Implementing Container Object Pattern for Cleaner Tests
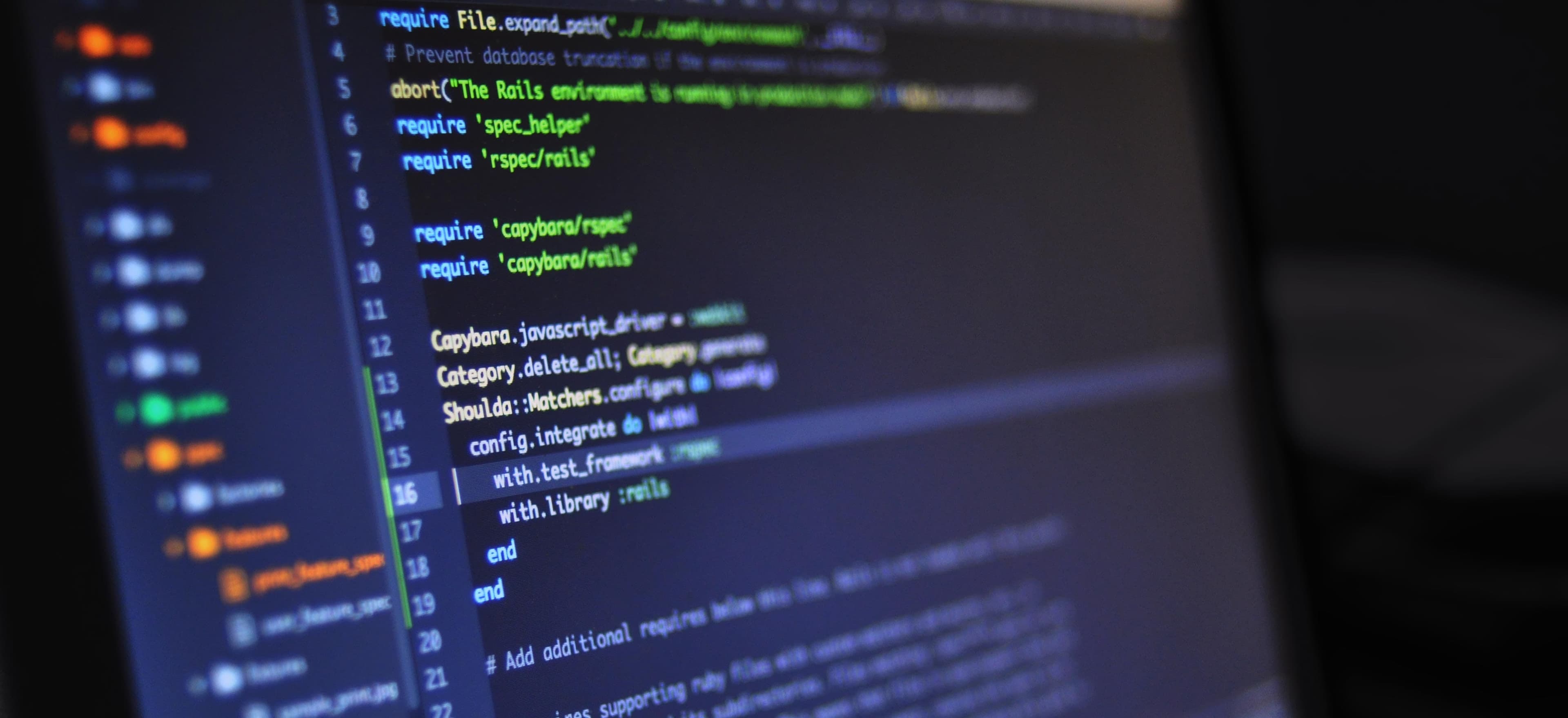
- Published on
Implementing Container Object Pattern for Cleaner Tests
Writing clean and maintainable tests is crucial for any software project. One way to achieve this is by using the Container Object pattern. In this article, we'll explore how to implement the Container Object pattern in Java to improve the readability and maintainability of your test code.
What is the Container Object Pattern?
The Container Object pattern, also known as the Test Data Builder pattern, is a design pattern used to create and manage test data in a more structured and readable way. It involves encapsulating the test data setup and creation logic within dedicated container classes, making the tests more focused and easier to maintain.
The Problem: Messy Test Setup
Consider a scenario where you have multiple test methods that require the same set of test data. Without the Container Object pattern, you might find yourself repeating the same setup code in each test method, leading to code duplication and increased maintenance overhead. Additionally, modifying the test data structure would require changes in multiple places, making the code error-prone.
The Solution: Container Object Pattern
By applying the Container Object pattern, we can encapsulate the test data setup within dedicated container classes. These container classes act as builders for creating the required test data, allowing us to centralize the data setup logic and eliminate redundancy.
Let's dive into a practical example to demonstrate how to implement the Container Object pattern for cleaner tests in Java.
Step 1: Create the Container Object Class
public class UserDataContainer {
private String username;
private String email;
private String password;
public UserDataContainer withUsername(String username) {
this.username = username;
return this;
}
public UserDataContainer withEmail(String email) {
this.email = email;
return this;
}
public UserDataContainer withPassword(String password) {
this.password = password;
return this;
}
public UserData build() {
return new UserData(username, email, password);
}
}
In this example, we've created a UserDataContainer
class to encapsulate the setup logic for creating user data. The class provides fluent builder methods (withUsername
, withEmail
, withPassword
) for setting the attributes of the user data and a build
method to instantiate the UserData
object.
Step 2: Create the Test Using Container Object
public class UserTest {
@Test
public void testUserRegistration() {
UserData userData = new UserDataContainer()
.withUsername("john_doe")
.withEmail("john@example.com")
.withPassword("P@ssw0rd")
.build();
// Perform test using the userData
}
}
In the test method testUserRegistration
, we use the UserDataContainer
to create the required test data in a fluent and readable manner. This approach centralizes the test data setup and improves the readability of the test code.
Benefits of Using Container Object Pattern
- Readability: The usage of fluent builder methods in the Container Object enhances the readability of the test setup code.
- Maintainability: Centralizing the test data setup logic within dedicated container classes makes it easier to modify and maintain the test data structure.
- Reduced Redundancy: Eliminates code duplication by encapsulating the setup logic, reducing maintenance overhead.
The Bottom Line
Implementing the Container Object pattern in your test code can significantly improve its readability, maintainability, and overall quality. By encapsulating the test data setup logic within dedicated container classes, you can create cleaner and more expressive tests.
In conclusion, the Container Object pattern is a valuable tool for writing cleaner, more maintainable tests in Java. By centralizing test data setup logic within container classes, you can improve the readability and reduce redundancy in your test code.
By utilizing the Container Object pattern, you can enhance the quality and maintainability of your test suite, ultimately leading to a more robust software development process.
Now that you have a better understanding of the Container Object pattern in Java, consider incorporating it into your testing practices to elevate the quality of your test code.
Remember, clean tests contribute to the overall success of your software project by providing confidence in the system's behavior and facilitating future development and refactoring efforts.
So, go ahead, start implementing the Container Object pattern, and witness the improvement in your test code's clarity and maintainability. Happy testing!