Unlocking the Power of Optional Class in Java 8
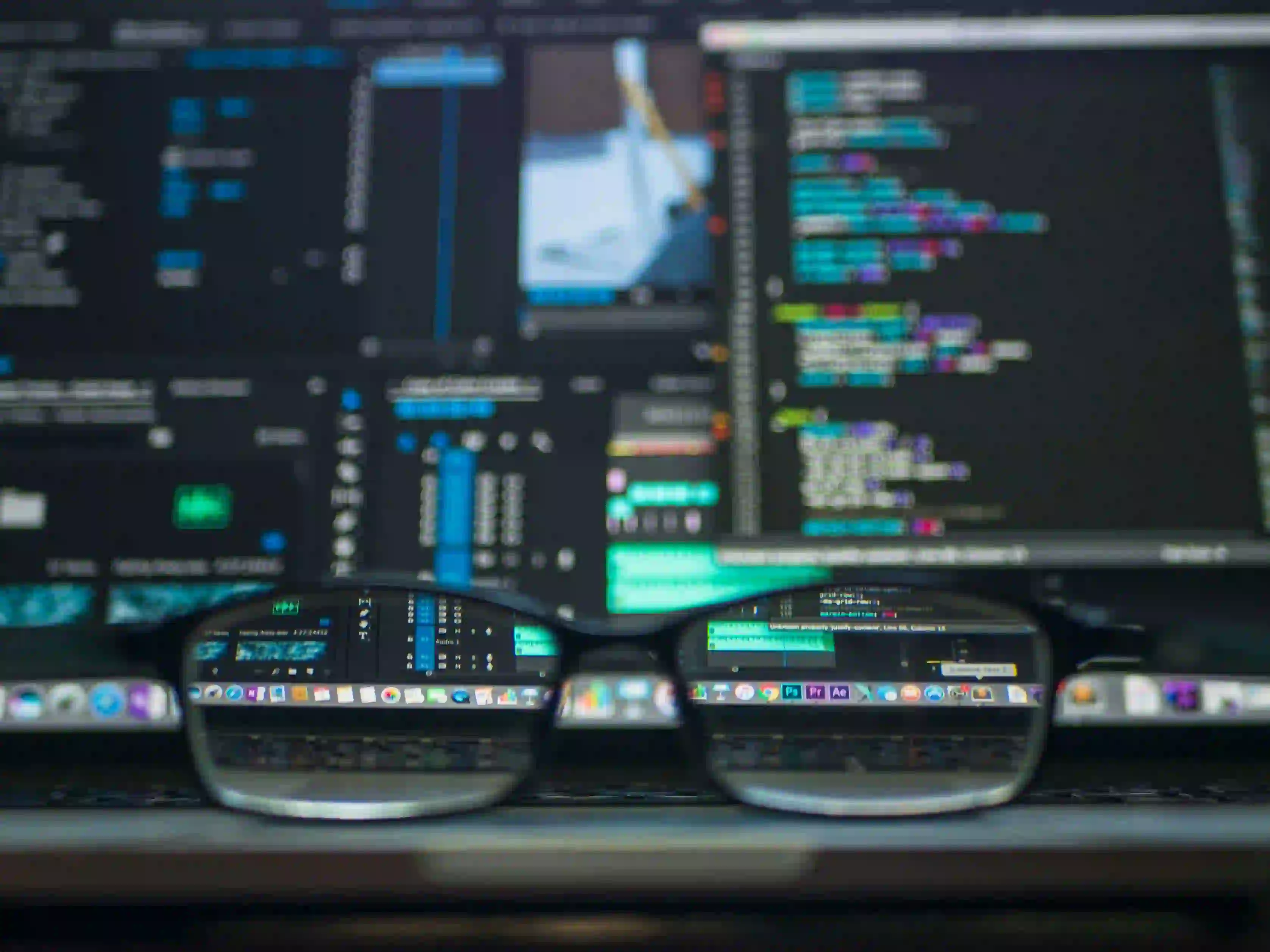
In Java programming, the Optional class was introduced in Java 8 to address the problem of null references. Dealing with nulls in code can lead to unexpected NullPointerExceptions, which can be a source of frustration for developers. The Optional class provides a way to work with values that may or may not be present, without explicitly using null.
