Overcoming Common Challenges in Agile Development
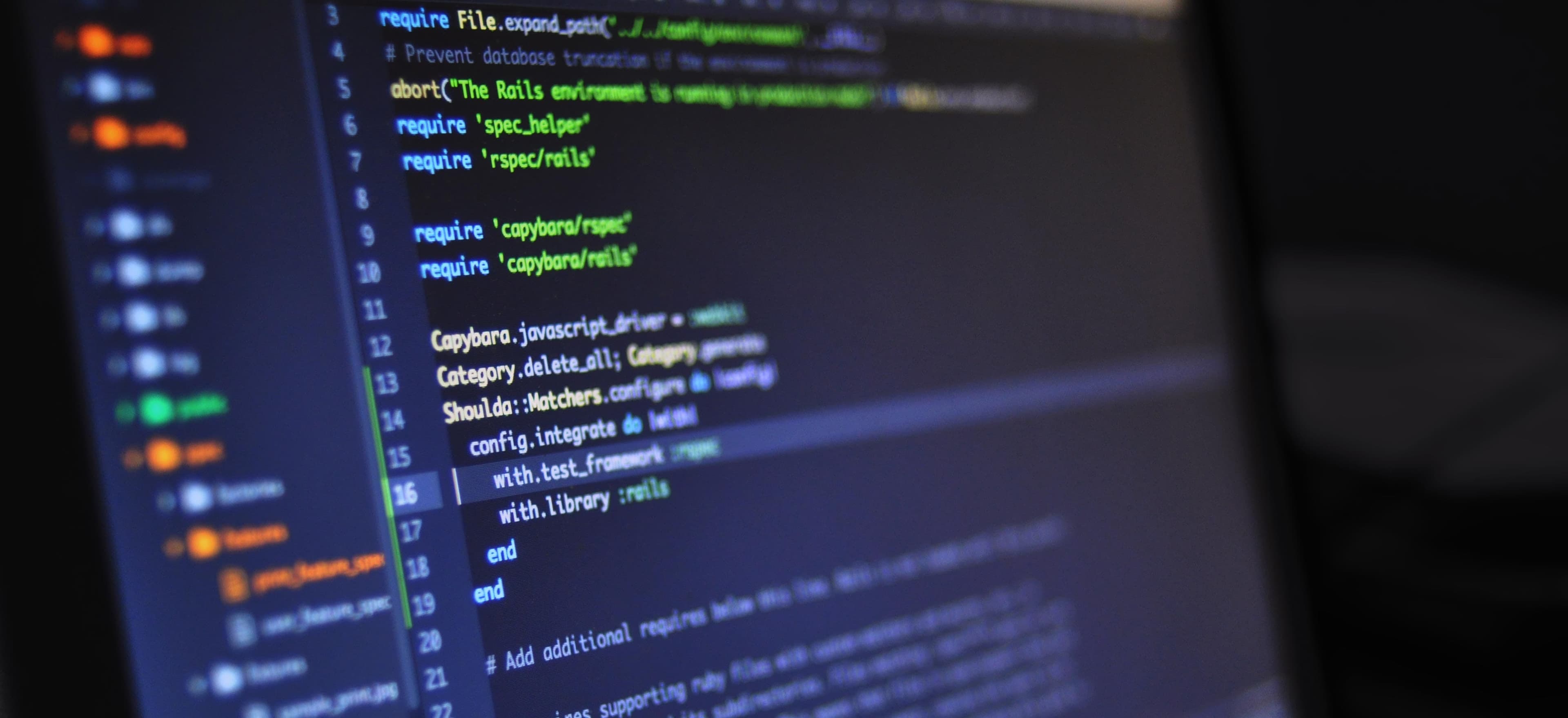
- Published on
Overcoming Common Challenges in Agile Development
Agile development has become the go-to approach for many software development teams due to its flexibility and iterative nature. However, like any methodology, it comes with its own set of challenges. In this post, we will explore some common challenges in Agile development and discuss strategies to overcome them using Java.
1. Maintaining Code Quality
Maintaining code quality is critical in Agile development to ensure that the project stays on track and technical debt doesn't accumulate. In Java, using tools like Checkstyle, PMD, and FindBugs can help enforce coding standards and identify potential issues early in the development process. Integrating these tools into the build process using tools like Maven or Gradle ensures that code quality checks are automated and consistently applied.
// Example of using Checkstyle in a Maven project
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.1</version>
<configuration>
<configLocation>checkstyle.xml</configLocation>
</configuration>
</plugin>
</plugins>
</build>
By integrating these tools into the development workflow, teams can catch potential issues early and maintain code quality throughout the project.
2. Managing Changing Requirements
One of the key principles of Agile is its flexibility in accommodating changing requirements. However, managing these changes while ensuring the stability of the codebase can be challenging. In Java, using a combination of design patterns such as Strategy and Factory can make the code more adaptable to change. Additionally, employing frameworks like Spring that support inversion of control (IoC) and dependency injection can make the code more modular and easier to modify.
// Example of using Strategy pattern in Java
public interface PaymentStrategy {
void pay(int amount);
}
public class CreditCardPaymentStrategy implements PaymentStrategy {
public void pay(int amount) {
// Implement credit card payment
}
}
public class PayPalPaymentStrategy implements PaymentStrategy {
public void pay(int amount) {
// Implement PayPal payment
}
}
By designing the code to be modular and following best practices such as dependency injection, teams can more effectively manage changing requirements without causing extensive rework.
3. Communication and Collaboration
Effective communication and collaboration are essential for Agile teams, especially in distributed or remote setups. In Java, using tools like Slack, Microsoft Teams, or Zoom for real-time communication can bridge the gap between team members. Leveraging version control systems like Git for collaborative development allows teams to seamlessly work on the same codebase without conflicts.
// Example of Git workflow for collaborative development
$ git pull // Get the latest changes
// Make and commit your changes
$ git push // Share your changes with the team
By establishing clear communication channels and utilizing collaboration tools, teams can overcome the challenges of working together in an Agile environment.
4. Testing and Continuous Integration
Ensuring that the code functions as expected and integrating changes seamlessly are integral parts of Agile development. In Java, utilizing tools like JUnit for unit testing and Jenkins for continuous integration can automate the testing and integration processes. By writing comprehensive unit tests and integrating them into the build pipeline, teams can catch regressions early and maintain a reliable codebase.
// Example of a JUnit test in Java
public class MathUtilsTest {
@Test
public void testAdd() {
// Test the add method
}
}
By automating testing and continuous integration, teams can ensure that the codebase remains stable and that new changes are integrated smoothly.
A Final Look
Agile development, while beneficial, comes with its own set of challenges. By addressing these challenges with the right tools, practices, and strategies, teams can navigate the Agile landscape more effectively. In Java, leveraging code quality tools, design patterns, communication and collaboration tools, and testing frameworks can help teams overcome common Agile challenges and deliver high-quality software in a more iterative and adaptive manner.
Incorporating these practices into Agile development can lead to more efficient processes, higher-quality code, and better collaboration within the team. So, when faced with Agile challenges, remember that Java offers a robust ecosystem of tools and best practices to overcome them.