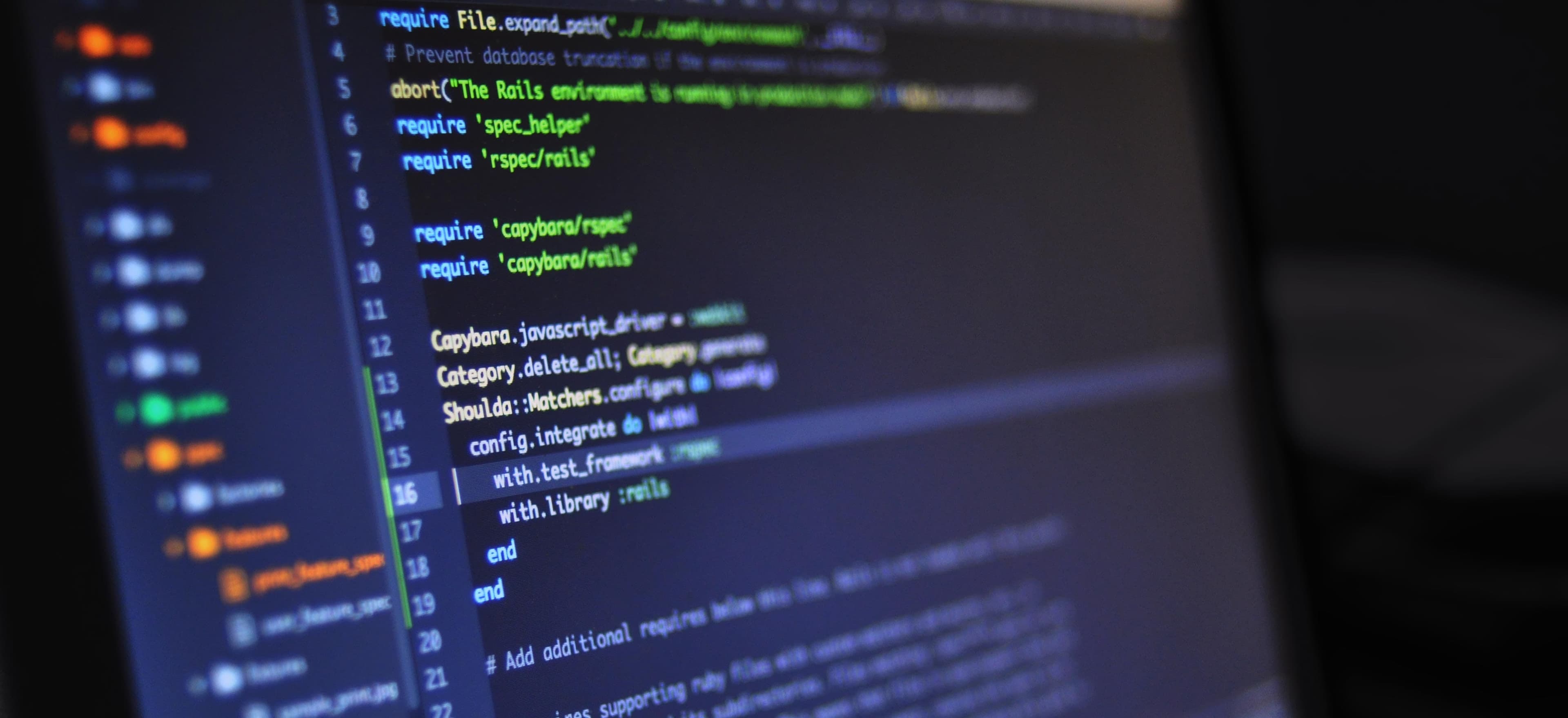
- Published on
In the world of computer-aided design (CAD), Java has cemented its position as a reliable programming language for developing robust and versatile applications. With the introduction of JavaFX, creating visually appealing and interactive user interfaces has become more streamlined and efficient. In this blog post, we will explore the process of building a CAD application using JavaFX, leveraging its powerful features to deliver a compelling user experience.
Why Choose JavaFX for CAD Applications?
JavaFX provides a rich set of features for creating modern user interfaces, including 2D and 3D graphics, multimedia, and animation capabilities. Its extensive library of UI controls and powerful styling options make it a suitable choice for developing CAD applications that require a high degree of interactivity and visual sophistication.
The ability to seamlessly integrate JavaFX with Java's robust backend capabilities allows developers to design CAD applications that are not only visually appealing but also highly functional and efficient in handling complex operations such as rendering, geometry manipulation, and data management.
Setting Up the Development Environment
Before diving into the development process, it's crucial to ensure that the required tools and dependencies are set up for JavaFX application development. You can use popular integrated development environments (IDEs) such as IntelliJ IDEA or Eclipse, both of which offer robust support for JavaFX projects.
