Improving Code Reusability with Bridge Design Pattern
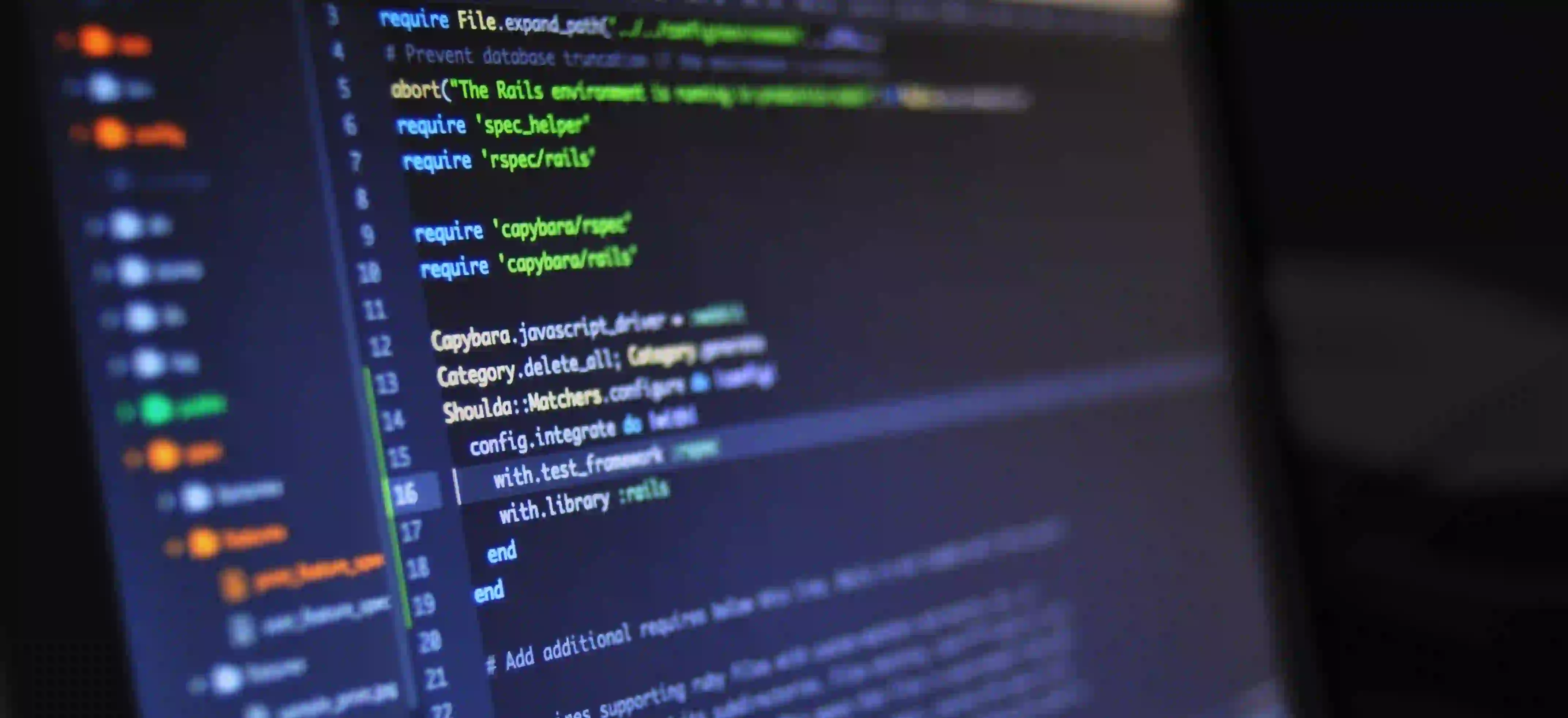
Improving Code Reusability with Bridge Design Pattern
In software development, code reusability is a crucial aspect of creating maintainable and efficient code. It allows developers to use existing code to avoid writing redundant logic, which in turn reduces development time and minimizes the chances of introducing bugs. One of the design patterns that promotes code reusability is the Bridge Design Pattern. This pattern is a structural pattern that decouples an abstraction from its implementation so that the two can vary independently.
