Troubleshooting CDI Bean Injection in JAX-RS Sub-Resources
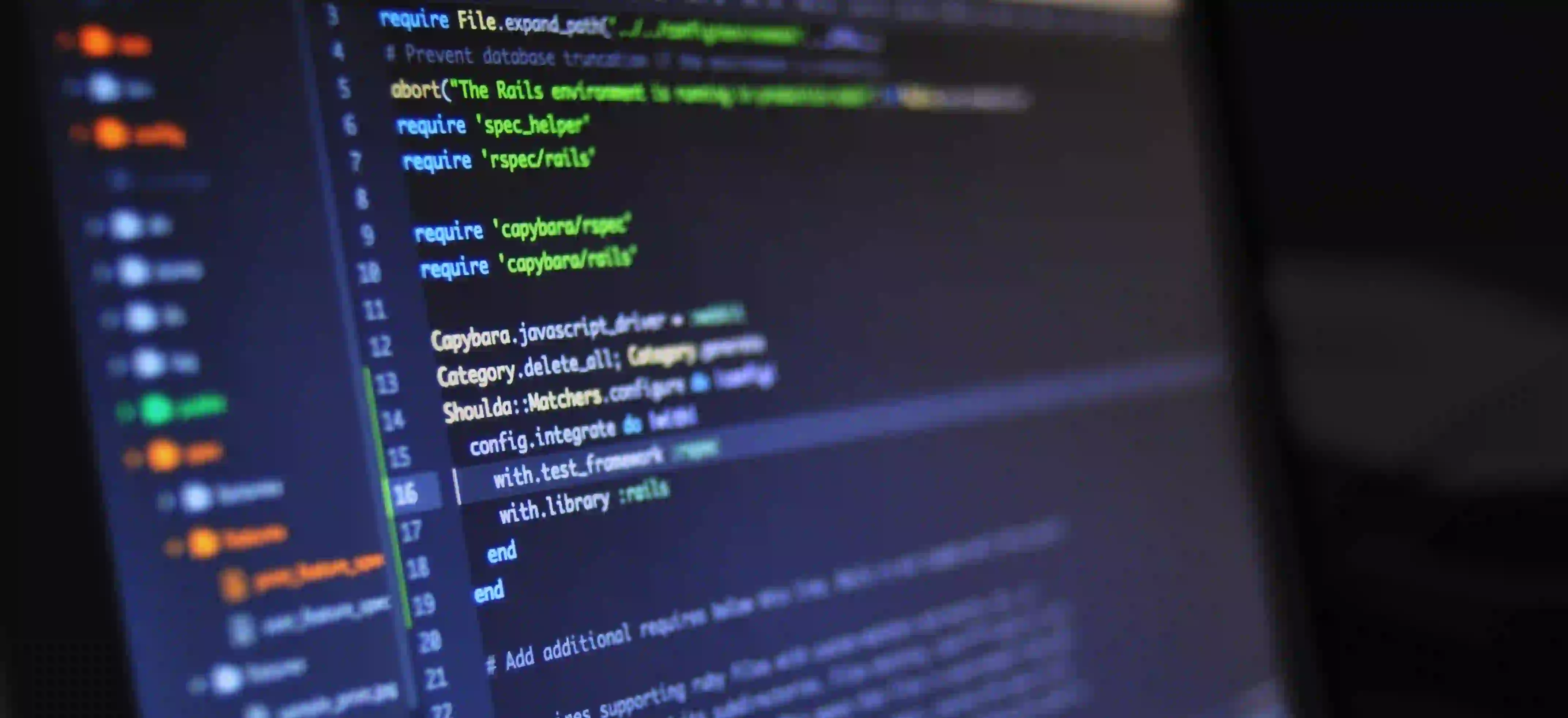
Troubleshooting CDI Bean Injection in JAX-RS Sub-Resources
In the world of Java EE, Contexts and Dependency Injection (CDI) and Java API for RESTful Web Services (JAX-RS) are two essential technologies. CDI allows for the injection of beans, while JAX-RS facilitates the development of RESTful web services. However, integrating CDI beans into JAX-RS sub-resources can sometimes be challenging. In this blog post, we will explore common issues and their solutions when dealing with CDI bean injection in JAX-RS sub-resources.
Understanding CDI Bean Injection in JAX-RS
Before diving into troubleshooting, it's important to understand how CDI bean injection works in JAX-RS. In JAX-RS, resource classes are often backed by CDI beans, allowing for the injection of dependencies using @Inject
. However, when dealing with sub-resources (nested resources within a resource), issues related to CDI bean injection can arise.
Issue: CDI Bean Not Injected in JAX-RS Sub-Resource
One common issue is when a CDI bean is not properly injected into a JAX-RS sub-resource. This can occur due to the scoping of the CDI bean within the context of the main resource, causing the sub-resource to be unaware of the bean's existence.
Solution: Using @Dependent
Scope
To address this issue, it's important to understand the scope of the CDI bean. By default, CDI beans have a scope of @Dependent
, which means the bean's lifecycle is bound to the lifecycle of its injection point. However, in the case of sub-resources, we want the bean to be shared within the entire resource hierarchy.
To achieve this, we can annotate the CDI bean with a broader scope, such as @RequestScoped
or @ApplicationScoped
, ensuring that the bean is available to the entire resource hierarchy, including sub-resources.
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class MyCDIBean {
// Bean implementation
}
By changing the scope of the CDI bean, we ensure that it is accessible to the JAX-RS sub-resource, resolving the injection issue.
Issue: Sub-Resource Not Recognized as CDI Bean
Another issue that developers may encounter is when a JAX-RS sub-resource is not recognized as a CDI bean, preventing the injection of dependencies.
Solution: Using @Path
on Sub-Resource Interface
When creating JAX-RS sub-resources, it's crucial to define an interface for the sub-resource and annotate it with @Path
. If the sub-resource interface is not properly annotated, CDI may not recognize it as a bean, leading to injection issues.
import javax.ws.rs.Path;
@Path("/subresource")
public interface SubResource {
// Sub-resource methods
}
By annotating the sub-resource interface with @Path
, we explicitly denote it as a JAX-RS resource, allowing CDI to recognize it as a bean and enabling proper injection of dependencies.
Issue: Missing @Inject
Annotation in Sub-Resource
In some cases, developers overlook the necessity of annotating sub-resource fields or constructor with @Inject
, resulting in the failure of CDI bean injection.
Solution: Explicit Use of @Inject
To ensure that CDI beans are injected into sub-resources, it's imperative to annotate the fields or constructor of the sub-resource with @Inject
. This explicitly specifies the dependencies that need to be injected.
import javax.inject.Inject;
public class MySubResource implements SubResource {
@Inject
private MyCDIBean myCDIBean;
// Sub-resource methods
}
By incorporating @Inject
in the sub-resource, we clearly indicate the dependencies that should be injected by CDI, resolving any injection-related issues.
A Final Look
Integrating CDI beans into JAX-RS sub-resources may present challenges, but by understanding the intricacies of CDI bean injection within the context of JAX-RS, developers can effectively troubleshoot and resolve issues. By leveraging appropriate bean scopes, annotating sub-resource interfaces, and explicit use of @Inject
, the seamless integration of CDI beans into JAX-RS sub-resources can be achieved.
With a clear understanding of these troubleshooting techniques, developers can streamline the development of robust and scalable RESTful web services within the Java EE ecosystem.
For more in-depth information on CDI and JAX-RS, refer to the following resources:
- CDI Documentation
- JAX-RS Specification