Additionally, ensure that the Java Development Kit (JDK) is installed on your system, as JavaFX is now bundled with the JDK, starting from JDK 11. This simplifies the setup process for JavaFX development, as there is no need to manage separate libraries or dependencies.
Creating the CAD Application Skeleton
To kick off the development process, let's create the basic structure of our CAD application using JavaFX. We'll start with a simple JavaFX application class that sets up the primary stage and initializes the application window.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class CADApplication extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("JavaFX CAD Application");
BorderPane root = new BorderPane();
Scene scene = new Scene(root, 800, 600);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this initial setup, we've created a basic JavaFX application that displays a window with a title and a predefined size. The BorderPane
serves as the root node for the application's layout, providing areas for placing the main components of the CAD interface.
Implementing 2D and 3D Graphics Capabilities
CAD applications often require sophisticated 2D and 3D graphics rendering capabilities to visualize complex designs and models. JavaFX offers a powerful set of APIs for rendering graphics, including geometric shapes, transformations, and scene graph manipulation.
Let's take a look at a simple example of drawing a 2D shape, such as a rectangle, and a 3D shape, such as a box, within our CAD application.
import javafx.scene.shape.Rectangle;
import javafx.scene.shape.Box;
import javafx.scene.paint.Color;
import javafx.scene.transform.Rotate;
import javafx.scene.transform.Translate;
// Inside the start method of CADApplication class
Rectangle rectangle = new Rectangle(100, 100);
rectangle.setFill(Color.BLUE);
rectangle.setX(100);
rectangle.setY(100);
root.getChildren().add(rectangle); // Adding the rectangle to the root pane
Box box = new Box(100, 100, 100);
box.setMaterial(new PhongMaterial(Color.RED));
box.getTransforms().addAll(
new Translate(300, 150, 0),
new Rotate(30, Rotate.Y_AXIS)
);
root.getChildren().add(box); // Adding the box to the root pane
In the above code snippet, we create and configure a 2D rectangle and add it to the root pane of the application. Similarly, we create a 3D box, apply transformations to it, and then add it to the root pane for 3D visualization within the CAD application.
Interactivity and User Input Handling
An effective CAD application should support user interactions such as mouse events, keyboard input, and gestures for seamless design manipulation. JavaFX provides robust event handling mechanisms that enable developers to capture and respond to user input effectively.
Let's incorporate a simple mouse event handler to capture the user's interaction with a 2D shape, allowing them to move the shape within the application window.
// Inside the start method of CADApplication class
rectangle.setOnMousePressed(event -> {
// Save the initial mouse cursor position relative to the shape's position
rectangle.setUserData(new Point2D(event.getSceneX() - rectangle.getX(), event.getSceneY() - rectangle.getY()));
});
rectangle.setOnMouseDragged(event -> {
// Update the shape's position based on the mouse movement
double offsetX = event.getSceneX() - (double) rectangle.getUserData();
double offsetY = event.getSceneY() - (double) rectangle.getUserData().getY();
rectangle.setX(offsetX);
rectangle.setY(offsetY);
});
In this example, we add event handlers to the 2D rectangle to track the user's mouse press and drag actions, updating the position of the shape accordingly. This simple implementation demonstrates the seamless interactivity that JavaFX facilitates for CAD applications.
Integrating Data Models and Persistence
For CAD applications, managing complex data models and providing options for data persistence are crucial aspects. JavaFX, in conjunction with Java's extensive support for data handling and storage, enables developers to integrate data models into their CAD applications and facilitate data persistence.
One common approach is to use Java's object-oriented capabilities to model geometric entities and their properties, such as coordinates, dimensions, and transformations. Additionally, Java provides robust support for data serialization and storage, allowing developers to save and load CAD designs efficiently.
public class GeometricEntity implements Serializable {
private double x;
private double y;
// Other properties and methods
// Serialization support
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
}
}
In this example, we create a simple GeometricEntity
class that represents a geometric entity in the CAD application. By implementing the Serializable
interface and defining serialization methods, we enable the object to be serialized for storage and deserialized for retrieval, thus supporting data persistence within the CAD application.
The Last Word
In this blog post, we've explored the process of building a CAD application using JavaFX, leveraging its powerful features for creating visually compelling and interactive user interfaces. From setting up the development environment to implementing 2D and 3D graphics, handling user interactions, and integrating data models, JavaFX offers a comprehensive toolkit for developing sophisticated CAD applications.
As the Java ecosystem continues to evolve, JavaFX remains a strong contender for building modern and feature-rich CAD applications, demonstrating its versatility in addressing the demanding requirements of design and engineering software.
By harnessing the capabilities of JavaFX in conjunction with Java's backend prowess, developers can craft CAD applications that not only meet the visual and interactive demands of users but also deliver robust functionality, efficiency, and data management capabilities, making JavaFX a compelling choice for CAD application development.
Start your journey into JavaFX and pave the way for crafting compelling CAD applications that marry powerful graphics capabilities with seamless user interactions, setting new benchmarks for design and engineering software.