Improving Search Results with Hibernate Search 4.2
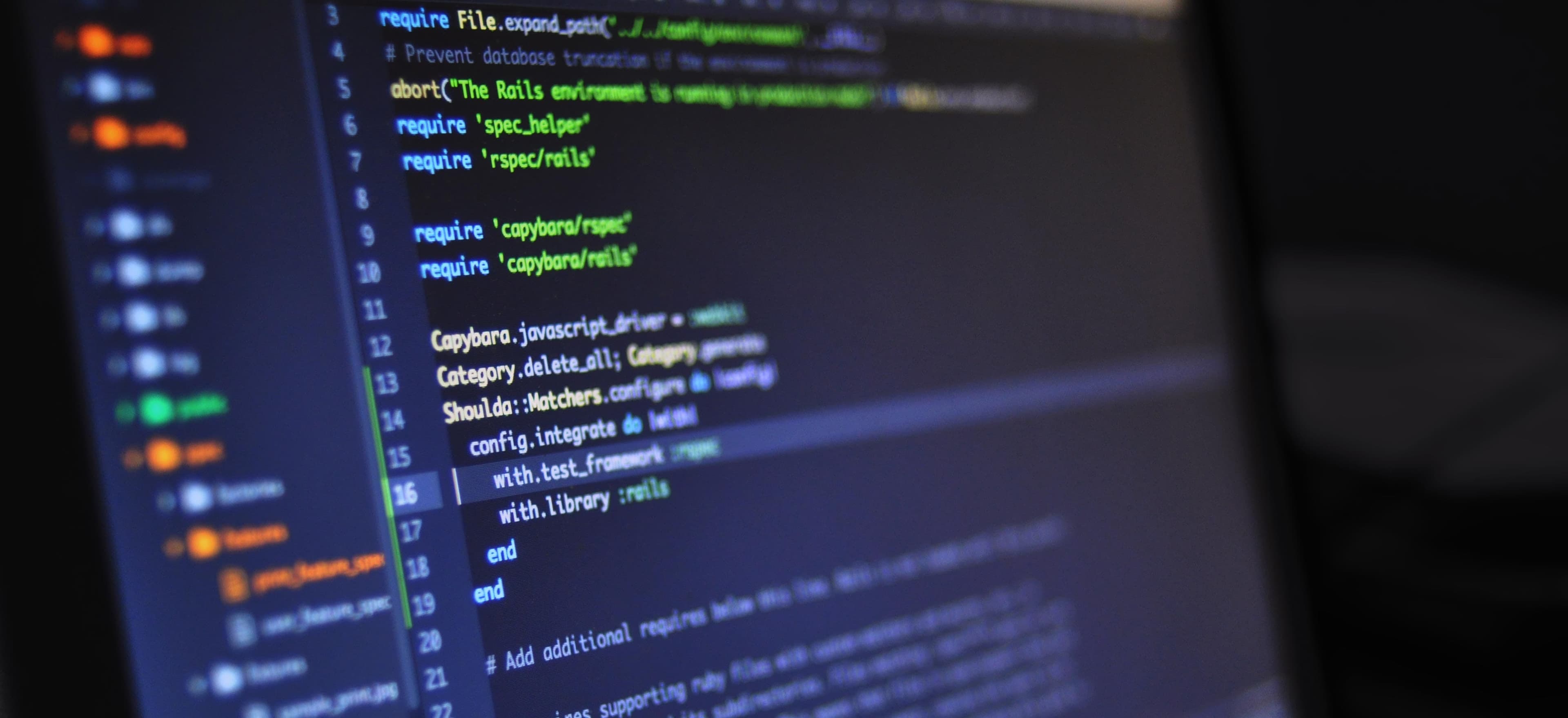
- Published on
Improving Search Results with Hibernate Search 4.2
In the world of enterprise applications, efficient search functionality is pivotal for providing a seamless user experience, and Hibernate Search 4.2 is a powerful tool for achieving this. By integrating full-text search capabilities into the Hibernate ORM framework, developers can enhance the search functionality of their applications.
In this article, we will delve into the features of Hibernate Search 4.2 and demonstrate how it can be leveraged to improve search results in Java applications.
