Optimizing Data Processing with Java 16 Stream MapMulti
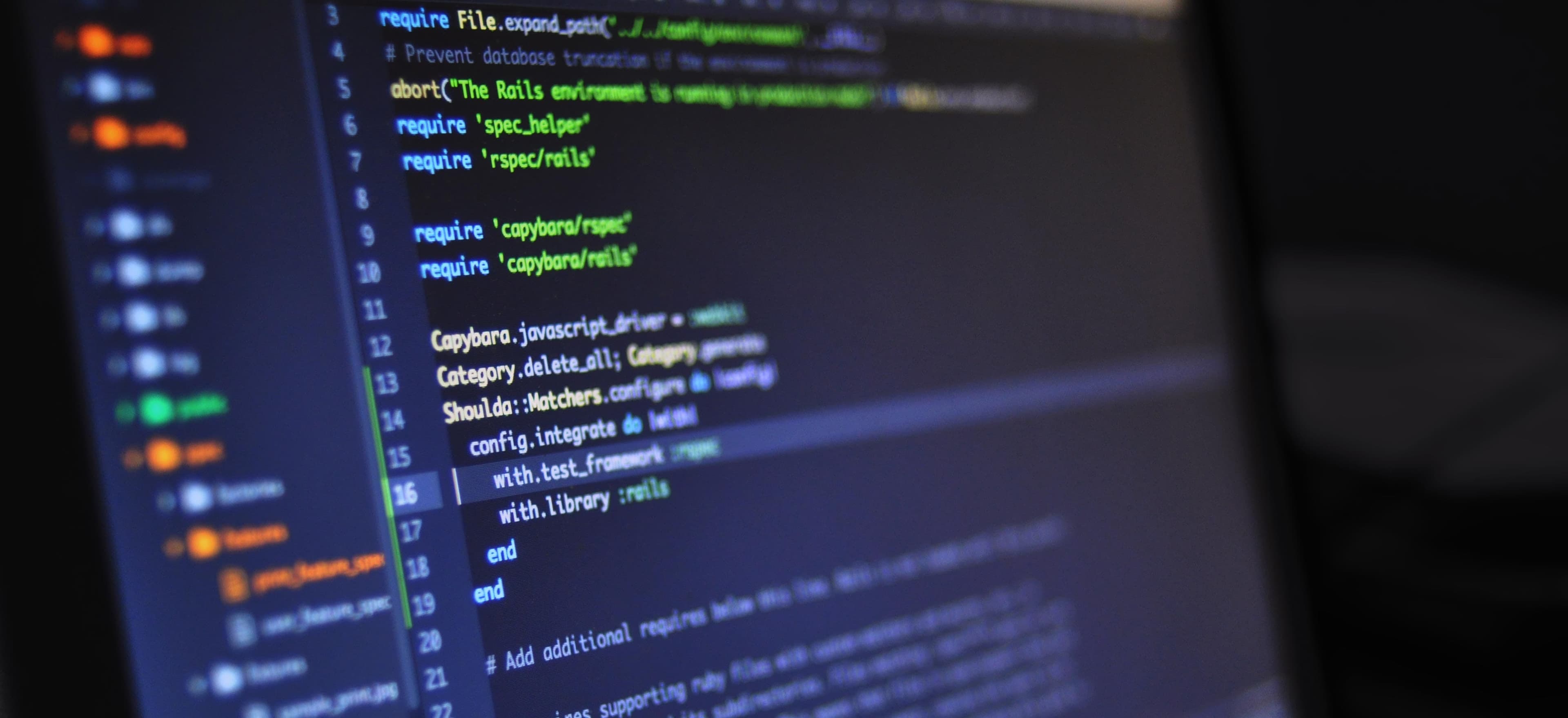
- Published on
Optimizing Data Processing with Java 16 Stream mapMulti
Data processing is a crucial aspect of any software application, and in Java, the Stream API has been a powerful tool for performing operations on collections of data. Java 16 introduced the mapMulti
method for streams, which adds a new dimension to how we can optimize data processing. In this post, we'll explore the mapMulti
method and its benefits in optimizing data processing in Java applications.
