The Challenge of On-the-Spot Hybrid Cloud Monitoring
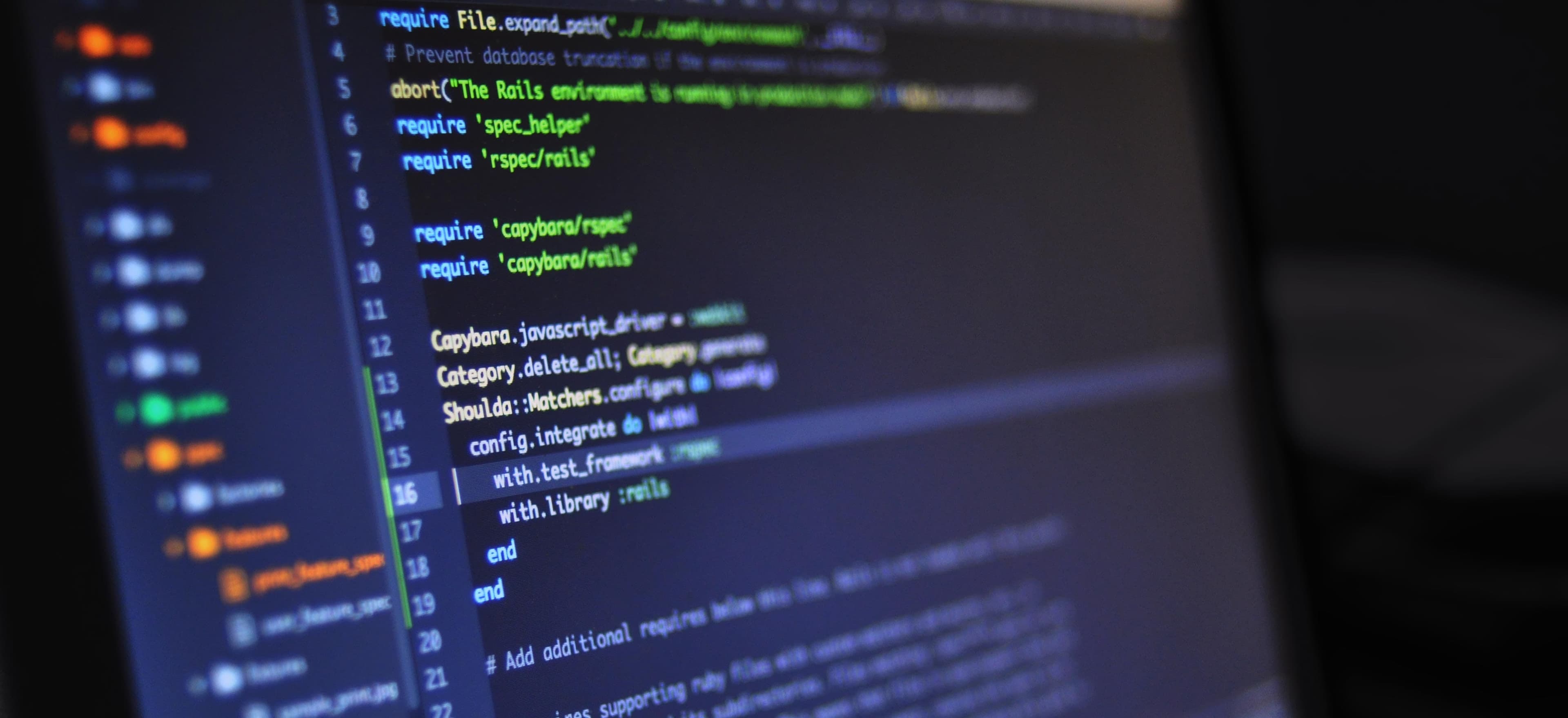
- Published on
The Challenge of On-the-Spot Hybrid Cloud Monitoring
In today's dynamic IT landscape, hybrid cloud adoption is rapidly increasing. This approach offers businesses the flexibility to run workloads across on-premises infrastructure, private cloud, and public cloud environments. While hybrid cloud brings numerous benefits, it also introduces complex monitoring challenges. Organizations need to ensure that their applications and services are performing optimally across these hybrid environments.
The Complexity of Hybrid Cloud Monitoring
Monitoring a hybrid cloud environment requires visibility into multiple layers of the infrastructure, including the on-premises data center, private cloud resources, and various public cloud providers such as AWS, Azure, and Google Cloud Platform. Each environment has its own set of metrics, logs, and performance data that need to be collected, correlated, and analyzed for effective monitoring.
