Efficient Big Integer Square Root in Java
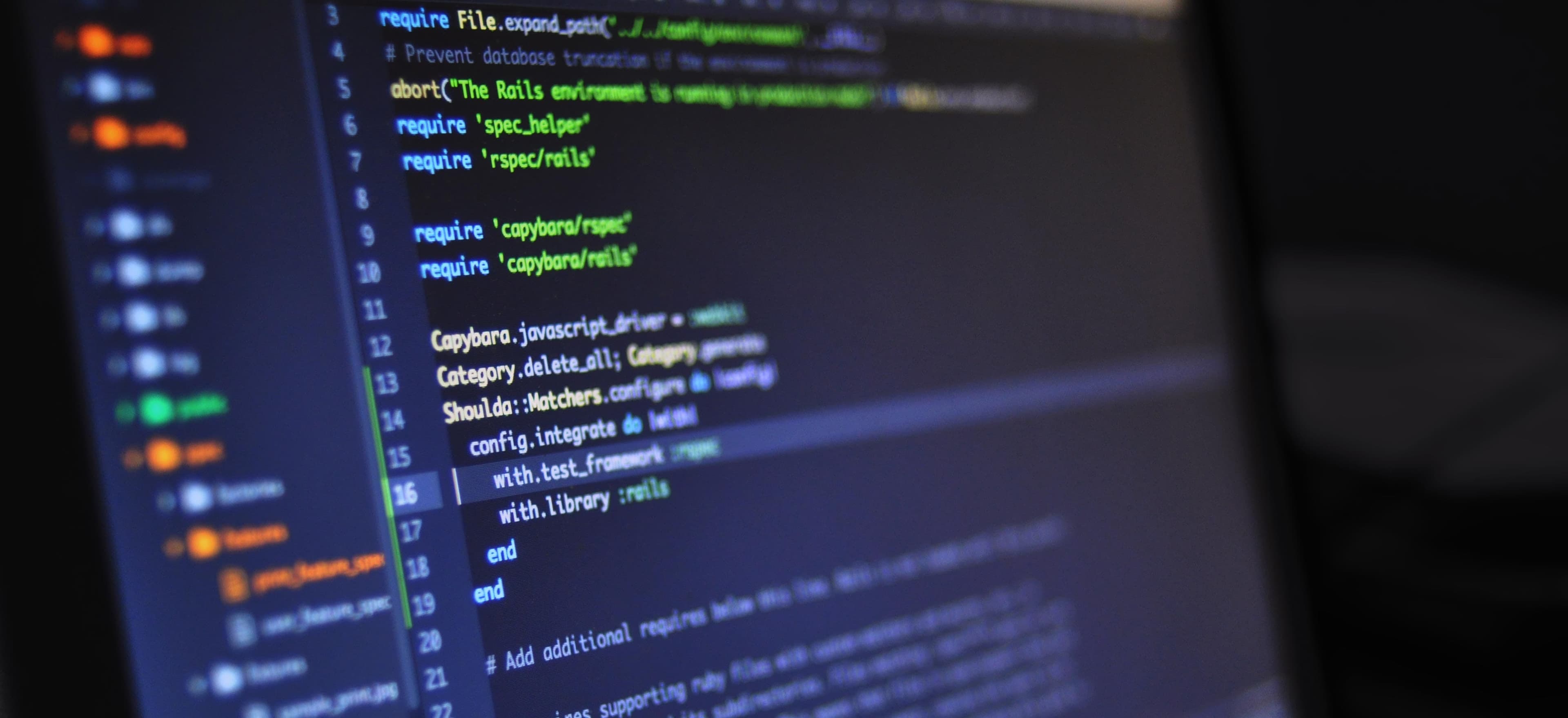
- Published on
Efficient Big Integer Square Root in Java
When working with large numbers in Java, specifically when dealing with big integers, finding the square root efficiently becomes a crucial task. In this blog post, we'll explore an efficient approach to calculating the square root of a big integer in Java.
Understanding the Problem
Calculating the square root of a big integer poses a challenge due to precision requirements and the potential for overflow. Traditional methods using Math.sqrt()
or BigDecimal
may not be suitable for big integers, as they are limited by the data type and are not optimized for large calculations.
Efficient Solution Using Binary Search
One efficient approach to calculate the square root of a big integer is by using binary search. This method leverages the fact that the square root of a number lies between 1 and the number itself. We can use binary search to narrow down the range and find the square root with precision.
Implementation
Let's take a look at a Java implementation of the efficient square root calculation using binary search:
import java.math.BigInteger;
public class BigIntegerSquareRoot {
public static BigInteger sqrt(BigInteger n) {
BigInteger start = BigInteger.ZERO;
BigInteger end = n;
while (start.compareTo(end) <= 0) {
BigInteger mid = start.add(end).divide(BigInteger.TWO);
if (mid.multiply(mid).compareTo(n) == 0) {
return mid;
} else if (mid.multiply(mid).compareTo(n) < 0) {
start = mid.add(BigInteger.ONE);
} else {
end = mid.subtract(BigInteger.ONE);
}
}
return end; // or start - both are the same at this point
}
public static void main(String[] args) {
BigInteger number = new BigInteger("10000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000");
System.out.println(sqrt(number));
}
}
In the sqrt
method, we use a binary search approach to find the square root of a given BigInteger
n. We initialize start
to 0 and end
to n. Then, we iterate through a while loop, adjusting the start
and end
based on the comparison of the midpoint mid
squared with n.
This approach ensures an efficient calculation of the square root without risking overflow issues commonly encountered when handling big integers.
Final Considerations
Calculating the square root of a big integer in Java requires careful consideration of efficiency and precision. By employing binary search with big integers, we can achieve an efficient and accurate square root calculation. This method is crucial for scenarios where traditional square root calculation methods fall short due to limitations in handling large numbers.
In summary, the binary search approach provides a robust solution for computing the square root of big integers in Java, ensuring optimal performance and precision.
For further exploration, you may find the BigInteger documentation and Java BigInteger class helpful.
Try implementing the provided solution in your Java projects dealing with big integers and experience the efficiency firsthand!