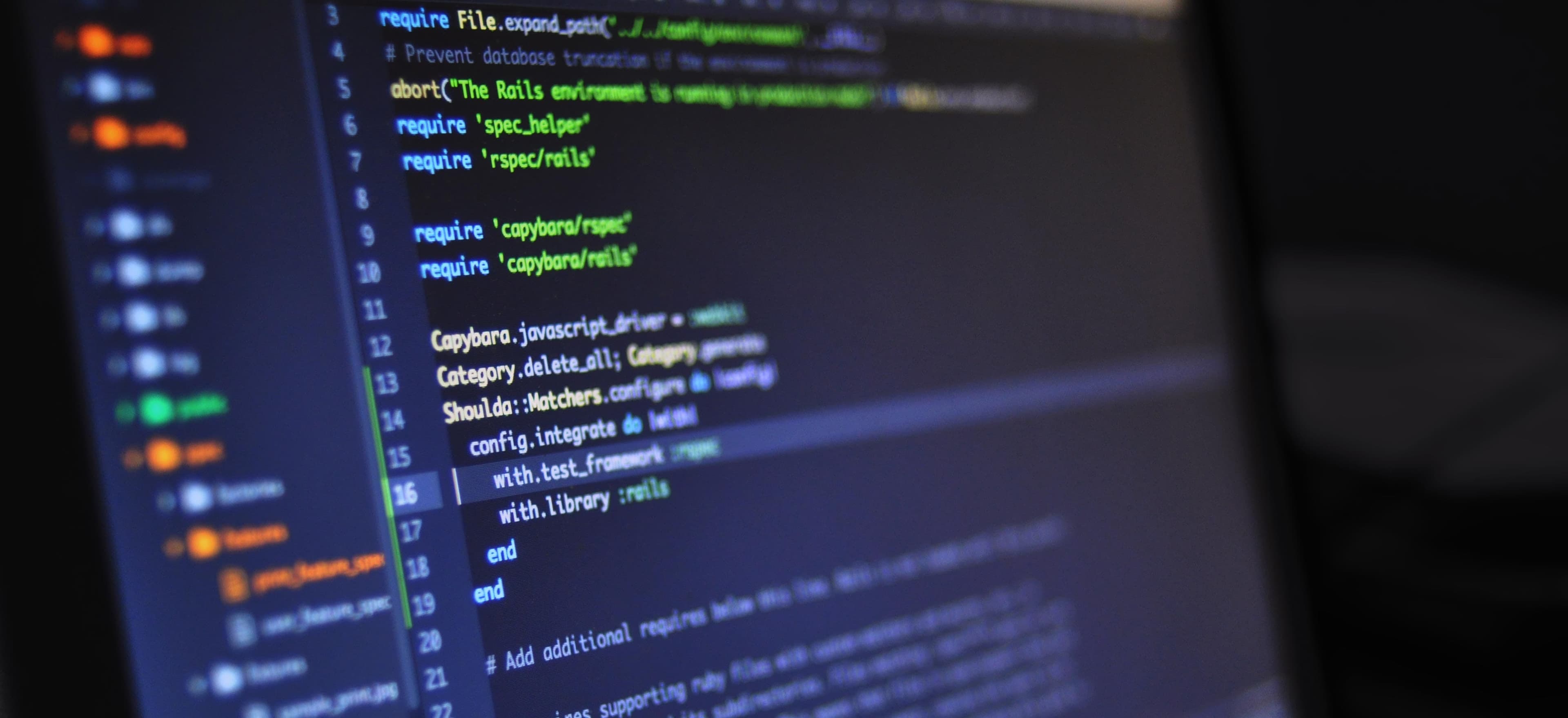
- Published on
Implementing Single Sign-On in a Spring Boot Application
Single Sign-On (SSO) is a crucial component for modern web applications, as it enables users to access multiple applications with a single set of credentials. This not only enhances user experience but also simplifies the access management process for both users and administrators. In this article, we will explore how to implement Single Sign-On in a Spring Boot application using the popular open-source identity and access management solution, Keycloak.
What is Single Sign-On (SSO)?
Single Sign-On (SSO) is a mechanism that allows users to access multiple applications with a single set of credentials. Once the user is authenticated by one application, they can seamlessly access other applications without needing to re-enter their credentials. This improves user experience and reduces the burden of managing multiple sets of credentials for different applications.
Benefits of Single Sign-On (SSO)
- User Convenience: Users don't have to remember multiple sets of credentials, leading to a smoother authentication process.
- Enhanced Security: Centralized authentication and authorization process ensures consistent security policies across all applications.
- Administrator Efficiency: Simplifies user management and reduces administrative overhead in handling credentials for multiple applications.
Implementing Single Sign-On with Keycloak and Spring Boot
Keycloak is an open-source Identity and Access Management solution aimed at modern applications and services. It provides features such as Single Sign-On, Identity Brokering, Social Login, User Federation, and centralized access control.
Setting Up Keycloak
To begin, you need to set up a Keycloak server. You can download the standalone Keycloak server from the official website or use the Docker image for quick setup. After setting up Keycloak, you need to create a new realm, client, and users for SSO integration.
Integrating Keycloak with Spring Boot
Step 1: Adding Keycloak Dependencies
Integrate Keycloak with your Spring Boot application by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>org.keycloak</groupId>
<artifactId>keycloak-spring-boot-starter</artifactId>
</dependency>
Step 2: Configuring Keycloak in application.properties
Configure your Spring Boot application to use Keycloak by adding the following properties in the application.properties
file:
keycloak.realm=<your-realm>
keycloak.auth-server-url=<keycloak-url>
keycloak.ssl-required=external
keycloak.resource=<your-client-id>
keycloak.credentials.secret=<client-secret>
keycloak.use-resource-role-mappings=true
Replace <your-realm>
, <keycloak-url>
, <your-client-id>
, and <client-secret>
with the appropriate values for your Keycloak realm and client.
Step 3: Securing Endpoints
Secure your endpoints by adding Keycloak configuration to your Spring Security configuration class:
@Configuration
@EnableWebSecurity
@ComponentScan(basePackageClasses = KeycloakSecurityComponents.class)
public class SecurityConfig extends KeycloakWebSecurityConfigurerAdapter {
@Autowired
public void configureGlobal(
AuthenticationManagerBuilder auth)
throws Exception {
KeycloakAuthenticationProvider keycloakAuthenticationProvider
= keycloakAuthenticationProvider();
auth.authenticationProvider(keycloakAuthenticationProvider);
}
@Bean
@Override
protected SessionAuthenticationStrategy sessionAuthenticationStrategy() {
return new RegisterSessionAuthenticationStrategy(
new SessionRegistryImpl());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
http
.authorizeRequests()
.antMatchers("/secured/*")
.hasRole("user")
.anyRequest()
.permitAll();
}
}
In this configuration, the /secured/*
endpoints are secured and require the user to have the user
role to access them.
Final Thoughts
Implementing Single Sign-On provides numerous benefits for both users and application administrators. Keycloak's seamless integration with Spring Boot makes it easier to incorporate SSO into your applications. By following the steps outlined in this article, you can enhance the security and usability of your applications through centralized authentication and authorization processes. Embracing SSO not only streamlines user experience but also helps in enforcing consistent security policies across your applications.
By leveraging Keycloak's powerful features and integrating it with Spring Boot, you can easily develop applications with robust authentication and authorization mechanisms.
In conclusion, the implementation of Single Sign-On in a Spring Boot application using Keycloak is a step towards creating secure, user-friendly, and efficient web applications.
Start implementing Single Sign-On in your applications and elevate the user experience while reinforcing security!