Troubleshooting Cognito Integration in Spring Boot
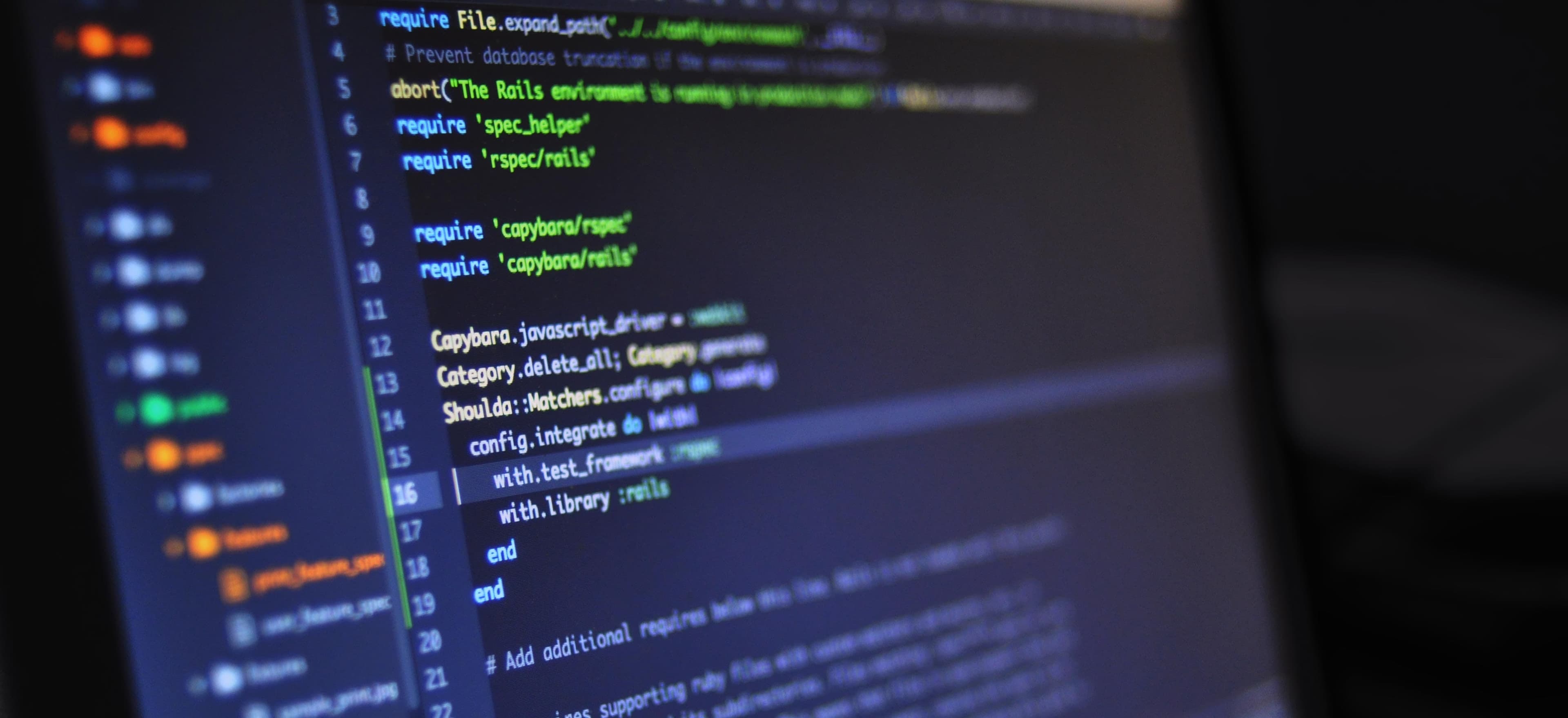
- Published on
Troubleshooting Cognito Integration in Spring Boot
Integrating Amazon Cognito authentication into a Spring Boot application can be a powerful way to secure your APIs and user interfaces. However, like any integration, it can come with its fair share of challenges. This post will cover some common issues and provide solutions for troubleshooting Cognito integration in Spring Boot.
Problem 1: Invalid Access Token
One of the most common issues when integrating with Cognito is receiving an "Invalid Access Token" error when trying to authenticate a user. This can be frustrating, but it's often caused by a simple misconfiguration.
Solution:
Check the audience and issuer of the access token being passed to Cognito. Ensure that the audience matches the client ID of your app in Cognito, and that the issuer is the Cognito User Pool's endpoint.
// Example Cognito JWT Validator
JWTVerifier verifier = JWT.require(Algorithm.HMAC256("secret"))
.withIssuer("https://cognito-idp.us-east-1.amazonaws.com/us-east-1_abcd1234")
.withAudience("your-client-id")
.build();
DecodedJWT jwt = verifier.verify(accessToken);
In the above example, make sure to replace "your-client-id"
with your actual Cognito client ID and "https://cognito-idp.us-east-1.amazonaws.com/us-east-1_abcd1234"
with the correct issuer URL for your Cognito User Pool.
Problem 2: CORS Errors
Another common issue when working with Cognito in a Spring Boot application is encountering CORS (Cross-Origin Resource Sharing) errors, especially when making AJAX requests from the frontend.
Solution:
To solve this, you can configure your Spring Boot application to allow cross-origin requests. One way to do this is by using the @CrossOrigin
annotation on your controller methods.
@RestController
@CrossOrigin
public class MyController {
// Controller methods
}
This will allow cross-origin requests from all domains. For more fine-grained control, you can specify origins and other CORS configuration options within the @CrossOrigin
annotation.
Problem 3: Token Refreshment
When using Cognito for authentication, you might encounter difficulties with token refreshment. Tokens have a limited lifespan, and it's essential to handle token refreshment correctly to avoid disruptions in your application's authentication flow.
Solution:
Implement a token refreshment mechanism in your Spring Boot application. This typically involves capturing the "token expired" error, then using the refresh token to obtain a new access token from Cognito.
AWSCognitoIdentityProvider cognitoIdentityProvider = AWSCognitoIdentityProviderClientBuilder
.standard()
.withRegion(Regions.YOUR_REGION)
.build();
AdminInitiateAuthRequest authRequest = new AdminInitiateAuthRequest()
.withAuthFlow(AuthFlowType.REFRESH_TOKEN)
.withClientId("your-client-id")
.withUserPoolId("your-user-pool-id")
.withAuthParameters(Collections.singletonMap("REFRESH_TOKEN", "your-refresh-token"));
AdminInitiateAuthResult authResult = cognitoIdentityProvider.adminInitiateAuth(authRequest);
String newAccessToken = authResult.getAuthenticationResult().getAccessToken();
// Use the new access token for further requests
Closing the Chapter
Integrating Cognito with a Spring Boot application can be a powerful way to add user authentication and authorization. However, like any integration, it comes with its challenges. By understanding and addressing common issues such as invalid access tokens, CORS errors, and token refreshment, you can ensure a smooth and secure authentication process for your users.
Remember to keep an eye on the audience and issuer of your access tokens, configure CORS settings as needed, and implement a robust token refreshment mechanism for uninterrupted authentication. With these troubleshooting tips in mind, you can optimize your Cognito integration in Spring Boot and provide a seamless user experience for your application.
For further insights into Cognito integration and Spring Boot development, check out the AWS Cognito documentation and the official Spring Boot documentation. These resources offer extensive information and best practices for leveraging Cognito and Spring Boot to their full potential.