What is the Optional class?
The Optional class is a container object that may or may not contain a non-null value. It is a more elegant way of handling the absence of a value without explicitly using null and writing conditional checks for null values. Optional provides methods for dealing with the presence or absence of a value, allowing you to write more concise and expressive code.
Why use Optional?
Using Optional can help to make your code more readable and less error-prone. It encourages developers to explicitly handle the case where a value might be absent, rather than allowing NullPointerExceptions to occur. By using Optional, you are forced to acknowledge the possibility of a value being absent and handle it appropriately.
Creating Optional objects
You can create an Optional object using its static methods. For example, to create an Optional object with a non-null value, you can use the of
method:
Optional<String> optional = Optional.of("Hello, world!");
If you are unsure whether the value is null, you can use the ofNullable
method to create an Optional object that may or may not contain a non-null value:
String nullableValue = possiblyNullMethod();
Optional<String> optional = Optional.ofNullable(nullableValue);
Accessing values from Optional
To retrieve the value from an Optional object, you can use the get
method:
Optional<String> optional = Optional.of("Hello, world!");
String value = optional.get();
However, it is recommended to avoid using get
directly, as it may result in NoSuchElementException if the value is not present. Instead, you can use methods like isPresent
and ifPresent
to safely access the value:
Optional<String> optional = Optional.of("Hello, world!");
if (optional.isPresent()) {
String value = optional.get();
System.out.println(value);
}
Or, using the ifPresent
method for a more concise approach:
Optional<String> optional = Optional.of("Hello, world!");
optional.ifPresent(System.out::println);
The ifPresent
method takes a Consumer as an argument and executes the Consumer if the value is present.
Handling absence of value
When a value is absent, you can specify a default value using the orElse
method:
String nullValue = null;
Optional<String> optional = Optional.ofNullable(nullValue);
String value = optional.orElse("Default Value");
System.out.println(value); // Output: Default Value
Another option is to provide a supplier for the default value, which is only invoked if the value is absent:
String nullValue = null;
Optional<String> optional = Optional.ofNullable(nullValue);
String value = optional.orElseGet(() -> "Default Value");
System.out.println(value); // Output: Default Value
Chaining Optional methods
You can chain multiple Optional methods together to perform sequential operations on the value. This allows for concise and readable code.
For example, you can combine map
and orElse
methods to manipulate the value if present or provide a default value if absent:
String name = possiblyNullName();
String defaultName = "Guest";
String result = Optional.ofNullable(name)
.map(String::toUpperCase)
.orElse(defaultName);
System.out.println(result);
In this example, if the name
is not null, it will be converted to uppercase using the map
method. If it is null, the orElse
method provides a default name.
Advantages of Optional
-
Reduced NullPointerExceptions: By using Optional, you can significantly reduce the occurrence of NullPointerExceptions in your code, leading to more robust and reliable applications.
-
Readability and Expressiveness: Optional encourages developers to handle the possibility of absent values explicitly, leading to more readable and expressive code.
-
Forced Encapsulation: Optional forces you to encapsulate the handling of absent values, making it easier to reason about the code and avoid unexpected bugs.
A Final Look
In conclusion, the Optional class in Java 8 provides a powerful way to handle the absence of values in a more explicit and concise manner. By embracing Optional, developers can write cleaner, safer, and more readable code, ultimately leading to more maintainable and reliable applications.
When used appropriately, Optional can unlock the potential for more robust error handling and improve the overall quality of Java code. Embracing Optional is a step towards writing cleaner and safer Java code.
So, the next time you encounter a situation where a value may or may not be present, consider using Optional to unlock its power and elevate the quality of your Java code.
Remember, embracing Optional is not only about writing safer code, but also about shaping a more robust and predictable codebase.
For more information on the Optional class and its methods, you may refer to the Java 8 Optional documentation for comprehensive details and examples.