Handling Servlet Exceptions Effectively
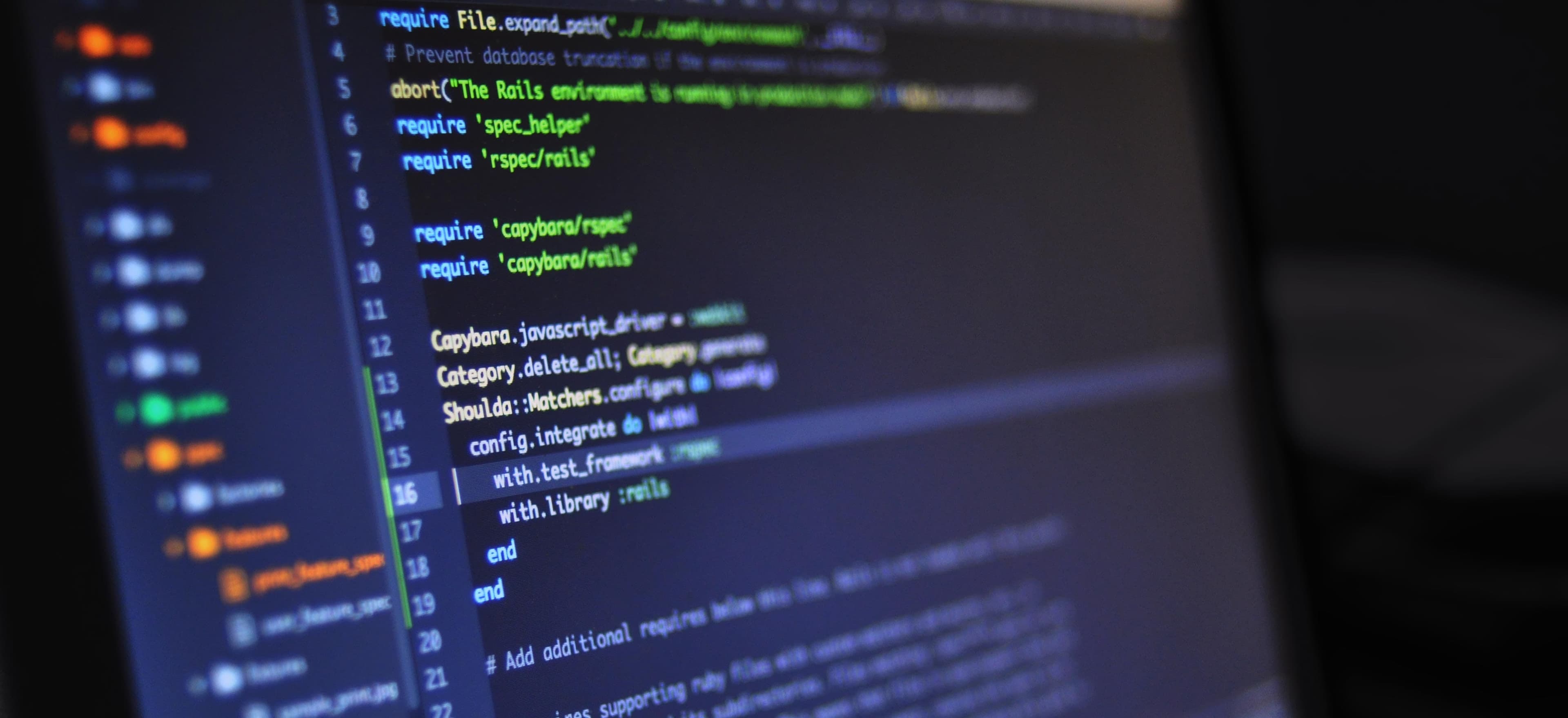
- Published on
Handling Servlet Exceptions Effectively
In any web application, Servlets play a crucial role in processing and responding to client requests. However, like any other piece of software, Servlets are prone to errors and exceptions. These exceptions can occur due to various reasons such as database connectivity issues, resource unavailability, or even due to client-side errors. It's essential for a robust web application to handle these exceptions gracefully and provide meaningful responses to the users. In this article, we will explore the best practices for handling Servlet exceptions effectively in Java web applications.