What is Hibernate Search 4.2?
Hibernate Search 4.2 is an extension of the Hibernate ORM framework that provides full-text search capabilities. It seamlessly integrates with Hibernate ORM and enables developers to perform advanced search operations on entities using Lucene indexes.
Setting up Hibernate Search 4.2
To begin using Hibernate Search 4.2, you need to add the necessary dependencies to your project. Below is a sample Maven dependency configuration for Hibernate Search 4.2:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-search</artifactId>
<version>4.2.0.Final</version>
</dependency>
Additionally, you'll need to define the Hibernate Search configuration in your persistence.xml
or hibernate.cfg.xml
file to enable indexing and searching.
Indexing Entities with Hibernate Search
After setting up the dependencies and configuration, you can annotate your entity classes to enable indexing with Hibernate Search. By marking the fields to be included in the search index, you can provide efficient full-text search capabilities for those entities.
Consider the following example of indexing a Product
entity using Hibernate Search annotations:
@Entity
@Indexed
public class Product {
@Id
@GeneratedValue
private Long id;
@Field(index = Index.YES, analyze = Analyze.YES, store = Store.NO)
private String name;
@Field(index = Index.YES, analyze = Analyze.YES, store = Store.NO)
private String description;
// Getters and setters
}
In this example, the @Indexed
annotation marks the Product
entity for indexing, while the @Field
annotations specify the fields to be included in the search index and define the indexing behavior for each field.
Performing Full-Text Searches
Once the entities are indexed using Hibernate Search, you can perform full-text searches using the Hibernate Search APIs. By leveraging the Lucene query capabilities, developers can execute complex search queries against the indexed entities.
Let's consider an example where we perform a full-text search for products based on a user-provided keyword:
FullTextEntityManager fullTextEntityManager = Search.getFullTextEntityManager(entityManager);
QueryBuilder queryBuilder = fullTextEntityManager.getSearchFactory()
.buildQueryBuilder()
.forEntity(Product.class)
.get();
Query luceneQuery = queryBuilder
.keyword()
.onFields("name", "description")
.matching("laptop")
.createQuery();
FullTextQuery fullTextQuery = fullTextEntityManager.createFullTextQuery(luceneQuery, Product.class);
List<Product> results = fullTextQuery.getResultList();
In this example, we obtain the FullTextEntityManager
from the standard EntityManager
and use the QueryBuilder
to construct a Lucene query for searching products based on the name
and description
fields. The resulting list contains the products matching the search query.
Optimizing Search Results
To optimize search results with Hibernate Search 4.2, there are several strategies and techniques to consider:
1. Analyzing and Indexing Fields
Proper analysis and indexing configuration for fields play a vital role in the relevance and accuracy of search results. Understanding how to configure the @Field
annotations to suit the nature of the data being indexed is crucial.
2. Utilizing Filters
Hibernate Search provides the ability to apply filters to search queries, allowing developers to narrow down the search results based on specific criteria. This can significantly improve the precision of search results.
3. Faceting
Faceting is a powerful feature in Hibernate Search that enables developers to provide aggregated results based on criteria. By using faceting, you can offer users insights into the distribution of search results, leading to a more interactive search experience.
4. Performance Optimization
Efficient use of caching, tuning indexing strategies, and employing advanced optimization techniques offered by Hibernate Search can significantly enhance the performance of search operations in your application.
By incorporating these strategies, you can ensure that the search functionality powered by Hibernate Search 4.2 delivers accurate and relevant results, contributing to an exceptional user experience.
A Final Look
Hibernate Search 4.2 is a valuable tool for improving search results in Java applications. By seamlessly integrating with Hibernate ORM and leveraging the power of Lucene indexing, it empowers developers to implement efficient and robust search functionality.
In this article, we explored the basic setup and usage of Hibernate Search 4.2, along with strategies for optimizing search results. By employing Hibernate Search 4.2, developers can elevate the search capabilities of their applications, resulting in a more satisfying user experience.
To learn more about Hibernate Search 4.2, visit the official documentation.
Experience the difference with Hibernate Search 4.2 and take your application's search functionality to the next level!