Solving Web App Scheduling with Quartz Scheduler
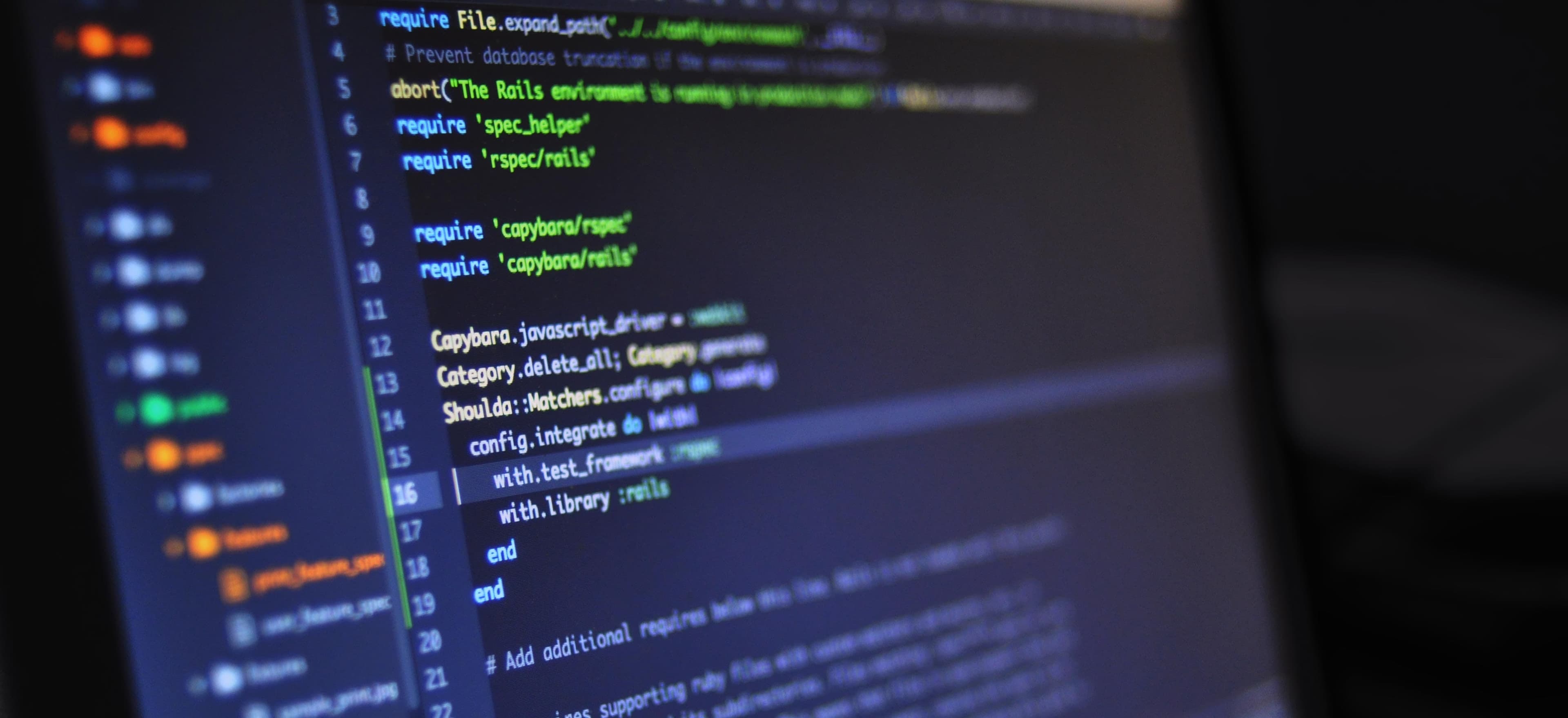
- Published on
Solving Web App Scheduling with Quartz Scheduler
Scheduling tasks within a web application is a crucial feature that allows for automated processes, such as sending out emails, generating reports, and performing routine maintenance. In Java, the Quartz Scheduler is a powerful and versatile library for scheduling jobs.
In this blog post, we will delve into the world of web app scheduling with Quartz Scheduler, covering its basic concepts, usage, and best practices.
What is Quartz Scheduler?
Quartz is an open-source job scheduling library that can be integrated into a wide range of Java applications. It provides powerful features for scheduling and executing jobs with customizable triggers, allowing developers to create complex schedules for recurring tasks.
