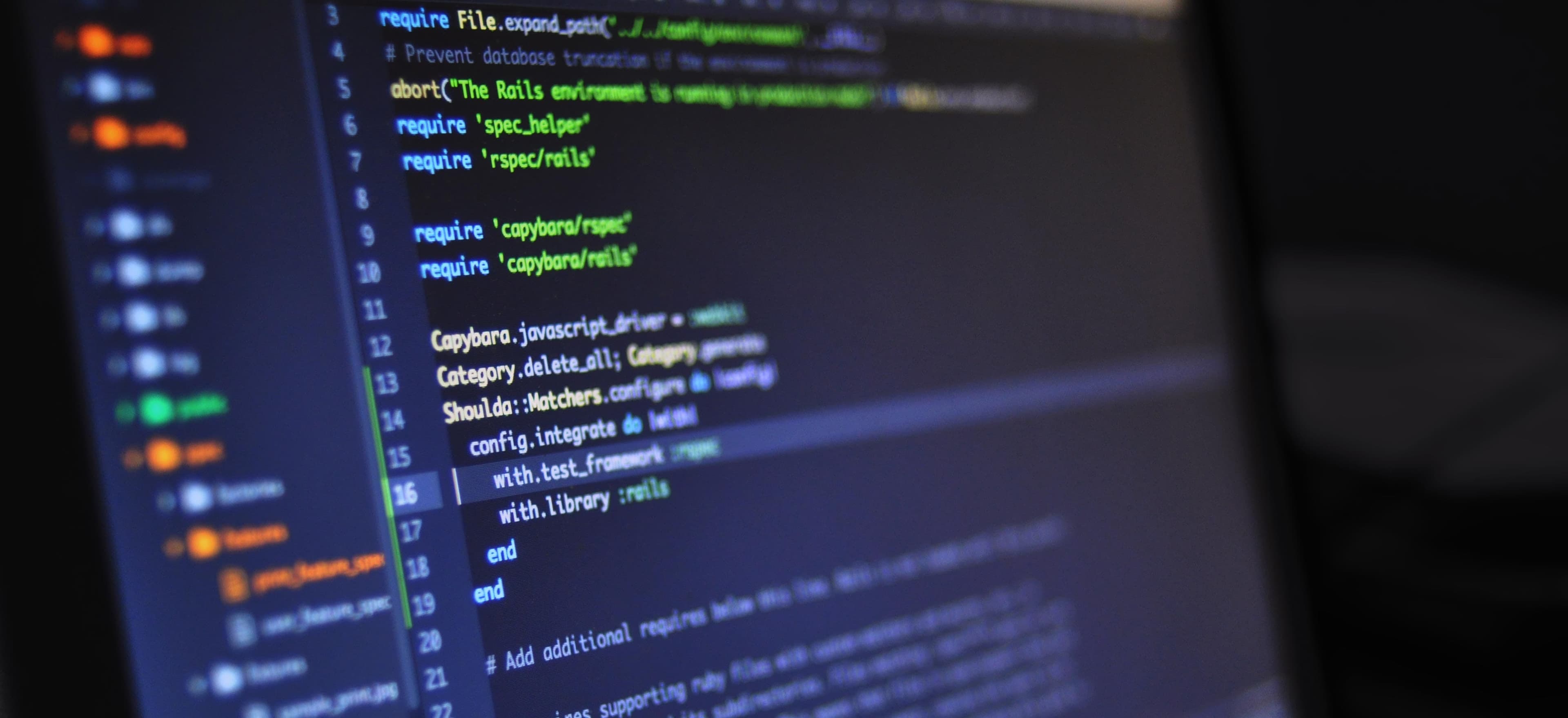
- Published on
Understanding Project Jigsaw in Java 9
Project Jigsaw is an initiative undertaken to modularize the Java SE platform and JDK. This comprehensive modularity framework was introduced in Java 9 to address the long-standing issue of modularity in the Java platform.
What is Modularity?
Modularity, in the context of software development, refers to the process of breaking down a system into smaller, independent modules. In Java, modularity allows developers to encapsulate code, manage dependencies, and improve the maintainability and reusability of their applications.
The Problem with Modularity in Java 8
Prior to Java 9, the Java platform lacked a built-in module system, leading to a monolithic and tightly-coupled structure. This made it challenging to create, manage, and maintain large-scale applications. Developers resorted to third-party tools and conventions, which often resulted in complexities and inconsistencies.
