Unlocking Java Puzzles: Common Pitfalls for OCA Exam
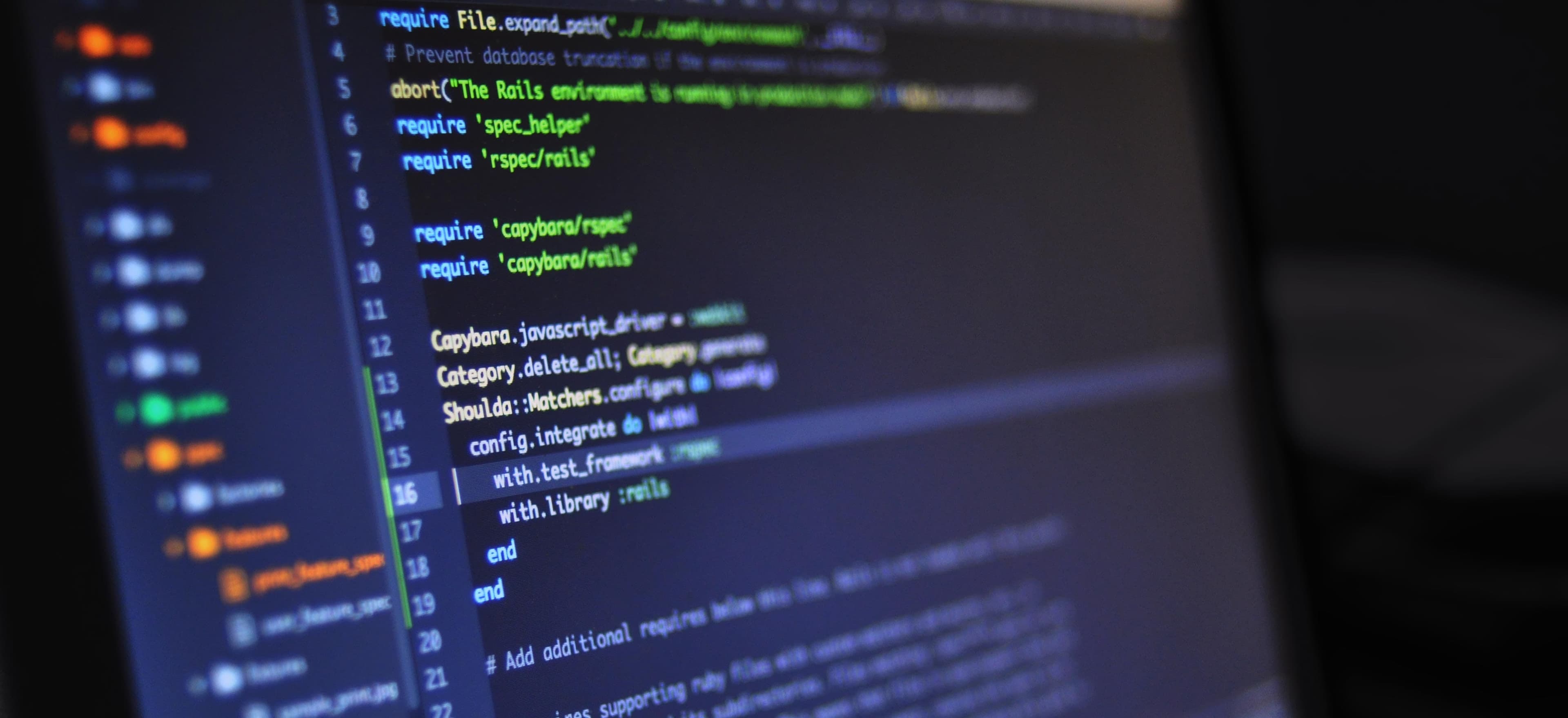
- Published on
Unlocking Java Puzzles: Common Pitfalls for OCA Exam
The Oracle Certified Associate (OCA) Java SE Programmer certification is a gateway for many aspiring developers. However, navigating through the complexity of Java can be challenging. This post will delve into common pitfalls that candidates often encounter during their preparation. We aim to unravel these puzzles and guide you in mastering the nuances of the Java programming language.
Understanding the OCA Exam Structure
Before diving into common pitfalls, it's crucial to understand the structure of the OCA exam. The OCA exam primarily covers:
- Java Basics
- Working with Java Data Types
- Operators and Control Flow
- Making Decisions
- Working with Arrays
- Using Objects
- Inheritance
- Encapsulation and Polymorphism
The Importance of Clear Understanding
Having a clear understanding of these foundational concepts is imperative. Candidates often struggle with Java's object-oriented basics, arrays, and exception handling, leading to confusion. Let's explore these areas and examine common pitfalls.
