Choosing the Best Java Documentation Framework
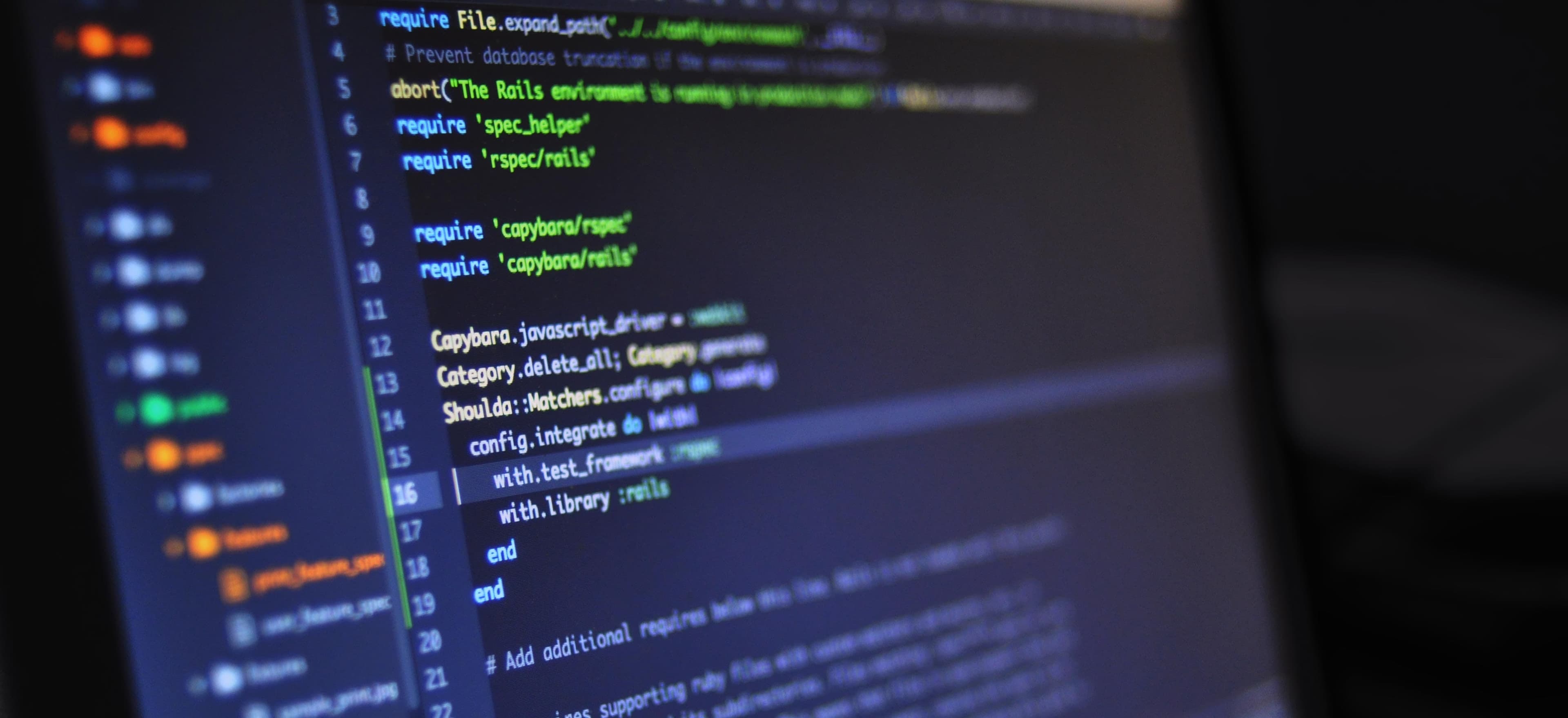
- Published on
When it comes to Java documentation, finding the best framework can significantly impact the software development process. Proper documentation ensures that code can be understood, maintained, and extended in the future. With numerous Java documentation frameworks available, it's crucial to select a framework that meets the project's specific needs. In this guide, we'll explore several Java documentation frameworks and discuss their features, use cases, and pros and cons to help you make an informed decision.
What is a Java Documentation Framework?
