Troubleshooting Common Java Errors for Beginners
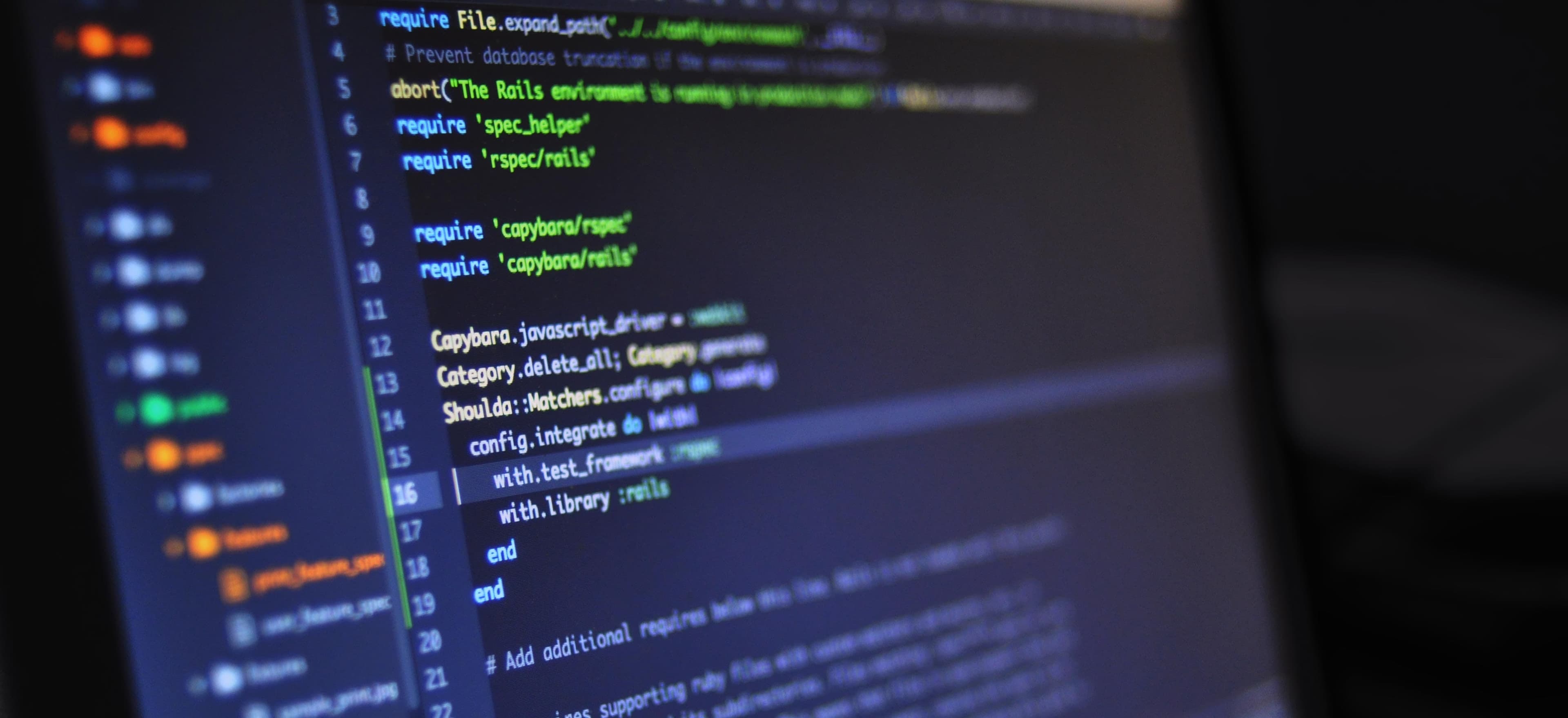
- Published on
Troubleshooting Common Java Errors for Beginners
Java is a robust and popular programming language, but like any language, it comes with its fair share of errors. As a beginner, encountering errors can be frustrating and daunting. However, understanding common Java errors and how to troubleshoot them is an essential skill for any Java developer. In this article, we will discuss some of the most frequent errors that beginners encounter when writing Java code and provide guidance on how to troubleshoot and resolve them.
1. Understanding the Error Messages
When you encounter an error in Java, the first step is to carefully read and understand the error message. Error messages in Java provide valuable information that can help you narrow down the issue and find a solution.
Let's consider an example - the "Cannot find symbol" error. This error occurs when the Java compiler cannot find a symbol referenced in your code, such as a variable or a method.
public class Example {
public static void main(String[] args) {
System.out.println(message);
}
}
In this example, if message
is not declared or instantiated, the compiler will throw a "Cannot find symbol" error. The error message will point you to the exact line where the issue occurred, helping you locate and fix the problem.
2. Common Errors and How to Fix Them
Error 1: Class, Interface, or Enum Expected
This error often occurs when there is a syntax issue, such as a missing semicolon or a misplaced brace.
Example:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!")
}
}
Solution:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Error 2: Null Pointer Exception
A NullPointerException
occurs when you try to access a member of an object that is null
.
Example:
String text = null;
int length = text.length();
Solution:
String text = null;
if (text != null) {
int length = text.length();
}
3. Utilizing Debugging Tools
Debugging is an essential skill for resolving errors in Java. Utilizing debugging tools such as breakpoints, watches, and stepping through the code can help you identify the root cause of the issue.
Breakpoints
Placing breakpoints in your code allows you to pause the program's execution at specific points and inspect the values of variables, helping you understand how the program flows and where issues may arise.
Watches
Watches enable you to monitor the value of specific variables as the program executes, providing insight into how values change and helping to pinpoint when and where errors occur.
Stepping
Stepping through the code, line by line, gives you a granular view of how the program is executing, making it easier to identify logic errors and unexpected behaviors.
4. Consulting Documentation and Online Resources
When you encounter a challenging error, don't hesitate to consult the official Java documentation and online resources such as Stack Overflow, Java forums, and tutorials. These sources often provide valuable insights and solutions to common and complex Java errors.
5. Leveraging IDE Features
Modern integrated development environments (IDEs) offer features that can significantly aid in troubleshooting Java errors. Code analysis, automated fixes, and real-time error highlighting are just a few examples of how IDEs can help streamline the error resolution process.
Automated Fixes
Many IDEs provide automated fixes for common errors, enabling you to quickly resolve issues based on suggestions from the IDE.
Real-time Error Highlighting
IDEs often highlight errors in real-time as you type, helping you catch and address issues as they occur, rather than after the entire program is written.
6. Seeking Peer Review and Collaboration
Seeking feedback from peers and more experienced developers can shed light on errors that may have been overlooked. Collaborating with others not only helps in resolving immediate errors but also fosters knowledge sharing and skill development.
7. Learning from Mistakes
As a beginner, it's essential to embrace errors as learning opportunities. Each error you encounter presents a chance to deepen your understanding of Java and become a more proficient developer.
Wrapping Up
Encountering errors is a natural part of the learning process when it comes to Java programming. By understanding common Java errors, leveraging debugging tools, utilizing IDE features, seeking guidance from documentation and peers, and embracing errors as learning opportunities, beginners can effectively troubleshoot and resolve Java errors. Remember, persistence and practice are key to mastering Java development. Happy coding!
In conclusion, becoming proficient in troubleshooting Java errors takes time and practice, but with the right approach and mindset, you can overcome challenges and become a more confident Java developer.