Addressing Bad Code in the JDK
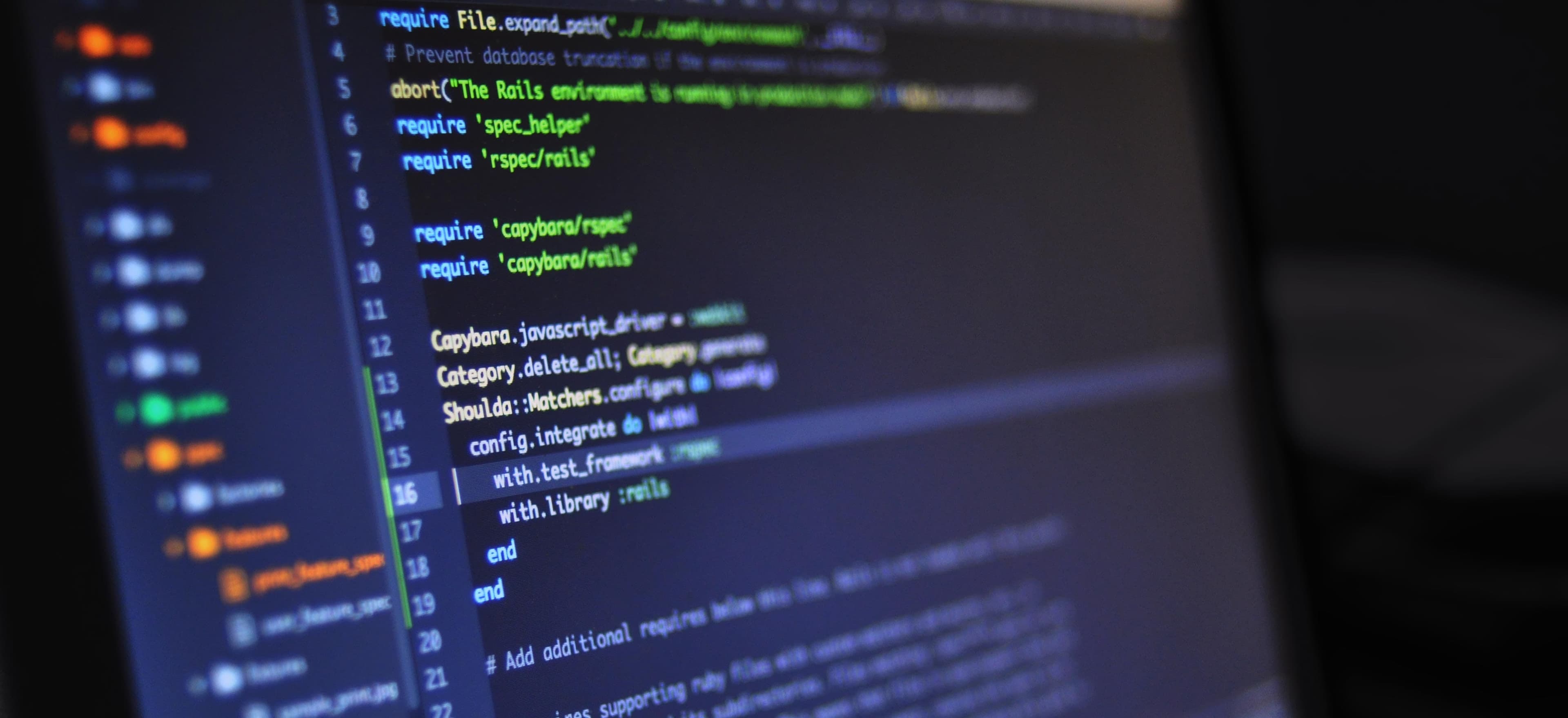
- Published on
Unveiling and Fixing Bad Code in the JDK
As developers, we often rely on the Java Development Kit (JDK) to build robust and efficient applications. However, the JDK, although extensive and powerful, is not immune to having its fair share of less than optimal code. In this article, we'll shine a light on some instances of less-than-ideal coding practices in the JDK and explore how these can be addressed.