A Java documentation framework provides a structure for creating, organizing, and presenting documentation for Java projects. It allows developers to generate consistent and readable documentation that can be easily shared with others. The frameworks often integrate with the codebase, allowing documentation to be automatically generated from inline comments and annotations within the source code.
Popular Java Documentation Frameworks
1. Javadoc
Javadoc is a standard documentation generation tool that comes with the Java Development Kit (JDK). It uses special tags, such as @param
, @return
, and @throws
, to document classes, methods, and fields. Javadoc generates HTML-based documentation from these tags, making it easy to navigate and understand codebases.
Benefits
- Javadoc is widely adopted and integrated into many Java development environments.
- It follows a simple and familiar syntax, making it easy for developers to write and understand.
Drawbacks
- Limited customizability and styling options.
- Lack of support for multiple output formats beyond HTML.
An example of Javadoc usage:
/**
* Calculates the sum of two numbers.
*
* @param a the first number
* @param b the second number
* @return the sum of a and b
*/
public int calculateSum(int a, int b) {
return a + b;
}
In this example, the @param
and @return
tags provide descriptive information about the method's parameters and return value.
2. Javadoc Markdown
Javadoc Markdown is an extension to Javadoc that allows developers to write documentation in Markdown format within Javadoc comments. This approach combines the simplicity of Markdown with the structured nature of Javadoc, providing a more human-readable and aesthetically pleasing documentation output.
Benefits
- Markdown syntax is widely used and easily accessible to developers.
- Allows for more expressive and visually appealing documentation.
Drawbacks
- Limited support for advanced formatting and layout customization.
- Requires additional tooling for rendering Markdown within Javadoc comments.
An example of Javadoc Markdown:
/**
* # Calculator
* A simple calculator class to perform basic arithmetic operations.
*
* ## calculateSum
* Method to calculate the sum of two numbers.
*
* - `a` : the first number
* - `b` : the second number
*
* Returns the sum of `a` and `b`
*
* ```java
* public int calculateSum(int a, int b) {
* return a + b;
* }
* ```
*/
public class Calculator {
// class implementation
}
3. Doclet APIs (Custom Documentation Generation)
Doclet APIs allow developers to create custom documentation generators by leveraging the Javadoc tool and its APIs. This approach is suitable for projects requiring highly specialized or non-standard documentation formats. Developers can define their own tags, processing rules, and output formats to tailor the documentation generation process to their specific needs.
Benefits
- Offers unparalleled flexibility and extensibility for documentation generation.
- Enables the creation of custom documentation formats and outputs.
Drawbacks
- Requires significant effort to design, implement, and maintain custom documentation generators.
- Steeper learning curve compared to using standard Javadoc or Javadoc Markdown.
An example of a custom Doclet:
// Custom Doclet implementation
Choosing the Right Framework
When choosing a Java documentation framework, consider the following factors:
1. Project Requirements
Evaluate the specific documentation needs of your project. Consider the required output formats, styling preferences, and integration with existing tools and workflows.
2. Developer Familiarity
Assess the familiarity of the development team with the chosen documentation framework. Opt for a framework that developers can easily adopt and use effectively.
3. Customization Needs
Determine if the project requires extensive customization and flexibility in documentation generation. Some frameworks offer more customization options than others, catering to varied project requirements.
4. Tooling and Integration
Consider whether the chosen framework seamlessly integrates with relevant development tools and build processes. Compatibility with the existing toolchain can streamline documentation generation and maintenance.
Final Considerations
Selecting the best Java documentation framework involves analyzing the project's specific needs, considering developer familiarity, and assessing customization and integration requirements. While Javadoc serves as a standard and versatile choice, Javadoc Markdown offers a more expressive and visually appealing approach to documentation. For projects with specialized documentation needs, custom Doclet APIs provide unparalleled flexibility but require additional effort to implement and maintain.
Ultimately, the choice of a Java documentation framework should align with the project's requirements, the team's expertise, and the desired level of customization and integration. By carefully evaluating these factors, you can confidently choose the best documentation framework to ensure clear, comprehensive, and maintainable documentation for your Java projects.