Handling Asynchronous Operations with CompletableFuture
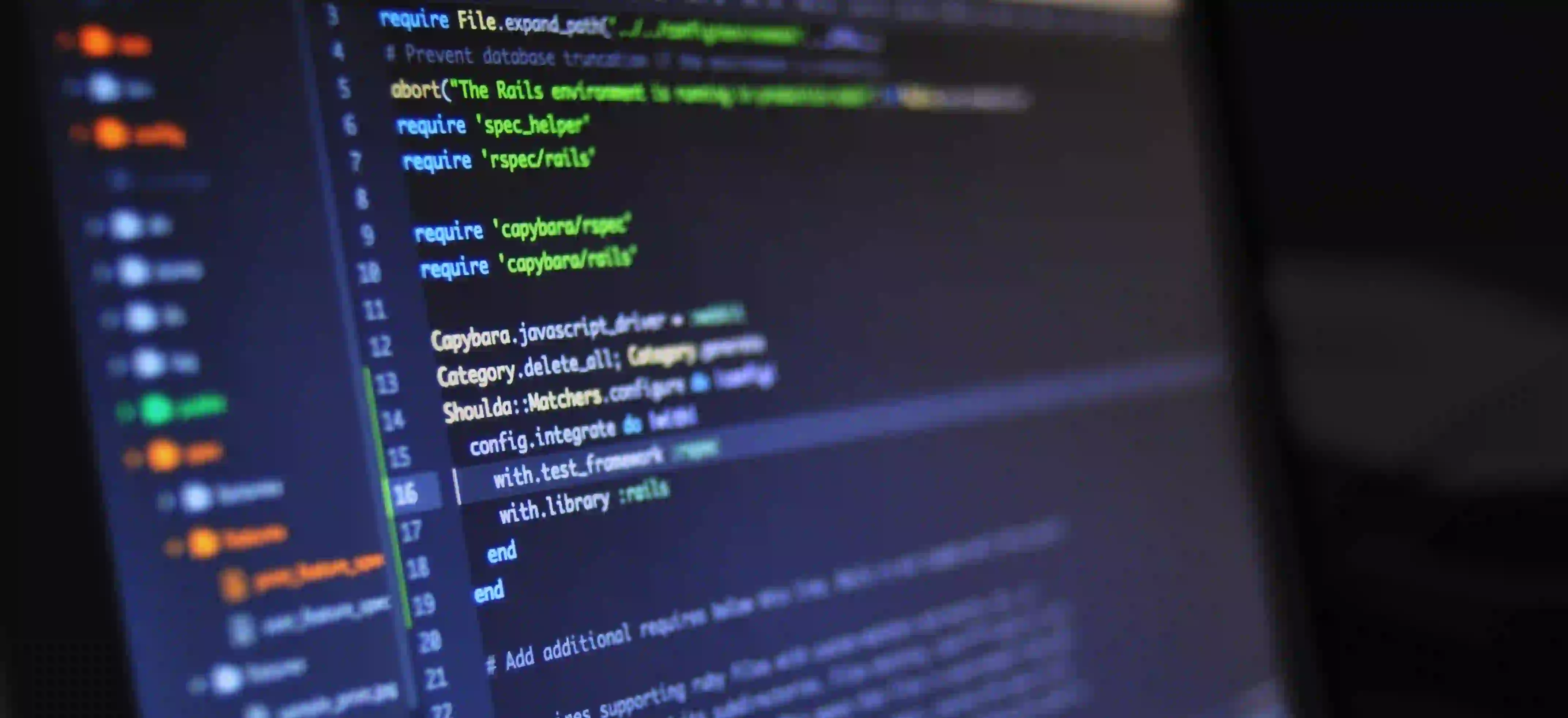
Handling Asynchronous Operations with CompletableFuture
In today's fast-paced world, handling asynchronous operations is crucial for building efficient and responsive applications. Asynchronous programming allows tasks to run parallel to each other, improving overall performance and responsiveness. In Java, the CompletableFuture
class provides a powerful way to work with asynchronous computations. In this article, we will explore how to effectively use CompletableFuture
to handle asynchronous operations in Java.
