Integrating Weld with Embedded Jetty Server
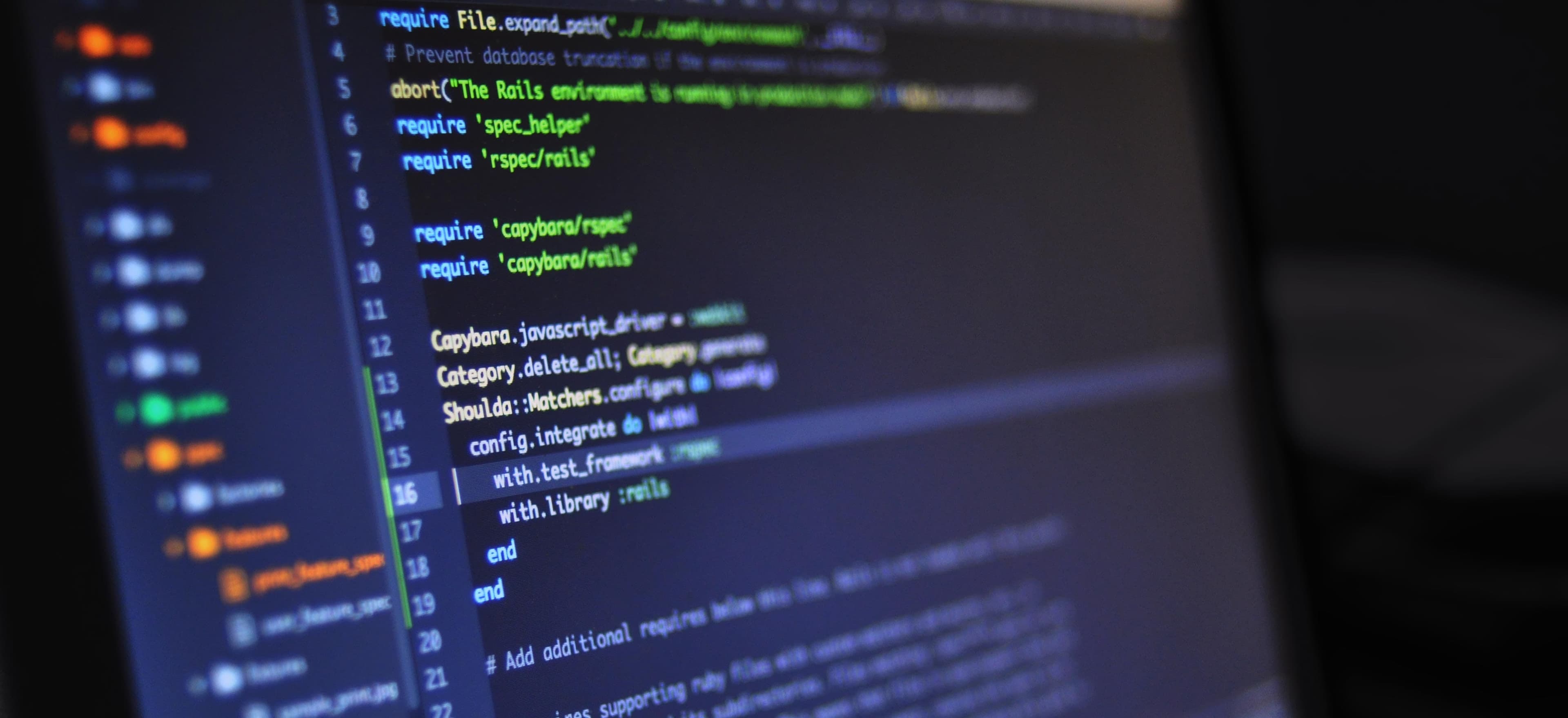
- Published on
Integrating Weld with Embedded Jetty Server
In this blog post, we'll discuss how to integrate Weld, the reference implementation of Contexts and Dependency Injection for Java (CDI), with an embedded Jetty server. This integration allows you to leverage the power of CDI in your web applications running on Jetty.
What is Weld?
Weld is an implementation of the CDI specification, which provides a powerful set of services for managing component lifecycle and dependencies in Java EE and Jakarta EE applications. It allows you to write decoupled and modular code by promoting the use of dependency injection, interception, and contextual lifecycle management.
What is Jetty?
