Handling API Request Overload: Best Practices
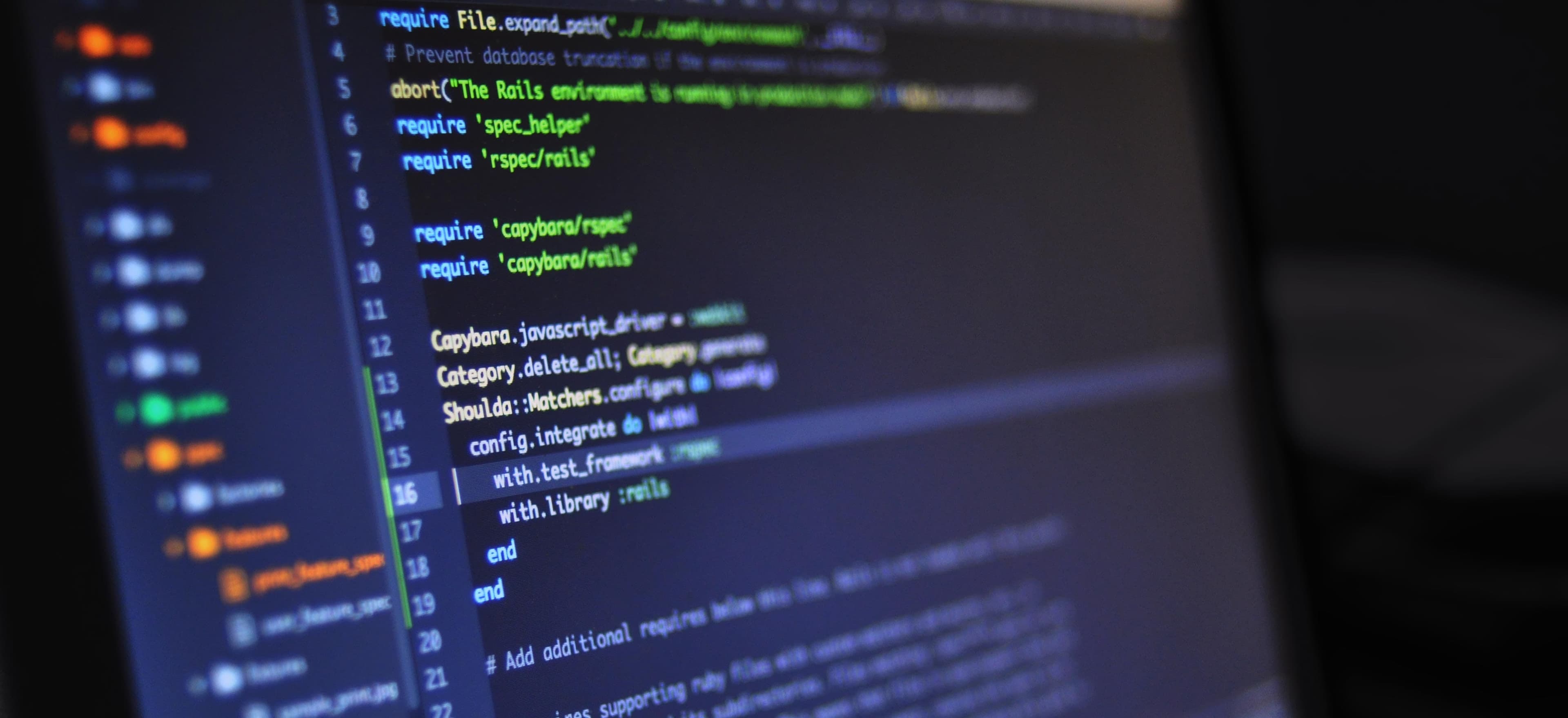
- Published on
Handling API Request Overload: Best Practices
In today's digital environment, APIs (Application Programming Interfaces) play a critical role in enabling communication and data exchange between different software systems. However, as a Java developer, you may encounter a common challenge: handling API request overload. When an API is bombarded with more requests than it can effectively process, it can lead to performance degradation, downtime, and unhappy users. Therefore, it's essential to implement best practices to handle API request overload effectively. In this article, we'll explore some of the best practices and strategies for mitigating API request overload in Java.
1. Implement Rate Limiting
One effective way to handle API request overload is by implementing rate limiting. Rate limiting allows you to control the number of requests an API can process within a defined time period. By setting limits on the number of requests per second or minute, you can prevent the API from being overwhelmed by a sudden influx of requests. To implement rate limiting in Java, you can utilize libraries such as Guava
or Bucket4j
to easily incorporate rate limiting capabilities into your API endpoints.