Understanding CompletableFuture
CompletableFuture
is a class introduced in Java 8 that represents a future result of an asynchronous computation. It allows you to chain asynchronous operations, combine multiple asynchronous tasks, handle errors, and compose complex workflows in a concise and expressive manner.
As its name suggests, CompletableFuture
represents a computation that will be completed in the future, and it provides a rich set of methods for interacting with the result of the computation.
Creating a Simple CompletableFuture
Let's start by creating a simple CompletableFuture
that performs a computation asynchronously. We'll use the supplyAsync
method to run a task in a separate thread and return the result as a CompletableFuture
.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executors;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello, CompletableFuture!");
}
}
In this example, we create a CompletableFuture
using the supplyAsync
method, which takes a Supplier
lambda as an argument. The lambda represents the asynchronous computation that will be executed in a separate thread.
Chaining Asynchronous Operations
One of the most powerful features of CompletableFuture
is the ability to chain multiple asynchronous operations together. This is achieved using methods like thenApply
, thenCompose
, and thenCombine
.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello,")
.thenApplyAsync(result -> result + " CompletableFuture!")
.thenApply(String::toUpperCase);
In this example, we first supply a partial result asynchronously. Then, we use thenApplyAsync
to append another string to the result. Finally, we use thenApply
to transform the result to uppercase. Each thenApply
stage represents a separate asynchronous operation that will be executed upon the completion of the previous stage.
Combining Multiple CompletableFutures
You can also combine the results of multiple CompletableFuture
instances using methods like thenCombine
and thenCompose
. This is useful when you have independent asynchronous tasks that need to be combined to produce a final result.
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> "Hello, ");
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> "CompletableFuture!");
CompletableFuture<String> combinedFuture = future1.thenCombine(future2, (result1, result2) -> result1 + result2);
In this example, we create two independent CompletableFuture
instances and then use thenCombine
to combine their results into a single CompletableFuture
.
Exception Handling
Error handling is an essential aspect of asynchronous programming. CompletableFuture
provides methods like exceptionally
and handle
to handle exceptions that occur during the execution of asynchronous tasks.
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
// Simulate a computation that may throw an exception
if (Math.random() < 0.5) {
throw new RuntimeException("Oops! Something went wrong");
}
return 100;
});
CompletableFuture<Integer> result = future
.exceptionally(ex -> {
System.out.println("Exception occurred: " + ex.getMessage());
return 0; // Default value
});
In this example, we use the exceptionally
method to handle the exception that may occur during the computation. If an exception occurs, we provide a default value of 0 and handle the exception gracefully.
Handling Asynchronous I/O Operations
CompletableFuture
is particularly useful for handling asynchronous I/O operations, such as making HTTP requests or querying databases. By leveraging CompletableFuture
, you can perform I/O operations asynchronously without blocking the main thread.
public CompletableFuture<String> fetchDataAsync() {
return CompletableFuture.supplyAsync(() -> {
// Perform asynchronous I/O operation, such as making an HTTP request
return httpClient.sendAsync(request, BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.join(); // Block and wait for the result
});
}
In this example, we define a method that returns a CompletableFuture
to fetch data asynchronously. We use the sendAsync
method of the HttpClient
class to perform an asynchronous HTTP request and obtain the response body. By using join
, we can block and wait for the result when necessary.
A Final Look
CompletableFuture
is a powerful tool for handling asynchronous operations in Java. Its rich set of methods and combinators allows you to express complex asynchronous workflows in a clear and concise manner. By using CompletableFuture
, you can improve the responsiveness and performance of your Java applications, especially when dealing with I/O-bound tasks.
In this article, we've only scratched the surface of what CompletableFuture
can do. I encourage you to explore the official Java documentation and experiment with CompletableFuture
to fully harness its potential in your projects. I hope this article has provided you with a solid understanding of how to handle asynchronous operations with CompletableFuture
in Java. Happy coding!