Understanding Servlet Exceptions
Servlet exceptions can be broadly categorized into two types: checked and unchecked exceptions. Unchecked exceptions are those that extend RuntimeException
or Error
, and are usually caused by programming errors or unexpected conditions. Checked exceptions are those that extend Exception
but not RuntimeException
, and are typically used for recoverable conditions that a program should catch and handle.
Using try-catch Blocks in Servlets
When writing Servlet code, it's essential to handle exceptions using try-catch blocks. This ensures that if an exception occurs during the execution of the Servlet, it can be caught, and appropriate actions can be taken. Here's an example of how try-catch blocks can be used in a Servlet:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
// Servlet logic that may throw an exception
} catch (SomeException e) {
// Handling the exception
log.error("An error occurred: " + e.getMessage());
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "An internal server error occurred");
}
}
In the above code, the doGet
method of the Servlet encapsulates its logic within a try block. If an exception of type SomeException
is thrown during the execution, it's caught in the catch block, and a suitable error response is sent to the client.
Using Exception Handling Frameworks
In complex web applications, handling exceptions at the Servlet level might not be sufficient. This is where exception handling frameworks like Spring MVC come into play. Spring MVC provides robust support for centralized exception handling through the use of @ControllerAdvice
and @ExceptionHandler
annotations. These annotations allow for the creation of global exception handlers that can deal with different types of exceptions across multiple Servlets. Here's an example of how Spring MVC's exception handling works:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(SomeException.class)
public ResponseEntity<String> handleSomeException(SomeException e) {
// Handling the exception
return new ResponseEntity<>("An error occurred: " + e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this example, the @ControllerAdvice
annotation marks the class as a global exception handler, and the @ExceptionHandler
annotation specifies which type of exception it can handle. When an exception of type SomeException
is thrown in any Servlet, it will be intercepted by this global handler, which will then provide a suitable response to the client.
Custom Error Pages
Another effective way to handle Servlet exceptions is by using custom error pages. These error pages can be configured in the web.xml file and will be displayed to the user when a specific type of exception occurs. Here's an example of how custom error pages can be configured in the web.xml file:
<error-page>
<exception-type>java.lang.Exception</exception-type>
<location>/errorpages/genericError.jsp</location>
</error-page>
In this example, when any Servlet throws a generic java.lang.Exception
, the user will be redirected to the /errorpages/genericError.jsp
page, which can provide a user-friendly message about the error.
Logging and Monitoring
Logging is a critical aspect of handling Servlet exceptions effectively. By using logging frameworks like Log4j or SLF4J, you can log detailed information about the exceptions that occur in your Servlets. This not only helps in debugging and identifying the root cause of the exception but also aids in monitoring the health of your application. Additionally, integrating tools like Splunk or ELK Stack for log aggregation and monitoring can provide real-time insights into the exception occurrences in your web application.
Graceful Error Messages
When an exception occurs in a Servlet, it's important to convey a clear and user-friendly error message to the client. Generic error messages such as "Internal Server Error" or "Something went wrong" are not informative and can lead to frustration for the end user. By providing specific and actionable error messages, you can guide the user on what went wrong and what they can do to resolve the issue. This can greatly enhance the user experience and help in building trust in your application.
In Conclusion, Here is What Matters
In this article, we've discussed various techniques for handling Servlet exceptions effectively in Java web applications. From using try-catch blocks for basic exception handling to leveraging advanced frameworks like Spring MVC for centralized exception handling, there are multiple approaches to ensure that your web application responds gracefully to unexpected errors. Additionally, logging, monitoring, and providing meaningful error messages are crucial aspects of effective exception handling. By incorporating these best practices, you can enhance the robustness and reliability of your Java web application, leading to a better user experience and increased user satisfaction.
Remember, effective exception handling is not just about fixing errors, but also about ensuring that your users are informed and supported when things don't go as planned.