Getting Started with Quartz Scheduler
To start using Quartz Scheduler in your Java web application, you'll need to add the dependency to your project. If you're using Maven, you can include the following dependency in your pom.xml
:
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz</artifactId>
<version>2.3.2</version>
</dependency>
Once you've added the dependency, you can create a simple Quartz job by implementing the Job
interface and overriding the execute
method:
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
public class MyJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
// Your job logic goes here
}
}
Scheduling a Job
After creating a job, you can schedule it using a Trigger
. Triggers are used to define when a job should be executed. Here's an example of scheduling a job to run every 10 seconds:
import org.quartz.*;
import org.quartz.impl.StdSchedulerFactory;
public class SchedulerExample {
public static void main(String[] args) throws SchedulerException {
SchedulerFactory schedulerFactory = new StdSchedulerFactory();
Scheduler scheduler = schedulerFactory.getScheduler();
scheduler.start();
JobDetail job = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever())
.build();
scheduler.scheduleJob(job, trigger);
}
}
In this example, we create a Scheduler
using StdSchedulerFactory
, define a JobDetail
for our MyJob
class, and create a Trigger
using TriggerBuilder
to schedule the job to run every 10 seconds.
Job Persistence
Quartz Scheduler provides the capability to persist jobs and triggers to a database, allowing for job state preservation and recovery in case of application restarts. This is especially useful for ensuring that scheduled jobs are not lost in the event of a system failure.
To enable job persistence, you can configure Quartz to use a database as a job store. Here's a simple example using H2 database:
Properties props = new Properties();
props.setProperty("org.quartz.jobStore.class", "org.quartz.impl.jdbcjobstore.JobStoreTX");
props.setProperty("org.quartz.jobStore.driverDelegateClass", "org.quartz.impl.jdbcjobstore.StdJDBCDelegate");
props.setProperty("org.quartz.jobStore.dataSource", "myDS");
props.setProperty("org.quartz.dataSource.myDS.driver", "org.h2.Driver");
props.setProperty("org.quartz.dataSource.myDS.URL", "jdbc:h2:~/quartz");
props.setProperty("org.quartz.dataSource.myDS.user", "sa");
props.setProperty("org.quartz.dataSource.myDS.password", "");
props.setProperty("org.quartz.dataSource.myDS.maxConnections", "5");
You can then use the props
object to initialize the SchedulerFactory
:
SchedulerFactory schedulerFactory = new StdSchedulerFactory(props);
By persisting jobs and triggers to a database, you ensure that your scheduled tasks are resilient to application restarts and can recover their state seamlessly.
Error Handling and Monitoring
When working with scheduled jobs in a web application, it's crucial to implement robust error handling and monitoring. Quartz Scheduler provides mechanisms for handling job execution exceptions and monitoring job execution status.
You can use the JobListener
interface to create a listener that responds to various job-related events, such as job execution success and failure. This allows you to implement custom error handling logic and get insights into job execution status in real time.
Furthermore, Quartz provides integration with logging frameworks like Log4j and SLF4J, enabling you to log job execution details and monitor scheduled tasks effectively.
Best Practices
When using Quartz Scheduler for web app scheduling, it's important to adhere to best practices to ensure reliable and efficient job execution:
-
Use Clustering: If your web application runs on multiple nodes, consider setting up Quartz Scheduler in a cluster mode to distribute job execution across the nodes and provide fault tolerance.
-
Handle Long-Running Jobs: For long-running jobs, consider using the
JobListener
interface to implement timeout and recovery mechanisms to prevent job execution from stalling the scheduler. -
Optimize Database Configuration: When using job persistence, optimize your database configuration to handle the load of storing and managing job and trigger data effectively.
By following these best practices, you can maximize the effectiveness of Quartz Scheduler in your web application and ensure smooth and reliable job scheduling.
My Closing Thoughts on the Matter
In conclusion, Quartz Scheduler is a powerful tool for implementing job scheduling in Java web applications. It provides a robust set of features for defining and executing scheduled tasks, as well as mechanisms for job persistence, error handling, and monitoring.
By leveraging Quartz Scheduler, you can automate routine tasks, improve system efficiency, and enhance the overall user experience of your web application.
In our next post, we will explore advanced scheduling techniques and integration with Spring Framework for seamless job management. Stay tuned for more insights into maximizing the potential of Quartz Scheduler in your Java web application!
Give Quartz Scheduler a try in your next web app project, and experience the benefits of automated task scheduling firsthand. Happy scheduling!