Pitfall 1: Misunderstanding Java Basics
A strong grasp of Java basics lays the groundwork for successful programming. One frequent mistake is conflating Java's data types.
Example: What are Primitive Types?
Java has two major categories of data types: primitive types and reference types.
// Primitive types
int age = 30;
double salary = 50000.99;
char initial = 'J';
boolean isEmployed = true;
Why It Matters:
These primitive types are the building blocks for data manipulation in Java, and a clear understanding here is essential for developing efficient code.
Common Confusion: Wrapper Classes
Candidates often get confused between primitive types and their corresponding wrapper classes.
// Wrapper types
Integer ageWrapper = Integer.valueOf(age);
Double salaryWrapper = Double.valueOf(salary);
Character initialWrapper = Character.valueOf(initial);
Boolean isEmployedWrapper = Boolean.valueOf(isEmployed);
Why It Matters:
Wrapper classes provide a way to use primitive data types as objects, allowing for features such as null values and methods.
Pitfall 2: Inefficiency with Control Flow Statements
Control flow statements, including loops and conditionals, often trip up candidates.
// Example of a for loop
for (int i = 0; i < 5; i++) {
System.out.println("The number is: " + i);
}
Key Point:
Using loops effectively is crucial, especially when working with array and collection processing. Always ensure to understand how the loop's condition influences its execution.
Common Mistake: Falling into Infinite Loops
An infinite loop can result from a poorly defined loop condition:
// This is an infinite loop
for (int i = 0; i < 5; ) {
System.out.println("This will never stop!");
}
Why It Matters:
This type of infinite loop is a superfluous pitfall that can complicate testing. Always ensure you increment your loop variable correctly.
Pitfall 3: Arrays Are Not Lists or Collections
Java's arrays are fixed in size, which can mislead those new to Java.
Creating and Using Arrays
// Declaration and initialization of an array
int[] numbers = new int[5];
numbers[0] = 10;
// Note that you need to declare the size once
Common Misunderstanding:
Many see arrays similarly to lists in higher-level languages, forgetting that Java arrays require a predefined size.
Example: Fixing Array Index Issues
try {
// Accessing an index that does not exist
System.out.println(numbers[5]); // This will throw an ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException e) {
System.err.println("Array index is out of bounds!");
}
Why It Matters:
Always validate array access to avoid exceptions. Understanding the bounds of your data structures will help in writing robust applications.
Pitfall 4: Object-Oriented Principles
Many exam candidates overlook the principles of encapsulation and polymorphism.
Encapsulation
Ensuring that class fields are private while providing public getters and setters encapsulates data, promoting data integrity.
public class Employee {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Why It Matters:
Encapsulation is fundamental in creating maintainable code. Accessing data via methods ensures validation and protects against unintended manipulation.
Polymorphism
Understanding polymorphism through method overloading and overriding is vital.
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
Why It Matters:
This flexibility allows for an enhanced interface for code, which is a crucial concept in Java programming and a frequent exam topic.
Pitfall 5: Exception Handling Mechanisms
Java features robust exception handling, which can be daunting.
Essential Try-Catch Blocks
try {
int division = 10 / 0;
} catch (ArithmeticException e) {
System.err.println("Cannot divide by zero!");
}
Why It Matters:
Effective use of exception handling can help create resilient applications. Always catch specific exceptions rather than a generic Exception type for clarity.
Common Mistake: Ignoring Finally Block
Omitting the finally block can lead to missed clean-up operations.
try {
// Operations that may throw an exception
} catch (Exception e) {
// Handle the exception
} finally {
// Always executed
System.out.println("Cleanup actions, if any.");
}
Why It Matters:
The finally block is crucial for ensuring that certain actions occur whether exceptions are thrown or not.
To Wrap Things Up
Preparing for the OCA Java exam can be a turbulent journey, but knowing these common pitfalls can turn the tide in your favor. Emphasizing a solid understanding of Java's foundational concepts – from data types to object-oriented principles and exception handling – can drastically improve your proficiency.
Additional Resources
If you're looking for more comprehensive study materials, consider exploring the following:
- Oracle's Official OCA Study Guide
- Java Programming and Software Engineering Fundamentals on Coursera
By preparing diligently and acknowledging these pitfalls, you'll be well on your way to achieving certification and embracing your journey as a Java developer. Happy coding!