Understanding Java Persistence Locking Mechanism
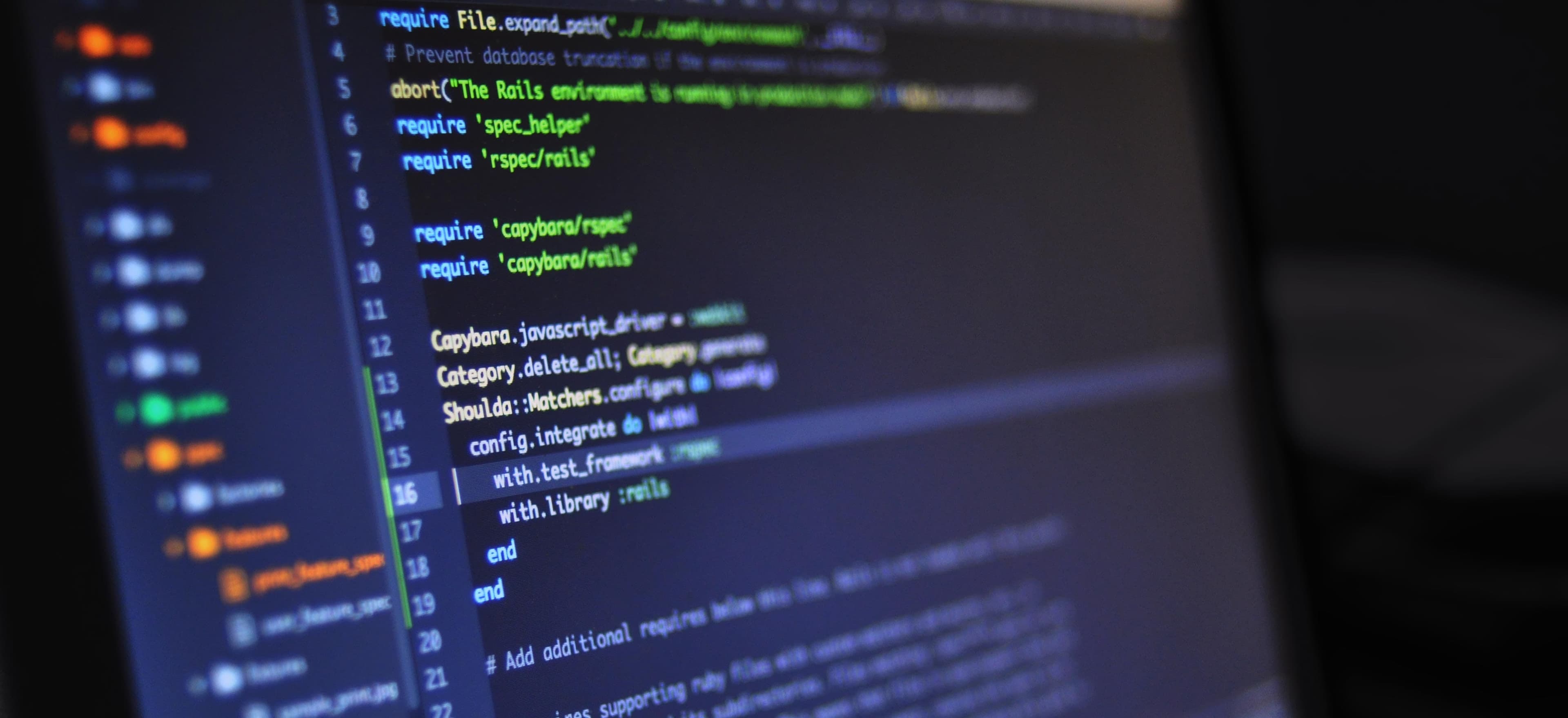
- Published on
Understanding Java Persistence Locking Mechanism
In Java Persistence, locking plays a crucial role in managing concurrent access to shared resources, ensuring data integrity, and preventing conflicts among multiple transactions. In this blog post, we'll explore the concept of locking in Java Persistence, its various types, and how to use them effectively in your applications.
What is Locking in Java Persistence?
Locking in Java Persistence refers to the mechanism for controlling access to database records to prevent concurrent transactions from interfering with each other. It ensures that only one transaction can modify a particular database record at a time, thereby maintaining data consistency and integrity.
Types of Locking in Java Persistence
1. Optimistic Locking
Optimistic locking is a strategy where the database record is not locked during the read operation. Instead, a version number or timestamp is used to detect conflicting updates. When a transaction attempts to update the record, the version or timestamp is checked to verify if the record has been modified by another transaction since it was read. If no conflict is detected, the update is allowed; otherwise, an exception is thrown.
Example of Optimistic Locking with JPA
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Version
private int version;
// other fields and methods
}
In the above example, the @Version
annotation is used to enable optimistic locking for the Product
entity. When a concurrent update occurs, the version field is checked to detect conflicts.
2. Pessimistic Locking
Pessimistic locking involves explicitly acquiring a lock on a database record for the duration of a transaction. This prevents other transactions from modifying the locked record until the lock is released. Pessimistic locking can be further categorized as:
- READ lock: Prevents other transactions from obtaining a WRITE lock on the same record.
- WRITE lock: Prevents other transactions from obtaining either READ or WRITE locks on the same record.
Example of Pessimistic Locking with JPA
entityManager.find(Product.class, productId, LockModeType.PESSIMISTIC_WRITE);
In this example, LockModeType.PESSIMISTIC_WRITE
is used to acquire a pessimistic WRITE lock on the Product
record with the specified productId
.
When to Use Optimistic Locking vs. Pessimistic Locking
Optimistic Locking
- Use Case: When the likelihood of concurrent updates is low.
- Pros: Minimal impact on system performance, as locks are not held during read operations.
- Cons: May lead to more frequent conflicts and retries in high-concurrency scenarios.
Pessimistic Locking
- Use Case: When the likelihood of concurrent updates is high, and potential conflicts need to be minimized.
- Pros: Provides exclusive access to locked records, reducing conflicts in high-concurrency scenarios.
- Cons: Can impact system performance due to locks being held for an extended period.
Best Practices for Using Locking in Java Persistence
-
Choose the Right Locking Strategy: Consider the concurrency requirements of your application to determine whether optimistic or pessimistic locking is more suitable.
-
Avoid Long-Lasting Transactions: Pessimistic locks should be used judiciously to prevent prolonged blocking of resources and potential deadlock situations.
-
Optimize Entity Versioning: When using optimistic locking, ensure that entity versioning is implemented effectively to minimize conflicts and retries.
-
Handle Locking Exceptions: When using optimistic locking, handle
OptimisticLockException
gracefully to retry the operation or notify the user about the conflict.
Summary
In Java Persistence, locking is a fundamental aspect of managing concurrent access to shared resources. Understanding the various types of locking mechanisms, including optimistic and pessimistic locking, and their appropriate usage is essential for developing robust and efficient database-driven applications.
By incorporating the right locking strategy based on your application's concurrency requirements and adhering to best practices, you can ensure data integrity, minimize conflicts, and optimize the performance of your Java Persistence applications.
To delve deeper into Java Persistence and its locking mechanisms, check out the official documentation for Java Persistence API (JPA).
Happy coding!