In this article, we’ll explore how the Bridge Design Pattern can be used to improve code reusability in Java. We’ll discuss its implementation, advantages, and present a practical example to demonstrate its usage.
Understanding the Bridge Design Pattern
The Bridge Design Pattern is used to separate the abstraction from its implementation, so that they can vary independently. In other words, it enables you to create a bridge between the abstraction and the implementation, allowing the two to evolve separately without being tightly coupled.
Participants of the Bridge Pattern
The Bridge pattern involves the following participants:
- Abstraction: This defines the high-level interface and maintains a reference to an object of type Implementor.
- RefinedAbstraction: This extends the interface defined by Abstraction.
- Implementor: This defines the interface for implementation classes. It doesn’t have to correspond directly to the Abstraction interface and can vary independently.
- ConcreteImplementor: This provides specific implementations of the Implementor interface.
By using the Bridge Pattern, the client can use the Abstraction to interact with the Implementor without being exposed to its implementation details.
Implementation of the Bridge Pattern in Java
Let’s consider an example to understand the implementation of the Bridge Design Pattern in Java. Suppose we are designing a Shape hierarchy, and we want to separate the shape abstraction from its rendering implementation.
Step 1: Define the Implementor Interface
public interface DrawingAPI {
void drawCircle(double x, double y, double radius);
}
The DrawingAPI
interface represents the Implementor, which defines the interface for implementation classes.
Step 2: Create ConcreteImplementor Classes
public class DrawingAPI1 implements DrawingAPI {
// Concrete implementation of DrawingAPI
// ...
}
public class DrawingAPI2 implements DrawingAPI {
// Another concrete implementation of DrawingAPI
// ...
}
The DrawingAPI1
and DrawingAPI2
classes represent ConcreteImplementor, which provides specific implementations of the DrawingAPI
interface.
Step 3: Define the Abstraction and RefinedAbstraction
public abstract class Shape {
protected DrawingAPI drawingAPI;
protected Shape(DrawingAPI drawingAPI) {
this.drawingAPI = drawingAPI;
}
public abstract void draw(); // Abstraction method
}
public class CircleShape extends Shape {
private double x, y, radius;
public CircleShape(double x, double y, double radius, DrawingAPI drawingAPI) {
super(drawingAPI);
this.x = x;
this.y = y;
this.radius = radius;
}
public void draw() {
drawingAPI.drawCircle(x, y, radius);
}
}
The Shape
class represents the Abstraction, which defines the high-level interface, and the CircleShape
class represents the RefinedAbstraction, which extends the interface defined by the Shape
class.
Advantages of the Bridge Design Pattern
The Bridge Design Pattern offers several advantages, including:
- Decoupling Abstraction and Implementation: It allows the abstraction and implementation to vary independently, making the system more flexible.
- Improved Extensibility: It makes it easier to add new abstractions and implementations without modifying the existing code.
- Enhanced Maintainability: It simplifies the maintenance of the codebase by reducing the impact of changes in one part of the system on the other.
Practical Example: GUI Frameworks
A practical example of the Bridge Pattern is seen in GUI frameworks. In Java, Swing and AWT (Abstract Window Toolkit) use the Bridge Pattern to separate the platform-independent components from the platform-specific implementations. For instance, the JButton
class acts as the Abstraction, while the ButtonUI
interface serves as the Implementor.
Bringing It All Together
In conclusion, the Bridge Design Pattern is a powerful tool for promoting code reusability in Java. By decoupling the abstraction from its implementation, it allows for more flexible, maintainable, and extensible code. When designing systems where parts of the system can vary independently, the Bridge Pattern proves to be a valuable asset.
By understanding and implementing the Bridge Pattern, developers can enhance the reusability, maintainability, and flexibility of their code, making it a fundamental pattern in the realm of software design.
To delve deeper into software design patterns and their implementation in Java, check out some highly recommended resources on Design Patterns and Head First Design Patterns.
Remember, the Bridge Design Pattern is just one of many design patterns that can elevate the quality of your code. Exploring other patterns such as the Singleton, Factory Method, and Observer patterns will lead to a deeper understanding of software design and its application in Java.