Maximizing Efficiency: Creating Agile Feature Teams
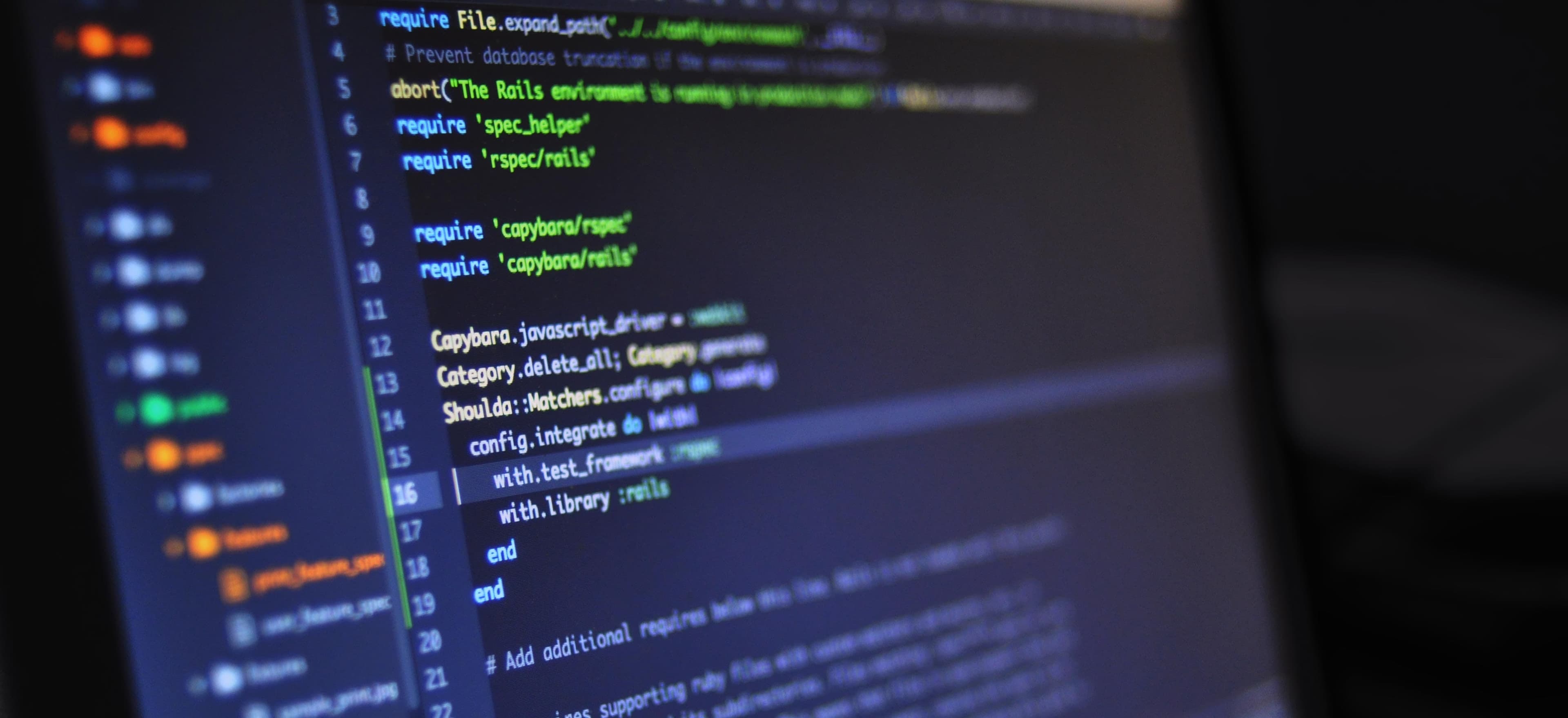
- Published on
Maximizing Efficiency: Creating Agile Feature Teams
In the fast-paced world of software development, efficiency is key. Agile methodologies have gained traction for their ability to adapt to changing requirements and deliver high-quality products. One crucial aspect of Agile development is the creation of feature teams. Feature teams are cross-functional teams that are responsible for delivering end-to-end customer value. In this article, we will explore how Java can be leveraged to create agile feature teams that maximize efficiency.
Embracing Cross-Functionality with Java
Java is a versatile programming language that can be applied to a wide array of tasks, making it an excellent choice for building cross-functional feature teams. By utilizing Java, teams can tap into the language's robustness, scalability, and performance to deliver a diverse set of features.