The Need for a Unified Monitoring Solution
To effectively monitor a hybrid cloud environment, organizations need a unified monitoring solution that can seamlessly collect and aggregate data from diverse sources. This solution should provide a holistic view of the entire infrastructure, enabling IT teams to detect and troubleshoot issues proactively, regardless of where they occur within the hybrid environment.
Leveraging Java for Monitoring Hybrid Cloud Environments
Java, with its robust ecosystem of libraries, frameworks, and tools, provides a solid foundation for building monitoring solutions for hybrid cloud environments. Let's explore how Java can be leveraged to address the challenges of on-the-spot hybrid cloud monitoring.
Using Java for Data Collection
Java's versatility makes it well-suited for collecting data from diverse sources within a hybrid cloud environment. Whether it's extracting performance metrics from on-premises servers, retrieving logs from private cloud instances, or accessing cloud provider APIs for resource utilization data, Java's rich set of libraries and APIs simplifies the process of data collection.
// Example of using Java to collect performance metrics from on-premises servers
public class PerformanceMonitor {
public Map<String, Double> collectPerformanceMetrics(List<Server> servers) {
Map<String, Double> metrics = new HashMap<>();
for (Server server : servers) {
// Use Java libraries to collect CPU, memory, and disk usage metrics
metrics.put(server.getName() + "_CPU", server.getCpuUsage());
metrics.put(server.getName() + "_Memory", server.getMemoryUsage());
metrics.put(server.getName() + "_Disk", server.getDiskUsage());
}
return metrics;
}
}
In this example, Java's ability to interact with system resources and external APIs allows for seamless collection of performance metrics from on-premises servers, an essential aspect of hybrid cloud monitoring.
Building Scalable Data Processing Pipelines with Java
Hybrid cloud environments generate a vast amount of data that needs to be processed and analyzed in real-time. Java's support for multi-threading, concurrency, and parallel processing makes it an ideal choice for building scalable data processing pipelines to handle the influx of monitoring data.
// Example of using Java to build a scalable data processing pipeline
public class DataPipeline {
private ExecutorService executorService;
public DataPipeline(int concurrentThreads) {
this.executorService = Executors.newFixedThreadPool(concurrentThreads);
}
public void processData(List<MonitoringData> data) {
for (MonitoringData datum : data) {
executorService.submit(() -> {
// Perform data processing and analysis using Java's concurrent processing capabilities
processAndAnalyze(datum);
});
}
}
}
In this example, Java's ExecutorService
and concurrent processing capabilities enable the creation of a scalable data processing pipeline, ensuring efficient analysis of monitoring data from disparate sources within the hybrid cloud environment.
Integrating with Cloud Provider APIs using Java SDKs
Cloud providers offer comprehensive APIs for accessing and managing resources within their environments. Java SDKs provided by major cloud platforms such as AWS SDK for Java, Azure SDK for Java, and Google Cloud Client Libraries facilitate seamless integration with these APIs, allowing for the retrieval of valuable performance and usage data from public cloud resources.
// Example of using AWS SDK for Java to retrieve performance data from Amazon EC2 instances
public class AwsCloudMonitor {
private AmazonEC2 ec2Client;
public AwsCloudMonitor() {
this.ec2Client = AmazonEC2Client.builder().build();
}
public Map<String, Double> retrievePerformanceMetrics(List<String> instanceIds) {
Map<String, Double> metrics = new HashMap<>();
DescribeInstancesRequest request = new DescribeInstancesRequest().withInstanceIds(instanceIds);
DescribeInstancesResult result = ec2Client.describeInstances(request);
// Extract CPU, memory, and network usage metrics from EC2 instances using AWS SDK for Java
// Populate 'metrics' map with the retrieved data
return metrics;
}
}
In this snippet, Java's integration with AWS SDK for Java enables the retrieval of performance metrics from Amazon EC2 instances, showcasing the capability to seamlessly interact with public cloud APIs within the context of hybrid cloud monitoring.
A Final Look
Monitoring hybrid cloud environments is a complex undertaking, but leveraging Java's capabilities for data collection, scalable data processing, and integration with cloud provider APIs can significantly simplify the monitoring process. By embracing Java as the foundation for building monitoring solutions, organizations can gain comprehensive visibility into their hybrid cloud infrastructure, enabling proactive identification and resolution of performance and availability issues.
Embracing Java as the foundation for building monitoring solutions can significantly simplify the monitoring process for hybrid cloud environments. By leveraging Java's capabilities for data collection, scalable data processing, and integration with cloud provider APIs, organizations can gain comprehensive visibility into their hybrid cloud infrastructure, enabling proactive identification and resolution of performance and availability issues.