Overcoming Common JUnit Testing Pitfalls
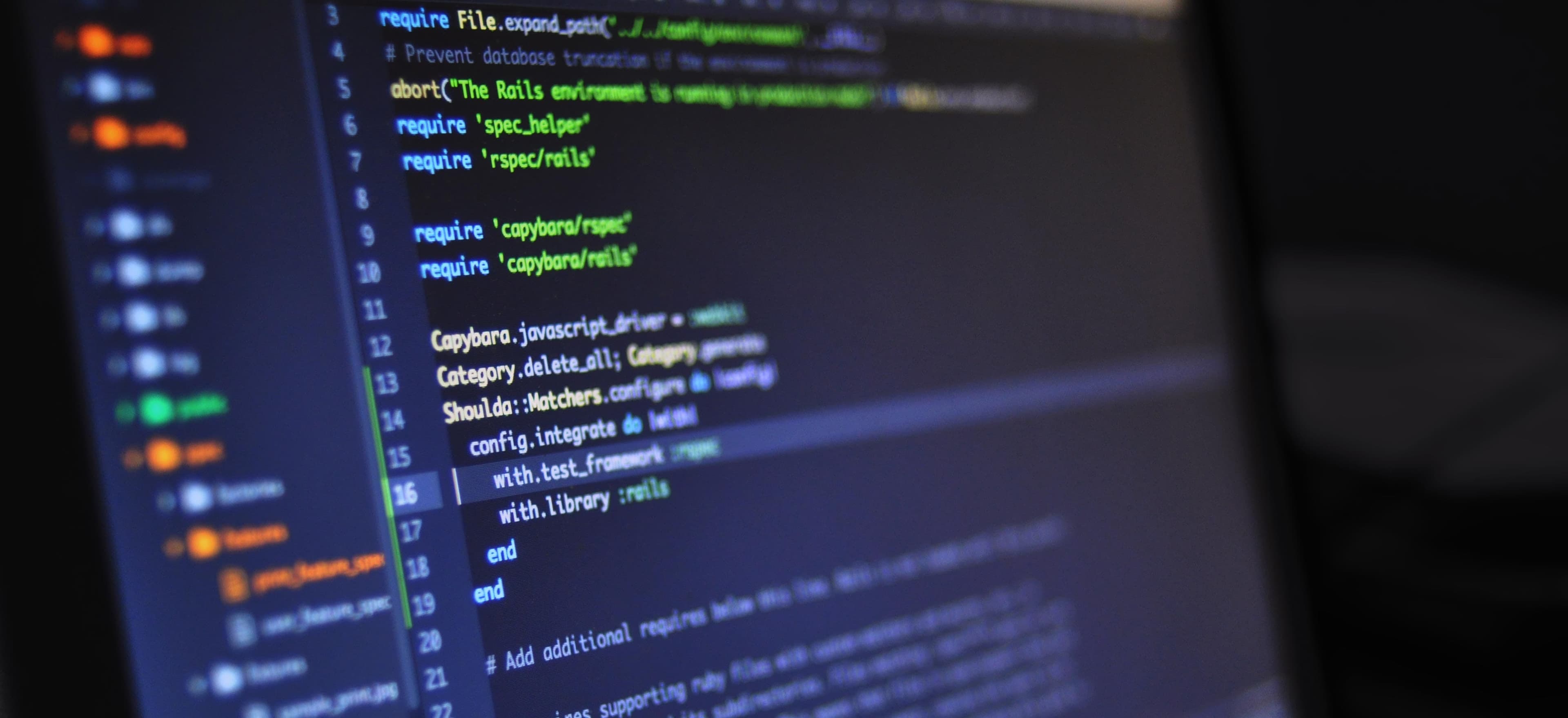
- Published on
Overcoming Common JUnit Testing Pitfalls
When it comes to Java development, writing robust and reliable code is a priority. One of the cornerstones of achieving this is writing effective unit tests. JUnit is a popular and powerful framework for writing and running unit tests in Java. However, even experienced developers can stumble into common pitfalls when using JUnit. In this article, we will explore some of these pitfalls and how to overcome them to write better, more effective JUnit tests.
Pitfall 1: Inconsistent Test Setup
A common mistake in JUnit testing is inconsistent test setup. This occurs when the setup code for tests is not uniform across test methods, leading to potential inconsistencies and unreliable tests.
public class UserServiceTest {
private UserService userService;
@Before
public void setup() {
userService = new UserService();
}
@Test
public void testUserCreation() {
// Test user creation
}
@Test
public void testUserUpdate() {
// Test user update
}
}
In the above example, the setup()
method using the @Before
annotation ensures that the userService
instance is initialized before each test method is executed. This guarantees a consistent test environment and prevents unexpected behaviors due to inconsistent setups.
Pitfall 2: Incorrect Assertions
Another common pitfall is using incorrect assertions, which can lead to misleading test results.
@Test
public void testUserCreation() {
User user = new User();
userService.createUser(user);
assertNotNull(user.getId());
}
In the above test, assertNotNull(user.getId())
is used to verify that the user ID is not null after creating a user. However, a better assertion would be to check if the user was actually created in the system rather than solely relying on the presence of an ID.
@Test
public void testUserCreation() {
User user = new User();
userService.createUser(user);
assertTrue(userService.getAllUsers().contains(user));
}
Pitfall 3: Incomplete Test Coverage
Incomplete test coverage is a significant pitfall that can undermine the effectiveness of unit tests. It occurs when not all possible code paths are tested, leaving gaps in test coverage.
public class MathUtilsTest {
@Test
public void testDivide() {
assertEquals(2, MathUtils.divide(4, 2));
// Missing test for division by zero
}
}
In the above example, only one code path of the divide
method is being tested. To ensure complete coverage, it is essential to also test edge cases, such as division by zero.
@Test
public void testDivideByZero() {
assertThrows(ArithmeticException.class, () -> {
MathUtils.divide(4, 0);
});
}
Pitfall 4: Unnecessary Test Dependencies
Having unnecessary dependencies in tests can make them brittle and difficult to maintain. It's essential to isolate the code under test and avoid unnecessary dependencies.
public class OrderServiceTest {
@Test
public void testOrderTotal() {
Product product = new Product("Test Product", 50.0);
Order order = new Order();
order.addProduct(product);
// Testing order total with unnecessary product creation
}
}
In the above example, creating a Product
instance directly in the test introduces an unnecessary dependency. Instead, consider using mock objects or stubs to isolate the behavior under test.
Pitfall 5: Ignoring Test Performance
Testing performance can often be overlooked, leading to slow and inefficient tests, especially when dealing with large codebases.
public class PerformanceTest {
@Test
public void testPerformance() {
// Test involving a large dataset
}
}
In the above example, the test testPerformance
might involve a large dataset, leading to slow test execution and hindering the overall test suite's performance. It's essential to consider and address performance implications in tests.
In summary, JUnit testing pitfalls can be overcome by maintaining consistent test setups, using correct assertions, ensuring complete test coverage, minimizing unnecessary dependencies, and considering test performance. By addressing these common pitfalls, developers can write more effective and reliable JUnit tests, contributing to the overall quality of their Java codebases.
It's crucial to continually improve your understanding of writing effective unit tests, and JUnit is an essential tool in the Java developer's arsenal. For further insights, the JUnit User Guide provides comprehensive documentation on JUnit, covering features, best practices, and advanced usage.
In conclusion, mastering JUnit testing can significantly enhance the stability and maintainability of Java applications. By recognizing and overcoming common pitfalls, developers can strengthen their testing practices and build more robust software.