Leveraging Java's Ecosystem
Java boasts a rich ecosystem with a plethora of libraries and frameworks that can expedite the development process. Whether it's Spring Boot for building microservices, Hibernate for database interactions, or JUnit for testing, Java's ecosystem provides a comprehensive toolkit for feature teams to work seamlessly across different domains.
// Example of utilizing Spring Boot for building a microservice
@RestController
public class FeatureController {
@Autowired
private FeatureService featureService;
@GetMapping("/feature/{id}")
public Feature getFeature(@PathVariable Long id) {
return featureService.getFeature(id);
}
// Additional endpoints and business logic
}
In the above code snippet, we see how Spring Boot's annotations allow for quick setup of a RESTful microservice, enabling feature teams to rapidly prototype and iterate on new features.
Facilitating Continuous Integration and Delivery (CI/CD)
Java's compatibility with CI/CD pipelines further enhances the efficiency of feature teams. Tools like Jenkins, GitLab CI, or CircleCI seamlessly integrate with Java projects, enabling automated builds, testing, and deployments. This streamlines the development workflow, allowing feature teams to focus on delivering value without being bogged down by manual processes.
// Example of a Jenkinsfile for a Java project
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'sh deploy.sh'
}
}
}
}
The Jenkinsfile above showcases how a Java project can be seamlessly integrated into a CI/CD pipeline, automating the build, test, and deployment processes.
Embracing Agile Principles
Agile development relies on principles that empower feature teams to adapt to change and deliver value iteratively. Java, with its flexibility and robustness, aligns seamlessly with these principles, enabling feature teams to embody agility in their development process.
Encouraging Collaboration and Communication
Java's object-oriented nature promotes clean and modular code, making it easier for feature teams to collaborate and communicate effectively. By adhering to coding best practices and design patterns, teams can ensure that their code is easily readable and maintainable, fostering a collaborative environment.
// Example of utilizing design patterns in Java
public interface Feature {
void execute();
}
public class ConcreteFeature implements Feature {
@Override
public void execute() {
// Business logic for the feature
}
}
The above code demonstrates how the use of the Strategy pattern in Java can facilitate a clear and modular structure, allowing feature teams to work on different features independently.
Iterative Development and Continuous Improvement
Java's flexibility and support for test-driven development (TDD) enable feature teams to embrace iterative development. By writing tests before implementation, teams can iteratively build and improve features, ensuring that the codebase remains robust and adaptable to changing requirements.
// Example of test-driven development in Java with JUnit
public class FeatureServiceTest {
@Test
public void testGetFeature() {
// Test logic to assert the behavior of the feature service
}
// Additional test cases for feature service
}
The code snippet above showcases how JUnit can be utilized for writing test cases to drive the development of features, promoting continuous improvement within feature teams.
Embracing Continuous Learning and Adaptation
Agile feature teams thrive on continuous learning and adaptation, and Java's vibrant community and extensive resources cater to this ethos. Feature teams can leverage a myriad of online resources, such as community forums, blogs, and documentation, to stay abreast of best practices and emerging trends in Java development.
Leveraging Online Resources
Online resources such as JavaWorld and Baeldung offer a wealth of articles, tutorials, and insights into Java development best practices. Feature teams can harness these resources to deepen their understanding of Java and stay updated with the latest advancements in the language.
Embracing Community Involvement
Engaging with the Java community through platforms like GitHub, Stack Overflow, and Reddit allows feature teams to seek help, share knowledge, and contribute to open-source projects. By actively participating in the community, teams can foster a culture of continuous learning and collaboration, further enhancing their proficiency in Java development.
My Closing Thoughts on the Matter
Creating agile feature teams that maximize efficiency is pivotal for delivering value in today's dynamic software development landscape. Java, with its versatility, robust ecosystem, and alignment with agile principles, serves as an instrumental tool for realizing this goal. By embracing cross-functionality, agile principles, and continuous learning, feature teams can harness Java's potential to drive efficient and adaptable development processes.
Incorporating Java into agile feature teams is not just about writing code—it's about fostering a mindset that values collaboration, adaptability, and continuous improvement. With the right approach, Java can empower feature teams to navigate the complexities of modern software development with confidence and efficacy.