Optimizing Stock Control for Real-Time Inventory Management
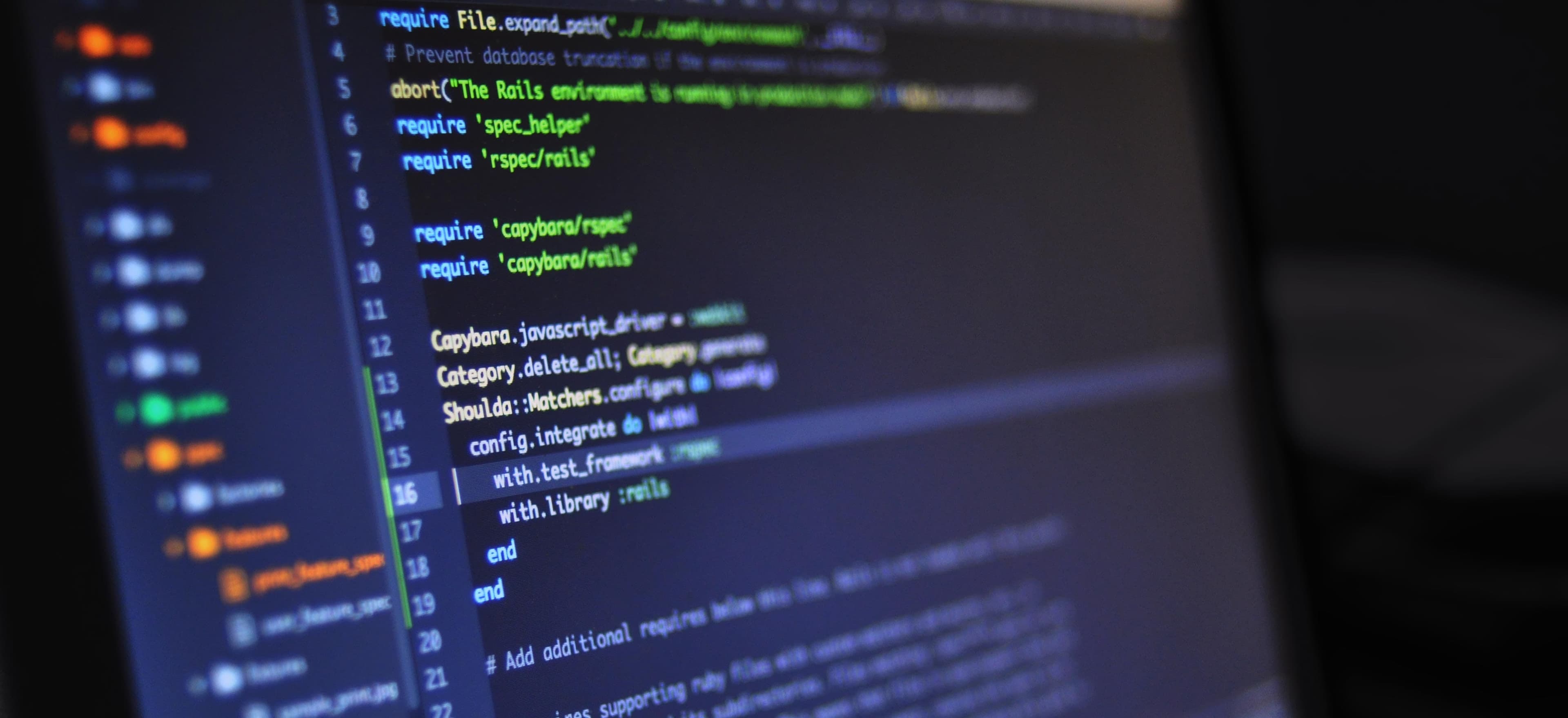
- Published on
Optimizing Stock Control for Real-Time Inventory Management
In today's fast-paced business environment, real-time inventory management is crucial for ensuring the smooth operation of supply chains. For businesses that deal with a large volume of stock, having an efficient stock control system in place can make all the difference in meeting customer demand and maintaining profitability.
Java, with its powerful and versatile features, offers a robust platform for developing real-time inventory management systems. In this blog post, we will explore how to optimize stock control for real-time inventory management using Java, and how it can help businesses streamline their operations and stay competitive in the market.
Understanding the Importance of Real-Time Inventory Management
Before delving into the technical aspects of optimizing stock control, it's important to understand the significance of real-time inventory management. Traditional inventory management systems often rely on periodic manual checks or batch updates, which can lead to inaccuracies and inefficiencies.
Real-time inventory management, on the other hand, provides up-to-the-minute visibility into stock levels, enabling businesses to make informed decisions, prevent stockouts, and minimize excess inventory. This not only enhances operational efficiency but also improves customer satisfaction by ensuring product availability.