Jetty is a lightweight, embeddable web server and servlet container that is often used in microservices and standalone web applications. It provides a simple and extensible API for creating web servers and handling HTTP requests.
Why Integrate Weld with Jetty?
By integrating Weld with Jetty, you can take advantage of CDI features such as dependency injection, scopes, and interceptors in your web applications. This allows for better modularization, testability, and maintainability of your code.
Now, let's dive into the steps to integrate Weld with an embedded Jetty server.
Step 1: Set Up a Maven Project
First, create a new Maven project or use an existing one. Ensure that the required dependencies for Weld and Jetty are included in the project's pom.xml
file. Here is an example of the necessary dependencies:
<dependencies>
<!-- Weld dependencies -->
<dependency>
<groupId>org.jboss.weld.se</groupId>
<artifactId>weld-se</artifactId>
<version>4.0.1.Final</version>
</dependency>
<!-- Jetty dependencies -->
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-server</artifactId>
<version>9.4.43.v20210629</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-servlet</artifactId>
<version>9.4.43.v20210629</version>
</dependency>
</dependencies>
Step 2: Configure Weld
Next, we need to initialize Weld and bootstrap the CDI container. We'll create a class to manually start Weld and obtain a reference to the CDI container:
import org.jboss.weld.environment.se.Weld;
import org.jboss.weld.environment.se.WeldContainer;
public class WeldContext {
private static Weld weld;
private static WeldContainer container;
public static void initialize() {
weld = new Weld();
container = weld.initialize();
}
public static void shutdown() {
if (container != null) {
container.shutdown();
}
if (weld != null) {
weld.shutdown();
}
}
public static WeldContainer getContainer() {
return container;
}
}
In this class, we use the Weld
and WeldContainer
classes provided by Weld to bootstrap the CDI environment. The initialize
method starts the Weld container, and the shutdown
method stops it when necessary. We also provide a method to retrieve the Weld container instance.
Step 3: Create a Servlet
Now, let's create a simple servlet that uses CDI to inject a bean and perform some operations. We'll use the @WebServlet
annotation provided by the Servlet API to define our servlet.
import jakarta.inject.Inject;
import jakarta.inject.Named;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
@WebServlet(urlPatterns = "/hello")
public class HelloServlet extends HttpServlet {
@Inject
@Named("greetingService")
private GreetingService greetingService;
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) {
String message = greetingService.greet("World");
// Send the 'message' as the HTTP response
}
}
In this servlet, we use the @Inject
annotation to inject the GreetingService
bean into the greetingService
field. The @Named
annotation is used to specify the qualifier for the injection, as there may be multiple implementations of the GreetingService
interface.
Step 4: Configure Jetty Server
We'll now create and configure the embedded Jetty server to use our servlet. We'll start by creating a main class to initialize the server and deploy our servlet:
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletContextHandler;
import org.eclipse.jetty.servlet.ServletHolder;
public class Main {
public static void main(String[] args) {
WeldContext.initialize(); // Initialize Weld
try {
Server server = new Server(8080);
ServletContextHandler context = new ServletContextHandler(ServletContextHandler.SESSIONS);
context.setContextPath("/");
server.setHandler(context);
context.addServlet(new ServletHolder(new HelloServlet()), "/hello");
server.start();
server.join();
} catch (Exception e) {
e.printStackTrace();
} finally {
WeldContext.shutdown(); // Shutdown Weld
}
}
}
In this main class, we initialize Weld using WeldContext.initialize()
, create an instance of the Jetty server, configure a servlet context, add our HelloServlet
to the context, start the server, and wait for it to finish processing requests.
Step 5: Run the Application
Finally, build the Maven project and run the Main
class. You can then access the HelloServlet
at http://localhost:8080/hello
and see the injected bean in action.
Wrapping Up
Integrating Weld with Jetty allows you to harness the power of CDI in your embedded web applications. By following the steps outlined in this blog post, you can leverage the benefits of dependency injection, scopes, and interceptors provided by CDI while using the lightweight and flexible Jetty server.
By combining these technologies, you can build modular, testable, and maintainable web applications with ease.
Give it a try and experience the seamless integration of Weld and Jetty in your next Java web project!
For further reading, you can refer to the official documentation of Weld and Jetty to explore more advanced features and best practices.
Happy coding!
Checkout our other articles