Introducing Project Jigsaw
Project Jigsaw was intended to bring a module system to Java that is scalable and maintainable. It aimed to address the following key objectives:
- Strong Encapsulation: The ability to hide internal details of a module and only expose specific parts to the external world.
- Reliable Configuration: Ensuring that the dependencies between modules are explicit and well-defined.
- Scalable Development: Supporting the creation and maintenance of large-scale applications through modularization.
Modules in Java 9
In Java 9, a new module-info.java
file was introduced to declare a module. This file resides in the root of the module directory and contains essential metadata about the module, including its dependencies.
Let's consider an example where we have a com.example
module depending on the com.util
module. The module-info.java
file for com.example
would look like this:
module com.example {
requires com.util;
}
In this code snippet, the requires
keyword specifies that the com.example
module depends on the com.util
module. This dependency declaration ensures a clear and explicit relationship between modules.
Benefits of Modularity with Project Jigsaw
The implementation of Project Jigsaw in Java 9 brings several benefits to developers and enterprises:
- Better Organization: Modules allow developers to organize their codebase into cohesive units, making it easier to understand and maintain.
- Strong Encapsulation: Modules provide a clear boundary for encapsulation, preventing unauthorized access to internal APIs.
- Improved Security: With strong encapsulation, modules enhance the security of an application by controlling access to internal components.
- Simplified Dependency Management: Defined module dependencies streamline the management of dependencies, reducing classpath issues and runtime errors.
How Project Jigsaw Resolves Modularity Issues
Project Jigsaw resolves the modularity issues in Java 8 through the following mechanisms:
-
Module Path and JAR Files:
In Java 9, modules are stored in JAR files, and the module path replaces the classpath. This allows for modularization at both compile-time and runtime, enabling better dependency management.
-
Module Declarations:
The
module-info.java
file provides a standardized way to declare module names, dependencies, and exports. The explicit declaration of dependencies improves the reliability of an application's configuration. -
Strong Encapsulation:
With Project Jigsaw, strong encapsulation ensures that the internal APIs of a module are shielded from external access, reducing the risk of unintended dependencies and potential conflicts.
-
Command-line Enhancements:
Java 9 introduces new command-line options to work with modules, enabling developers to compile, package, and run modular applications effectively.
Code Migration to Modular Structure
Migrating existing code to leverage the module system introduced by Project Jigsaw involves the following steps:
-
Identify Modules:
Analyze the codebase to identify cohesive and independent components that can be encapsulated as modules.
-
Create
module-info.java
:Introduce the
module-info.java
file in each module to declare its name, dependencies, and exported packages. -
Update Build Process:
Modify build scripts and processes to account for the new module structure and dependencies.
-
Resolve Reflection Issues:
In cases where reflection is used to access inaccessible members, update the code to comply with the strong encapsulation enforced by modules.
Leveraging Java 9 Modularity in Development
To showcase the benefits of Java 9 modularity, let's consider an example where we refactor a simple Java application into modules.
Before Modularization:
// com.example.MyApplication in a monolithic structure
package com.example;
import com.util.Utility;
public class MyApplication {
public static void main(String[] args) {
Utility.doSomething();
}
}
After Modularization:
// com.example module-info.java
module com.example {
requires com.util;
}
// com.example.MyApplication
package com.example;
import com.util.Utility;
public class MyApplication {
public static void main(String[] args) {
Utility.doSomething();
}
}
In this example, we have modularized the com.example
module, explicitly declaring its dependency on the com.util
module. This approach isolates the dependencies and enforces a clear module boundary, leading to better maintainability and reusability.
Final Considerations
Java 9's Project Jigsaw addresses the longstanding modularity issues in the Java platform, introducing a robust module system to enhance code organization, encapsulation, and dependency management. With the module-info.java
file and strong encapsulation, developers can leverage modularity to build scalable, maintainable, and secure applications.
Embracing modularity with Project Jigsaw paves the way for a more efficient Java development ecosystem, fostering better software architecture and improved code quality.
Java 9's modularity, powered by Project Jigsaw, stands as a pivotal advancement in Java development, offering a structured approach to building and maintaining robust and scalable software solutions.
For further reading on Java 9 and Project Jigsaw, refer to the official Oracle documentation.