Choosing the Right Service Discovery Tool
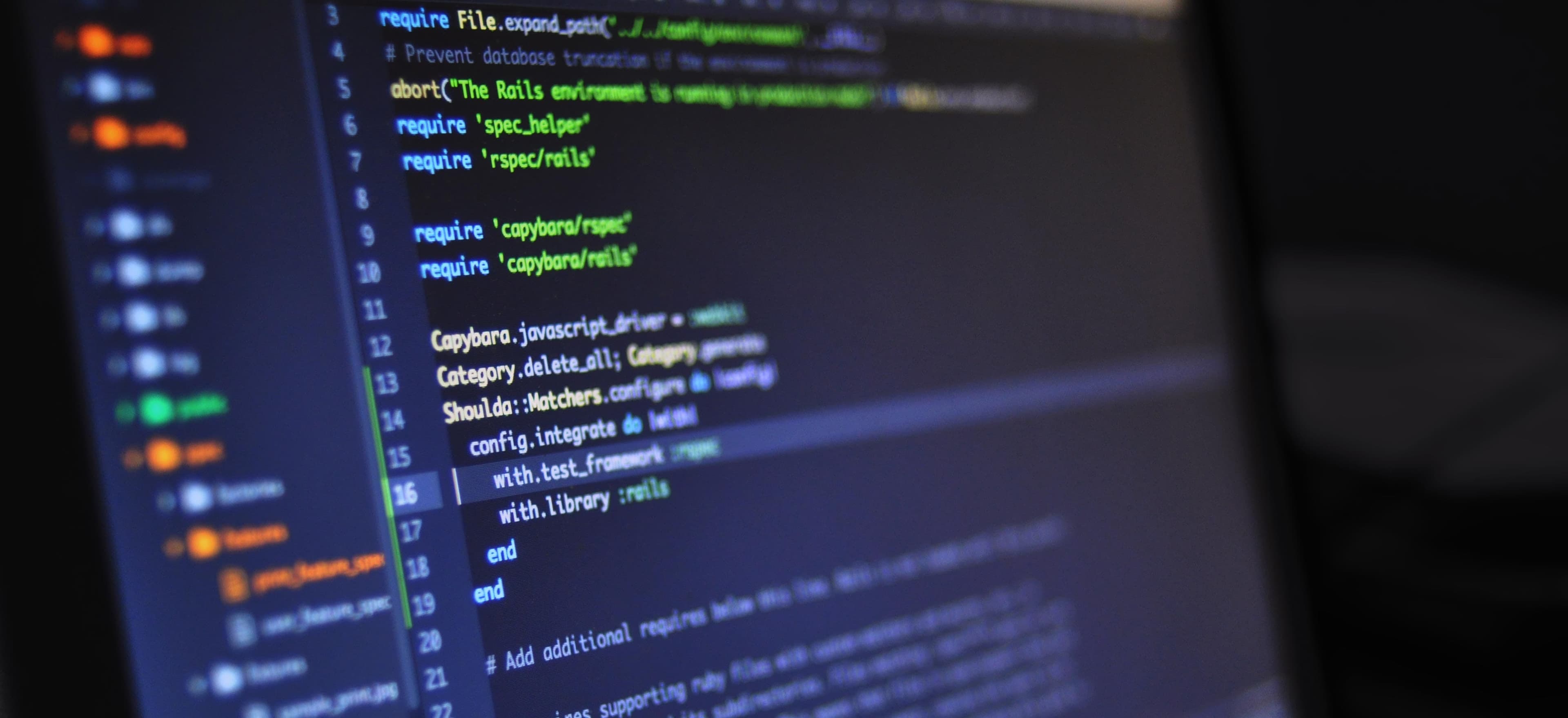
- Published on
Service discovery is a critical aspect of building modern, scalable, and resilient applications. In a microservices architecture, where services come and go dynamically, service discovery plays a crucial role in enabling seamless communication between services.
Java, as a popular programming language for building enterprise applications, offers a variety of tools and libraries for implementing service discovery. In this article, we'll explore the concept of service discovery and evaluate some of the best service discovery tools in the Java ecosystem.
What is Service Discovery?
Service discovery is the process of automatically detecting and locating services in a dynamic and cloud-native environment. When services are deployed in a distributed system, they need to find each other to establish communication. Traditional methods of configuring static IP addresses or using configuration files become impractical in dynamic environments where services can be added, removed, or scaled up and down automatically.
Service discovery solves this problem by providing a dynamic registry where services can register themselves and discover the locations of other services. This allows services to locate and communicate with each other without any manual intervention, making the system more agile and adaptable to changes.
Criteria for Choosing a Service Discovery Tool
Before we dive into specific service discovery tools, it's essential to understand the criteria for evaluating and choosing the right tool for your Java application.
