Troubleshooting Common Issues in Spring Integration for EAI
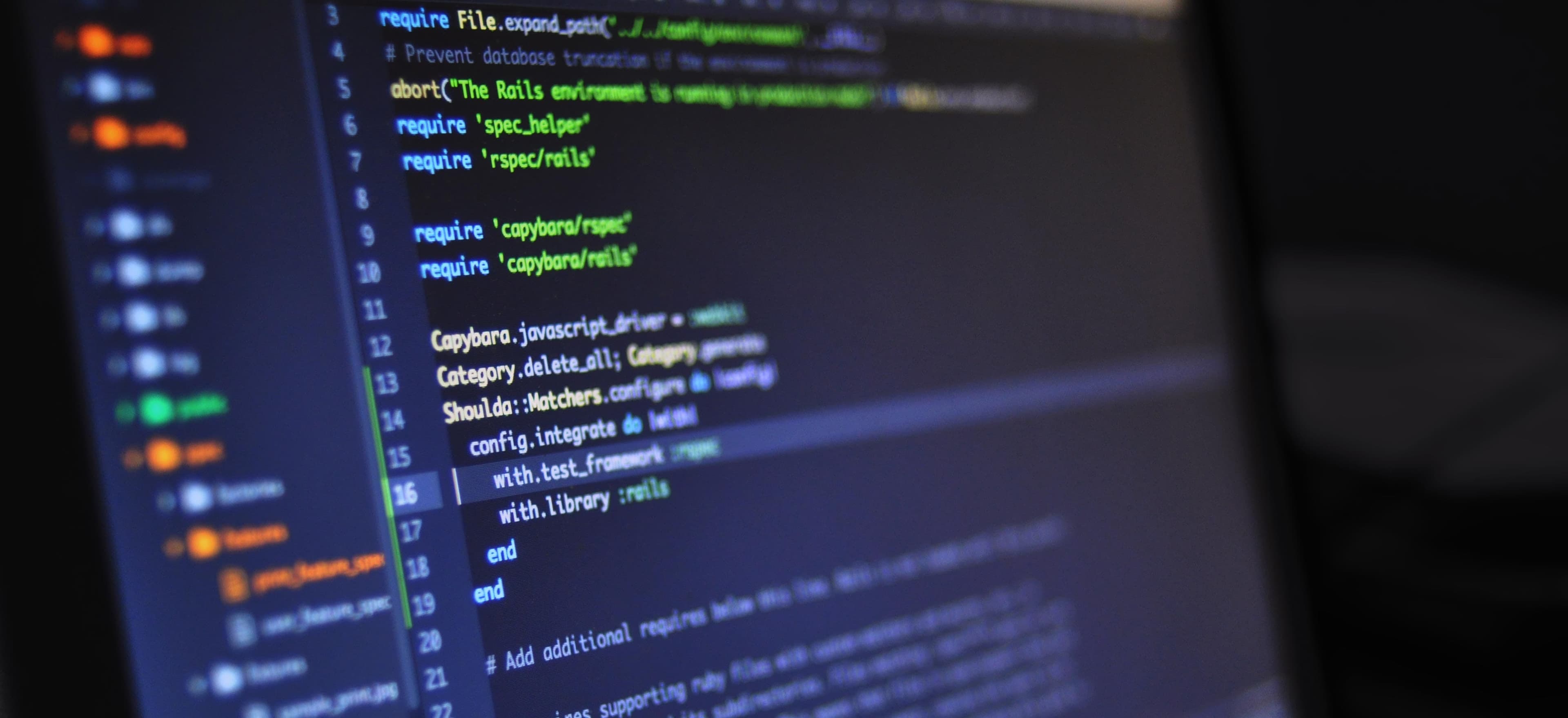
- Published on
Troubleshooting Common Issues in Spring Integration for EAI
When working on Enterprise Application Integration (EAI) using Spring Integration, developers often encounter various issues that can impede the seamless flow of data and processes within their applications. In this post, we will explore some common issues that developers face with Spring Integration and discuss troubleshooting strategies to address them effectively.
1. Message Not Getting Routed to the Correct Channel
One common issue in Spring Integration is when a message is not routed to the correct channel as expected. This can occur due to misconfiguration or misunderstanding of the messaging flow within the application.
@Bean
public IntegrationFlow sampleFlow() {
return IntegrationFlows.from("inputChannel")
.handle((p, h) -> {
// processing logic
return p;
})
.channel("outputChannel")
.get();
}
In the above code snippet, the message is expected to flow from the inputChannel
to the .handle()
method and then to the outputChannel
. If the message does not reach the outputChannel
, check if the message payload is being returned from the .handle()
method. Also, ensure that the outputChannel
is correctly configured.
2. Error Handling and Dead Letter Channel
Error handling is crucial in any integration application. In Spring Integration, the Dead Letter Channel is used to handle messages that cannot be processed successfully after a certain number of retries.
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("inputChannel")
.handle((p, h) -> {
// processing logic that may throw an exception
return p;
}, e -> e.advice(retryAdvice()))
.handle("errorHandler", "handleError")
.get();
}
@Bean
public RequestHandlerRetryAdvice retryAdvice() {
return new RequestHandlerRetryAdvice();
}
In the above code, the retryAdvice()
method configures the retry mechanism for the .handle()
method. If the maximum retry count is reached, the message will be routed to the error handler for further processing.
3. Dealing with Large Messages
Handling large messages efficiently in Spring Integration is essential for maintaining optimal performance. When dealing with large messages, consider using streaming and chunking to process the messages in smaller parts.
@Bean
public IntegrationFlow largeMessageHandlingFlow() {
return IntegrationFlows.from("inputChannel")
.<String, String>transform(String::toUpperCase)
.split(s -> s.delimiters(","))
.handle((p, h) -> {
// process individual message parts
return p;
})
.aggregate()
.handle((p, h) -> {
// aggregate processed parts
return p;
})
.get();
}
In the above code, the .split()
method is used to split the large message into smaller parts, which are then processed individually before being aggregated back together.
Lessons Learned
In this post, we have explored some common issues in Spring Integration for EAI and discussed strategies for troubleshooting them. By understanding the underlying causes of these issues and applying the appropriate troubleshooting techniques, developers can ensure the smooth operation of their integration applications.
For further reading, you can delve into Spring Integration Documentation to gain a comprehensive understanding of the framework's capabilities and best practices.
Remember, debugging and troubleshooting are integral parts of the development process, and mastering these skills will undoubtedly enhance your proficiency as a Spring Integration developer.