Efficient Sorting with Redis and Jedis
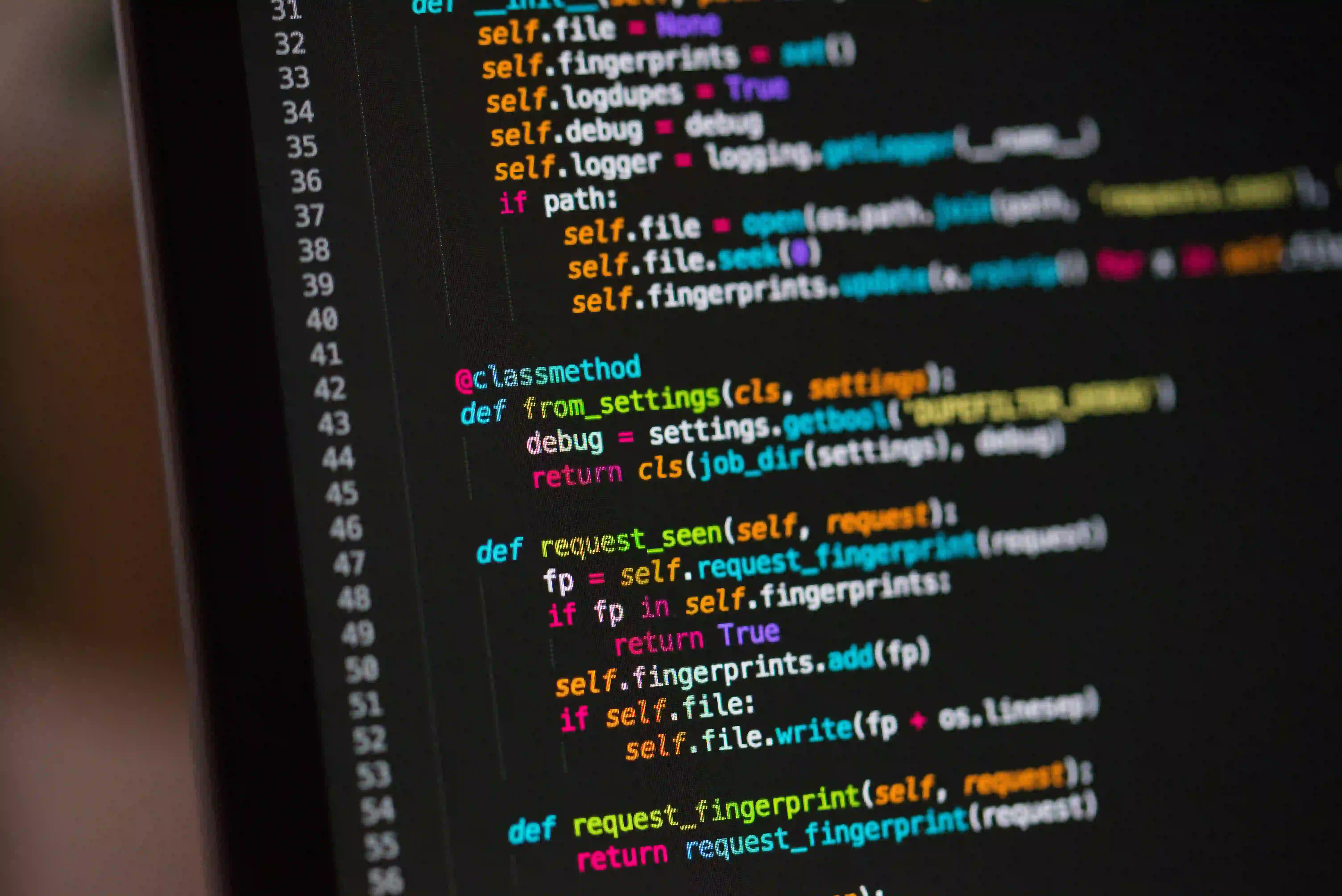
Efficient Sorting with Redis and Jedis
Sorting is a fundamental operation in programming, and when dealing with large datasets, the need for efficient sorting becomes even more crucial. In this blog post, we will explore how Redis, a popular in-memory data store, can be used for efficient sorting, and how the Java Redis client, Jedis, can be utilized to interact with Redis from a Java application.