Leveraging Java for Real-Time Inventory Management
Java's popularity and widespread use in enterprise applications make it an ideal choice for developing real-time inventory management systems. Java's strong support for multithreading, networking, and database connectivity allows developers to create scalable, responsive, and reliable inventory control solutions.
By leveraging Java's robust libraries, such as java.util.concurrent for concurrent data structures and java.sql for database interactions, developers can build a solid foundation for real-time inventory management systems.
Let's take a closer look at some key strategies for optimizing stock control in real-time inventory management using Java.
Using Concurrent Data Structures for Thread-Safe Operations
In a real-time inventory management system, concurrent access to stock data is inevitable, especially in a multi-user environment. To ensure data consistency and integrity, it's essential to use thread-safe data structures when manipulating stock information.
Java provides a rich set of concurrent data structures, such as ConcurrentHashMap and CopyOnWriteArrayList, which are designed for concurrent access without the need for external synchronization. By using these structures, developers can perform operations on stock data concurrently with minimal risk of data corruption or inconsistency.
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class Inventory {
private Map<String, Integer> stock = new ConcurrentHashMap<>();
public void updateStock(String productCode, int quantity) {
stock.put(productCode, quantity);
}
public int getStock(String productCode) {
return stock.getOrDefault(productCode, 0);
}
}
In this example, we use a ConcurrentHashMap to store stock information, ensuring that the updateStock and getStock methods can be safely accessed by multiple threads without the need for explicit synchronization.
Implementing Event-Driven Architecture for Real-Time Updates
Real-time inventory management relies on timely updates to stock levels based on various events, such as sales transactions, stock replenishments, and supplier deliveries. Implementing an event-driven architecture allows the system to react to these events in real time and update stock levels accordingly.
Java provides comprehensive support for event handling through frameworks like Spring Framework and Apache Kafka. By leveraging these frameworks, developers can design a reactive inventory management system that responds to stock-related events with minimal latency.
public interface StockUpdateListener {
void onStockUpdated(String productCode, int newStockLevel);
}
public class InventoryManager {
private List<StockUpdateListener> listeners = new ArrayList<>();
public void addStockUpdateListener(StockUpdateListener listener) {
listeners.add(listener);
}
public void updateStock(String productCode, int quantity) {
// Perform stock update
notifyListeners(productCode, newStockLevel);
}
private void notifyListeners(String productCode, int newStockLevel) {
for (StockUpdateListener listener : listeners) {
listener.onStockUpdated(productCode, newStockLevel);
}
}
}
In this example, the InventoryManager class notifies registered listeners of stock updates, allowing other parts of the system to react to real-time stock changes, such as updating UI displays or triggering automated stock replenishments.
Leveraging Database Transactions for Atomic Stock Operations
When managing stock control, it's crucial to ensure atomicity and consistency in stock operations to avoid data anomalies. Java's support for database transactions through JDBC or JPA enables developers to enforce atomic stock operations, such as stock updates and reservations, within a transactional boundary.
By encapsulating stock-related operations within database transactions, developers can ensure that stock updates either succeed entirely or fail entirely, preventing partial updates or inconsistencies in stock data.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class StockService {
private static final String DB_URL = "jdbc:mysql://localhost:3306/inventory";
private static final String DB_USER = "username";
private static final String DB_PASSWORD = "password";
public void updateStock(String productCode, int quantity) {
String updateQuery = "UPDATE products SET stock = stock + ? WHERE product_code = ?";
try (Connection connection = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
PreparedStatement statement = connection.prepareStatement(updateQuery)) {
connection.setAutoCommit(false);
statement.setInt(1, quantity);
statement.setString(2, productCode);
statement.executeUpdate();
connection.commit();
} catch (SQLException e) {
// Handle exception
}
}
}
In this example, the updateStock method performs a stock update within a database transaction, ensuring that the stock level is updated atomically without the risk of intermediate inconsistencies.
Conclusion
Real-time inventory management is a critical aspect of modern supply chain operations, and optimizing stock control is essential for ensuring accurate and up-to-date stock information. By leveraging the powerful features of Java, such as concurrent data structures, event-driven architecture, and database transactions, developers can build robust and responsive inventory management systems that meet the demands of today's dynamic business environment.
Incorporating these strategies into real-time inventory management systems not only improves operational efficiency but also enhances customer satisfaction by ensuring product availability and timely fulfillment.
In the ever-evolving landscape of inventory management, Java continues to be a reliable and versatile technology for building scalable and performant solutions that drive business success.
Optimizing stock control for real-time inventory management is not just a technical endeavor but a strategic investment in ensuring the competitiveness and resilience of businesses in an increasingly competitive market.
Start optimizing your stock control with Java today and stay ahead of the curve in real-time inventory management.
Contact us to learn more about how our Java expertise can help you optimize your inventory management.
Remember, keeping your inventory management system updated in real-time is crucial for your business' success.
Checkout our other articles