1. Integration with Java Ecosystem
The service discovery tool should seamlessly integrate with Java-based microservices frameworks and libraries. It should provide client libraries or SDKs that are easy to use within Java applications.
2. Scalability and Performance
The tool should be able to handle a large number of services and requests without introducing significant latency or performance overhead. It should scale horizontally to accommodate the dynamic nature of cloud-native environments.
3. Resilience
The service discovery tool should be resilient to failures and network partitions. It should have built-in mechanisms for handling outages and recovering from temporary disruptions.
4. Consistency and Consensus
Consistency and consensus algorithms are crucial for ensuring that the service registry remains accurate and up to date, even in the presence of network partitions or failures.
5. Monitoring and Metrics
The tool should provide visibility into the health and performance of the service registry. Monitoring capabilities and metrics collection are essential for operational visibility and troubleshooting.
Best Service Discovery Tools for Java
Now that we understand the criteria, let's explore some of the best service discovery tools in the Java ecosystem.
1. Eureka
Netflix's Eureka is a widely-used service registry and discovery tool, especially in conjunction with Spring Cloud for building Java-based microservices. Eureka uses a client-side discovery approach, where each service instance registers itself with the Eureka server. Other services can then query the Eureka server to discover available service instances.
// Example of Eureka client registration using Spring Cloud
@EnableDiscoveryClient
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
Why Eureka?
- Eureka integrates seamlessly with the Spring Cloud ecosystem, making it an excellent choice for Java microservices built with Spring Boot.
- Client-side discovery reduces the load on the service registry and allows for more flexible routing and load balancing strategies.
2. Consul
Consul, from HashiCorp, is a comprehensive tool for service discovery, service mesh, and key-value store. It provides a dynamic service catalog where services can register, deregister, and discover each other. Consul also supports health checks and distributed key-value storage, making it a versatile choice for building resilient applications.
// Example of Consul client registration using Java client library
Consul client = Consul.builder().build();
AgentClient agentClient = client.agentClient();
agentClient.register(8080, 3, "service-1", "tag-1");
Why Consul?
- Consul's built-in support for service mesh capabilities like Connect and intentions makes it a compelling choice for advanced networking and security requirements.
- It provides a rich HTTP API and client libraries for integrating with Java applications.
3. Zookeeper
Apache ZooKeeper, while not exclusively a service discovery tool, is commonly used as a distributed coordination and consensus service. It can be leveraged for service discovery by using ephemeral znodes to represent service instances. ZooKeeper's strong consistency guarantees and battle-tested reliability make it suitable for scenarios where strong coordination and consensus are critical.
// Example of ZooKeeper service registration using Curator framework
CuratorFramework client = CuratorFrameworkFactory.newClient("zookeeper1:2181", new RetryNTimes(3, 1000));
client.start();
client.create().withMode(CreateMode.EPHEMERAL).forPath("/services/service-1", "data".getBytes());
Why Zookeeper?
- ZooKeeper's proven reliability and strong consistency make it a solid choice for critical infrastructure and coordination requirements.
- It provides a rich set of recipes and abstractions through the Curator framework, simplifying the integration with Java applications.
4. Kubernetes
For Java applications running in Kubernetes clusters, leveraging Kubernetes' built-in service discovery and load balancing features is a natural choice. Kubernetes Services abstract away the details of the underlying network, providing a stable endpoint for accessing a group of pods. Java applications can simply use the service name to discover and communicate with other services within the same Kubernetes cluster.
// Example of Kubernetes service discovery using environment variables
String serviceName = System.getenv("SERVICE_NAME");
String serviceHost = System.getenv(serviceName + "_SERVICE_HOST");
String servicePort = System.getenv(serviceName + "_SERVICE_PORT");
Why Kubernetes?
- For Java applications running in Kubernetes, leveraging the built-in service discovery and load balancing capabilities simplifies the overall architecture and reduces the need for additional service discovery layers.
- Kubernetes' service abstraction provides a stable and self-configuring way for Java services to discover and communicate with each other.
My Closing Thoughts on the Matter
In conclusion, service discovery is a critical component of modern microservices architectures, and choosing the right service discovery tool is crucial for building scalable, resilient, and efficient Java applications. Each of the discussed tools - Eureka, Consul, ZooKeeper, and Kubernetes - brings its own strengths and considerations, so the choice ultimately depends on the specific requirements and constraints of your application environment.
When selecting a service discovery tool, it's essential to consider factors such as integration with the Java ecosystem, scalability, resilience, consistency, and monitoring capabilities. By carefully evaluating these criteria and understanding the strengths of each tool, you can make an informed decision that best suits your Java microservices architecture.
In the rapidly evolving landscape of microservices and cloud-native technologies, the right service discovery tool can make a significant difference in the agility, reliability, and performance of your Java applications. Choose wisely, and build with confidence.
Remember, the best tool is the one that seamlessly aligns with your application's needs and environment. Choose wisely and build robust, scalable services with the power of Java!