// Example of using Guava RateLimiter to limit API requests
RateLimiter rateLimiter = RateLimiter.create(10.0); // Allow 10 requests per second
if (rateLimiter.tryAcquire()) {
// Process the API request
} else {
// Return an error response indicating the rate limit has been exceeded
}
Why It Matters
Implementing rate limiting ensures that your API resources are allocated efficiently, prevents abuse or misuse of the API, and maintains a consistent level of service for all consumers.
2. Use Asynchronous Processing
When dealing with a high volume of API requests, leveraging asynchronous processing can significantly improve the scalability and responsiveness of your API. By handling requests asynchronously, your API can continue to accept new requests while processing existing ones in the background. In Java, you can employ frameworks such as CompletableFuture
or RxJava
to achieve asynchronous processing of API requests, thus preventing request queuing and timeouts during peak loads.
// Example of using CompletableFuture for asynchronous API request processing
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Process the API request asynchronously
});
// Continue processing or return a response while the request is being handled asynchronously
Why It Matters
Asynchronous processing allows your API to handle a larger number of concurrent requests without becoming blocked or experiencing delays, resulting in improved overall performance and user experience.
3. Implement Caching
Introducing caching mechanisms can significantly reduce the load on your API by storing and serving frequently accessed data without the need to repeatedly fetch it from the underlying data source. In Java, you can incorporate caching using libraries like Ehcache
or Caffeine
to cache API responses or computed data, thereby minimizing the processing load on the API servers and reducing response times for repeated requests.
// Example of using Ehcache for API response caching
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("apiResponseCache",
CacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, String.class, ResourcePoolsBuilder.heap(100)))
.build(true);
Cache<Long, String> apiResponseCache = cacheManager.getCache("apiResponseCache", Long.class, String.class);
String cachedResponse = apiResponseCache.get(apiRequestKey);
if (cachedResponse != null) {
// Serve the cached response
} else {
// Process the request and cache the response
apiResponseCache.put(apiRequestKey, apiResponse);
}
Why It Matters
By implementing caching, you can reduce the load on your API servers, improve response times, and enhance the overall scalability of your API infrastructure.
4. Horizontal Scaling with Load Balancing
Horizontal scaling involves adding more server instances to distribute the incoming API requests across multiple machines, thus increasing the overall capacity and throughput of the API. Load balancing mechanisms are essential in distributing incoming requests evenly across these server instances. In Java, you can leverage load balancing solutions provided by cloud platforms like AWS Elastic Load Balancing or use open-source load balancers such as Haproxy
or Nginx
to achieve efficient distribution of API traffic.
// AWS Elastic Load Balancing configuration to distribute API traffic across multiple EC2 instances
// Not actual Java code, but shows the configuration concept
elb.configureBackendInstances(apiInstances);
elb.setLoadBalancingAlgorithm(LoadBalancingAlgorithm.ROUND_ROBIN);
Why It Matters
Horizontal scaling with load balancing ensures that your API infrastructure can handle increased traffic and provides fault tolerance by distributing requests across multiple server instances.
5. Resource Monitoring and Alerting
Proactively monitoring the resource utilization and performance metrics of your API infrastructure is crucial for identifying and addressing potential overload situations. By utilising monitoring tools such as Prometheus
or New Relic
in conjunction with alerting systems like PagerDuty
or Opsgenie
, you can set up proactive alerting based on predefined thresholds for metrics such as CPU usage, memory usage, request latency, and error rates.
// Example of defining a Prometheus alert rule for high API request latency
ALERT HighRequestLatency
IF api_request_duration_seconds_bucket{job="api-server",le="0.5"} > 0.05
FOR 5m
LABELS {severity="critical"}
ANNOTATIONS {
summary = "High API Request Latency",
description = "API request latency is above the threshold for 5 minutes."
}
Why It Matters
Proactive monitoring and alerting enable you to detect and address potential API overload situations before they impact the user experience, ensuring the stability and reliability of your API infrastructure.
A Final Look
Effectively handling API request overload is crucial for maintaining the performance, reliability, and scalability of your Java-based API. By implementing strategies such as rate limiting, asynchronous processing, caching, horizontal scaling with load balancing, and proactive monitoring, you can ensure that your API infrastructure is resilient to high traffic volumes and provides a consistent level of service to its consumers.
Incorporating these best practices not only safeguards your API from overload scenarios but also enhances the overall user experience, resulting in satisfied and engaged users. As the digital landscape continues to evolve, mastering the art of handling API request overload will be essential for Java developers striving to build robust and high-performing API solutions.
Remember, the key to effective API handling lies in proactive planning, constant monitoring, and a willingness to adapt and evolve with the ever-changing demands of the digital ecosystem. By embracing these best practices, you can navigate the challenges of API request overload with confidence and ensure the seamless operation of your Java-based API infrastructure.
Happy coding!