Understanding mapMulti
The mapMulti
method in Java 16 Stream API is a powerful addition that allows for the transformation and flattening of elements in a stream. It operates on each element of the stream and emits zero or more elements to the downstream consumer. This capability enables more efficient processing of data, especially when dealing with complex transformations and operations on elements within a stream.
Benefits of mapMulti
The mapMulti
method offers several benefits for optimizing data processing in Java applications:
-
Efficient Data Transformation:
mapMulti
provides a more efficient way to transform and process data within a stream by allowing the emission of multiple elements per input element. -
Improved Performance: By enabling a more streamlined processing workflow,
mapMulti
can lead to performance improvements, especially when dealing with large datasets. -
Simplified Code: It can lead to more concise and readable code compared to traditional approaches, making the data processing logic easier to understand and maintain.
Now, let's delve into some practical examples to understand how mapMulti
can be used to optimize data processing.
Example Usage of mapMulti
Consider a scenario where we have a collection of orders, and we want to transform each order into a set of its individual items. Traditionally, we might use nested loops or complex mapping operations to achieve this. However, with mapMulti
, we can simplify this process.
import java.util.List;
import java.util.stream.Stream;
public class Order {
private List<String> items;
// Assume constructor and other methods are implemented
public Stream<String> flatMapItems() {
return items.stream().mapMulti((item, consumer) -> {
for (String word : item.split("\\s+")) {
consumer.accept(word);
}
});
}
}
In this example, the flatMapItems
method uses mapMulti
to efficiently transform each order into a stream of its individual items. The mapMulti
function takes a consumer that allows the emission of multiple elements for each input element.
When to Use mapMulti
mapMulti
is particularly useful when you need to perform operations that result in multiple output elements for each input element in the stream. This could include scenarios such as parsing, flattening, or complex transformation of data.
By leveraging the mapMulti
method, you can streamline data processing and improve the overall efficiency of your Java applications.
Comparing map
vs mapMulti
To further illustrate the benefits of mapMulti
, let's compare it with the traditional map
method in the context of data processing.
// Using map to split and flatten words from a sentence
String sentence = "The quick brown fox";
List<String> words = Arrays.stream(sentence.split("\\s+"))
.map(word -> word.split(""))
.flatMap(Arrays::stream)
.collect(Collectors.toList());
// Using mapMulti to achieve the same result
Stream<String> wordStream = Arrays.stream(sentence.split("\\s+"))
.mapMulti((word, consumer) -> {
for (String letter : word.split("")) {
consumer.accept(letter);
}
});
List<String> letters = wordStream.collect(Collectors.toList());
In this comparison, we see that while both map
and mapMulti
can achieve the same result, the code utilizing mapMulti
presents a more straightforward and concise approach, especially when dealing with complex transformations or operations on elements within the stream.
Final Thoughts
In conclusion, Java 16's mapMulti
method brings a valuable addition to the Stream API, offering a more efficient and streamlined approach to data processing. By enabling the emission of multiple elements for each input element in a stream, mapMulti
simplifies complex transformations and operations, leading to improved performance and more readable code.
When optimizing data processing in Java applications, it's essential to leverage the capabilities offered by mapMulti
to handle scenarios that involve the generation of multiple output elements for each input element.
As Java continues to evolve, the additions and improvements to the Stream API, such as mapMulti
, demonstrate the language's commitment to enhancing data processing capabilities and performance.
Incorporating the mapMulti
method into your data processing workflows can lead to more efficient and maintainable code, ultimately contributing to the overall performance and scalability of your Java applications.
To further explore the capabilities of Java streams and their optimization, check out the official Java documentation and Java Stream API for additional insights and best practices.
By leveraging mapMulti
and other powerful features of Java streams, you can take your data processing capabilities to the next level and unlock new efficiencies in your applications.