Avoiding Declarative SQL Mode Pitfalls
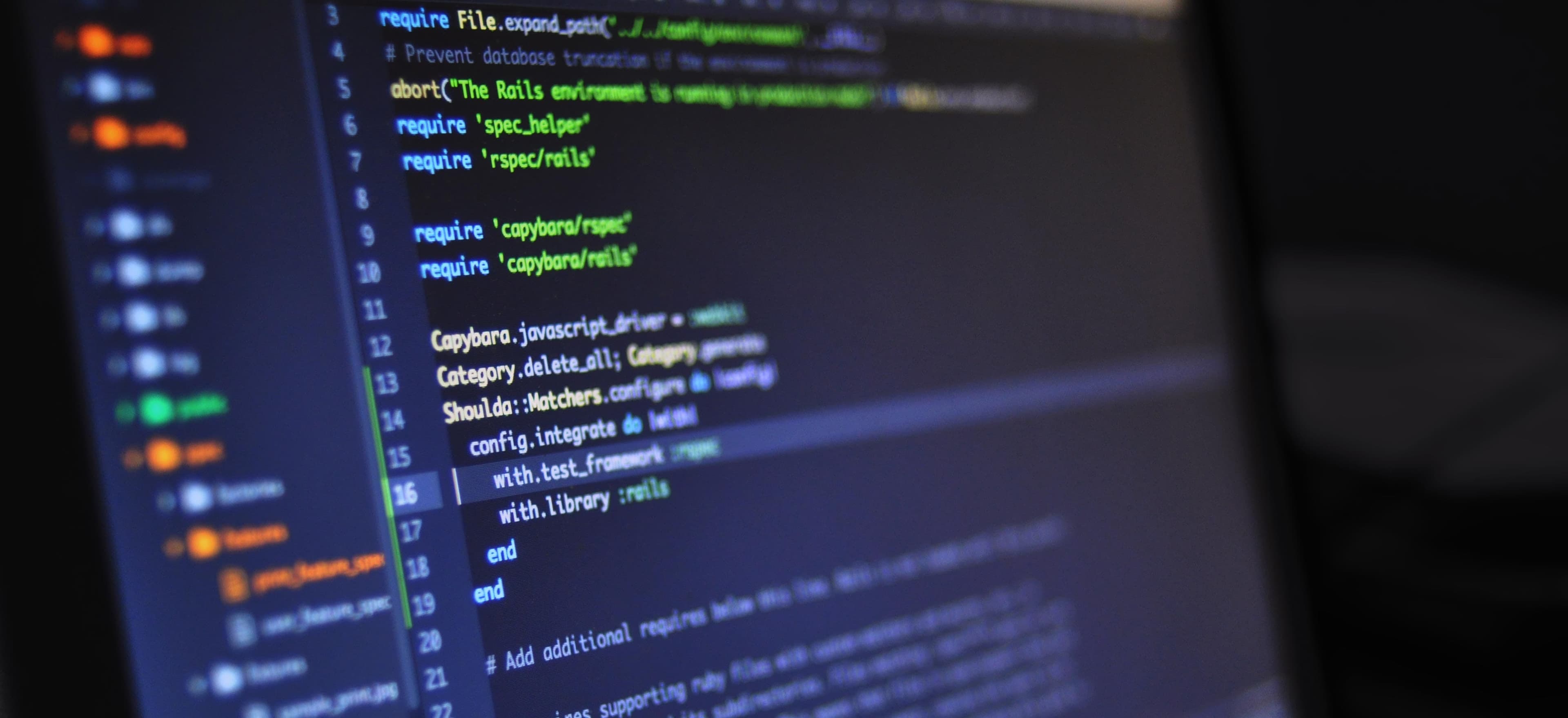
- Published on
Avoiding Declarative SQL Mode Pitfalls
Declarative SQL is one of the most common ways to interact with a database, and it provides a high level of abstraction for working with data. However, it also comes with its own set of pitfalls that can lead to performance issues, security vulnerabilities, and suboptimal usage of database resources. In this article, we'll explore some of the common pitfalls of using declarative SQL and discuss strategies for avoiding them.
Pitfall 1: Overusing SELECT *
Using SELECT *
to retrieve all columns from a table is a common shortcut, but it can have serious performance implications, especially on large tables. When you use SELECT *
, you're retrieving all columns, including potentially unnecessary data, which can lead to increased network traffic and slower query execution.
Solution
Instead of using SELECT *
, explicitly list the columns you need in the query. This not only makes the query more efficient by fetching only the necessary data but also provides better readability and maintainability.
// Bad practice
String query = "SELECT * FROM users";
// Good practice
String query = "SELECT id, name, email FROM users";
Pitfall 2: Not Utilizing Indexes
Indexes are essential for efficiently querying large datasets. Without proper indexes, queries may result in full table scans, which can be resource-intensive and slow.
Solution
Identify the columns that are frequently used in WHERE
, JOIN
, and ORDER BY
clauses and create indexes on those columns. This can significantly improve the performance of your queries by allowing the database to quickly locate the necessary rows.
// Creating an index in SQL
CREATE INDEX idx_name ON users(name);
Pitfall 3: Using LIKE
for Exact Matches
Using LIKE
for exact matches can create unnecessary overhead as it's typically used for pattern matching. When you're looking for an exact match, =
is more efficient.
Solution
Prefer using =
for exact matches instead of LIKE
to improve query performance.
// Bad practice
String query = "SELECT * FROM products WHERE name LIKE 'apple'";
// Good practice
String query = "SELECT * FROM products WHERE name = 'apple'";
Pitfall 4: Not Handling NULL Values Properly
NULL values can lead to unexpected behavior if not handled carefully. Comparisons involving NULL values can yield unexpected results if not explicitly accounted for.
Solution
When comparing columns that can contain NULL values, use IS NULL
or IS NOT NULL
to explicitly check for nullity.
// Bad practice
String query = "SELECT * FROM employees WHERE department_id = 1234";
// Good practice
String query = "SELECT * FROM employees WHERE department_id = 1234 OR department_id IS NULL";
Pitfall 5: Ignoring SQL Injection Attacks
Failure to sanitize user inputs in SQL queries can lead to SQL injection attacks, which can result in unauthorized access to your database and compromise the integrity of your data.
Solution
Use parameterized queries or prepared statements to avoid SQL injection attacks. Parameterized queries separate the SQL code from the user inputs, preventing malicious manipulation of the SQL code.
// Bad practice - Vulnerable to SQL injection
String query = "SELECT * FROM users WHERE username = '" + userInput + "'";
// Good practice - Using parameterized query
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users WHERE username = ?");
statement.setString(1, userInput);
ResultSet result = statement.executeQuery();
The Closing Argument
Declarative SQL is a powerful tool for working with databases, but it's important to be mindful of the potential pitfalls that come with it. By understanding these pitfalls and applying best practices, you can write more efficient, secure, and maintainable SQL code.
Remember to always consider the performance implications of your queries, utilize indexes effectively, handle NULL values appropriately, and guard against SQL injection attacks. These practices will not only improve the efficiency of your database operations but also enhance the security and stability of your applications.
By avoiding these common pitfalls, you'll be well on your way to mastering declarative SQL and leveraging it to its full potential in your applications.
For further reading, you can delve deeper into these topics:
Happy coding!