The Importance of Addressing Bad Code
Before delving into specific instances, it's crucial to understand why addressing bad code in the JDK is significant. The JDK serves as the foundation for countless Java applications, and any inefficiencies or bugs in its code can have widespread repercussions. By identifying and rectifying these issues, we not only improve the JDK itself but also enhance the overall quality and performance of Java-based software.
Example of Suboptimal Code in the JDK
Let's start by examining a piece of actual code from the JDK that may not be optimal. Consider the following method from the java.util.HashMap
class:
/**
* Returns the value to which the specified key is mapped in this map,
* or {@code null} if the map contains no mapping for the key.
*
* @param key the key whose associated value is to be returned
* @return the value to which the specified key is mapped, or
* {@code null} if this map contains no mapping for the key
*/
public V get(Object key) {
if (key == null)
return getForNullKey();
int hash = hash(key.hashCode());
for (Entry<K,V> e = table[indexFor(hash, table.length)];
e != null;
e = e.next) {
Object k;
if (e.hash == hash && ((k = e.key) == key || key.equals(k)))
return e.value;
}
return null;
}
While this code functions as intended, there is a potential issue with its readability. The use of a single-letter variable name (k
) and a complex conditional statement may hinder comprehension and maintainability.
Refactoring for Readability
To address the readability concern, we can refactor the method as follows:
public V get(Object key) {
if (key == null) {
return getForNullKey();
}
int hash = hash(key.hashCode());
for (Entry<K,V> entry = table[indexFor(hash, table.length)];
entry != null;
entry = entry.next) {
Object entryKey;
if (entry.hash == hash && ((entryKey = entry.key) == key || key.equals(entryKey))) {
return entry.value;
}
}
return null;
}
By using meaningful variable names such as entry
and entryKey
, and adding braces for clarity, we've enhanced the code's readability without altering its functionality. This refactoring facilitates easier maintenance and comprehension for developers who work on the JDK codebase or leverage these classes in their applications.
Handling Null Values in the JDK
Another example of suboptimal code in the JDK can be found in the handling of null values. Consider the following code snippet from the java.util.ArrayList
class:
/**
* Inserts the specified element at the specified position in this list.
*
* @param index index at which the specified element is to be inserted
* @param element element to be inserted
* @throws IndexOutOfBoundsException {@inheritDoc}
*/
public void add(int index, E element) {
rangeCheckForAdd(index);
ensureCapacityInternal(size + 1); // Increments modCount!!
System.arraycopy(elementData, index, elementData, index + 1, size - index);
elementData[index] = element;
size++;
}
In the above code, there is no explicit check or handling for the case where element
is null. This omission can potentially lead to unexpected behavior or null pointer exceptions when null
is passed as the element
argument.
Improved Handling of Null Values
To address this issue, we can modify the add
method as follows:
public void add(int index, E element) {
rangeCheckForAdd(index);
ensureCapacityInternal(size + 1); // Increments modCount!!
if (element == null) {
elementData[size] = null;
} else {
System.arraycopy(elementData, index, elementData, index + 1, size - index);
elementData[index] = element;
}
size++;
}
By adding a check for null
and assigning it directly to elementData[size]
, we prevent potential issues arising from a null element
and maintain the integrity and consistency of the list.
Contribution and Collaboration
Addressing bad code in the JDK is not a task that should be undertaken in isolation. The open-source nature of the JDK allows developers to contribute to its enhancement and maintenance. By participating in initiatives such as OpenJDK, developers can collaborate with the global community to address and rectify suboptimal code, thereby improving the overall quality and performance of the JDK.
The Last Word
In conclusion, identifying and rectifying suboptimal code in the JDK is an essential undertaking for ensuring the robustness and reliability of Java-based applications. By refactoring for readability, handling edge cases such as null values, and actively contributing to the improvement of the JDK, developers can play a vital role in enhancing the core foundations of Java development.
With a collective effort to address bad code, we can elevate the JDK to new levels of excellence, laying the groundwork for even more powerful and resilient Java applications in the future.
Remember, the pursuit of code excellence is an ongoing journey, and every contribution, no matter how small, makes a significant impact.
Let's continue to champion the quality and integrity of the JDK and the Java ecosystem as a whole.