Maximizing Value: Navigating the Valhalla Development Project
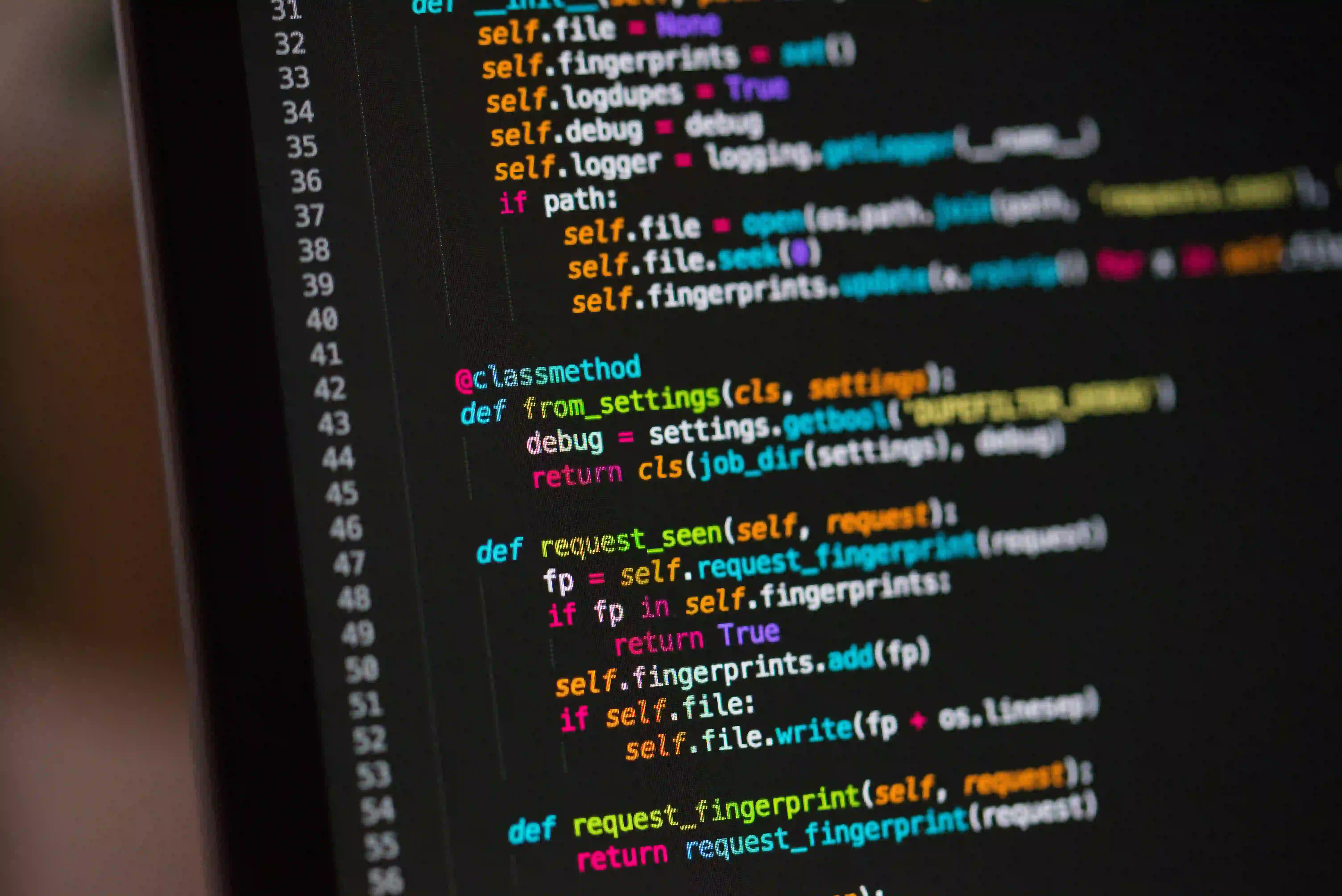
Maximizing Value: Navigating the Valhalla Development Project
The Valhalla Development Project is an ambitious endeavor requiring a solid and efficient codebase. In this post, we will delve into Java's capabilities and explore how to utilize them to maximize value in this project. We will cover essential topics such as code modularity, performance optimization, and best practices to ensure seamless progress.
Modularization for Efficiency
Modularity is crucial for maintaining a scalable and maintainable codebase. By breaking down the project into cohesive modules, we ensure that each component has well-defined responsibilities and interfaces. Java's modular system, introduced in Java 9, allows us to achieve this seamlessly.
Example of Module Declaration in Java
module com.valhalla.core {
requires transitive com.valhalla.util;
exports com.valhalla.core.models;
// other module declarations
}
In this example, the com.valhalla.core
module declares its dependencies and exports the necessary packages. This promotes encapsulation and loose coupling, facilitating easier integration of different modules.
The 'Why' Behind Modularity
Modularity enhances code maintainability, promotes reusability, and facilitates parallel development. It also enables better manageability of dependencies and provides a clear structure, making the codebase more comprehensible for new developers joining the project.
Performance Optimization with Concurrency
In a project as extensive as Valhalla, optimizing performance is paramount. Java provides robust support for concurrent programming through its java.util.concurrent
package. Leveraging multithreading and concurrent data structures can significantly boost the project's responsiveness and throughput.
Utilizing Java's Executor Framework
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < n; i++) {
executor.submit(() -> processTask(i));
}
executor.shutdown();
In this snippet, we create a fixed thread pool to execute tasks concurrently. This is a concise yet powerful approach to improve performance.
The 'Why' Behind Concurrency
Concurrency allows efficient utilization of system resources, leads to improved responsiveness, and is essential for handling multiple tasks simultaneously. By harnessing the power of concurrency, we can maximize the project's performance while ensuring efficient resource management.
Embracing Best Practices for Maintainability
Maintainability is a cornerstone of any successful software project. Adhering to best practices not only enhances the code's readability but also simplifies debugging and refactoring processes.
Enforcing Code Style with Checkstyle
Integrating Checkstyle into the project's build process enables us to enforce a consistent code style across the codebase. This helps in maintaining a uniform and high-quality code structure.
The 'Why' Behind Best Practices
By embracing best practices, we reduce technical debt, enhance code comprehensibility, and streamline the onboarding process for new team members. Consistent adherence to best practices promotes smoother collaboration and facilitates long-term maintainability.
Leveraging Java's Ecosystem for Efficacy
Java's ecosystem offers a plethora of libraries and frameworks to expedite development and enhance the project's capabilities. Leveraging these tools can significantly accelerate the project's progress and bolster its functionality.
Integration of JUnit for Robust Testing
@Test
public void calculateTotalCost() {
// Test logic for calculating total cost
// Assert expected result
}
By incorporating JUnit for automated testing, we can ensure the reliability and correctness of the project's codebase. This fosters a robust and resilient system.
The 'Why' Behind Ecosystem Integration
Utilizing established libraries and frameworks accelerates development, reduces development effort, and enhances the project's reliability. By leveraging Java's vibrant ecosystem, we can tap into a wealth of resources to fortify the Valhalla project.
In conclusion, through strategic modularity, performance optimization, adherence to best practices, and effective utilization of Java's ecosystem, the Valhalla Development Project can maximize its value and potential. By embracing these principles and harnessing Java's capabilities, we pave the way for a robust, scalable, and efficient codebase.
To delve deeper into modularity in Java, you can refer to the official documentation on Java Platform, Standard Edition & Java Development Kit.
Similarly, for a comprehensive understanding of performance optimization through concurrency in Java, exploring the resources provided by the Java Concurrency in Practice book can be invaluable.