What is Redis?
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, and sorted sets, and provides high-performance capabilities for data manipulation and retrieval.
Introducing Jedis
Jedis is a Java Redis client that provides a high-level interface for interacting with a Redis server from a Java application. It enables Java developers to perform various operations such as setting and getting values, working with transactions, and executing commands on Redis.
Sorting with Redis
Redis provides a powerful SORT
command that allows sorting of elements in a list, set, or sorted set based on certain criteria. When used in conjunction with the power of Redis data structures, the SORT
command can efficiently handle large datasets and perform complex sorting operations.
Let's delve into a practical example of how to use the SORT
command in Redis to perform efficient sorting.
First, let's assume we have a Redis list named user_ids
containing user identifiers, and a corresponding Redis hash for each user, representing user details such as name and age.
try (Jedis jedis = new Jedis("localhost")) {
// Populating the user_ids list
jedis.lpush("user_ids", "1001", "1002", "1003", "1004");
// Storing user details in Redis hashes
jedis.hset("user:1001", "name", "Alice");
jedis.hset("user:1001", "age", "25");
jedis.hset("user:1002", "name", "Bob");
jedis.hset("user:1002", "age", "30");
jedis.hset("user:1003", "name", "Charlie");
jedis.hset("user:1003", "age", "20");
jedis.hset("user:1004", "name", "Dave");
jedis.hset("user:1004", "age", "27");
}
In this example, we have populated the user_ids
list with user identifiers and stored the user details in Redis hashes with keys user:<id>
. Now, let's use the SORT
command to sort the user identifiers based on the age of the users.
try (Jedis jedis = new Jedis("localhost")) {
String sortedUserIds = jedis.sort("user_ids", SortingParams.by("user:*->age"));
System.out.println(sortedUserIds);
}
In the code snippet above, we are using the SORT
command with the key user_ids
and specifying the sorting order based on the age
field in the Redis hashes. The SORT
command returns the sorted user identifiers, which can be further processed or displayed as required.
By utilizing Redis' SORT
command, we can efficiently sort and retrieve large datasets based on various criteria, leveraging the in-memory processing power of Redis.
Understanding the 'why' behind the code
The SORT
command in Redis offers remarkable flexibility in sorting data based on different criteria. In our Java code, we used the SORT
command to sort user identifiers based on user age. This is a typical real-world scenario where knowing how to leverage Redis for efficient sorting can significantly impact the overall performance of an application.
-
Redis as a Data Sorting Engine: Redis is adept at handling and sorting large datasets due to its in-memory nature, making it well-suited for scenarios where high-performance sorting is required.
-
Reduced Application Complexity: By offloading sorting operations to Redis, we can reduce the computational burden on the application server, leading to simpler and more maintainable application code.
-
Efficient Use of Memory: Leveraging Redis' data structures and in-memory processing for sorting can lead to efficient memory utilization and better overall system performance.
In conclusion, the SORT
command in Redis, paired with Jedis in a Java application, provides a robust mechanism for handling efficient sorting of large datasets, offering benefits in terms of performance, scalability, and code simplicity.
Concluding Thoughts
In this blog post, we explored the power of Redis and Jedis for efficient sorting. By using the SORT
command in Redis and the Jedis Java client, we can leverage Redis' innate ability to handle and sort data with exceptional performance, benefiting various use cases ranging from real-time analytics to high-throughput applications.
Redis and Jedis serve as a compelling combination for enabling efficient sorting capabilities, and understanding the nuances of these tools can undoubtedly enhance the performance and scalability of Java applications dealing with large datasets.
So, the next time you find yourself in need of efficient sorting for your Java application, consider harnessing the capabilities of Redis and Jedis to streamline your data sorting operations.
Start sorting with Redis and Jedis today, and unlock the potential for high-performance sorting in your Java applications!