The Challenge of Managing Dual Data Migrations
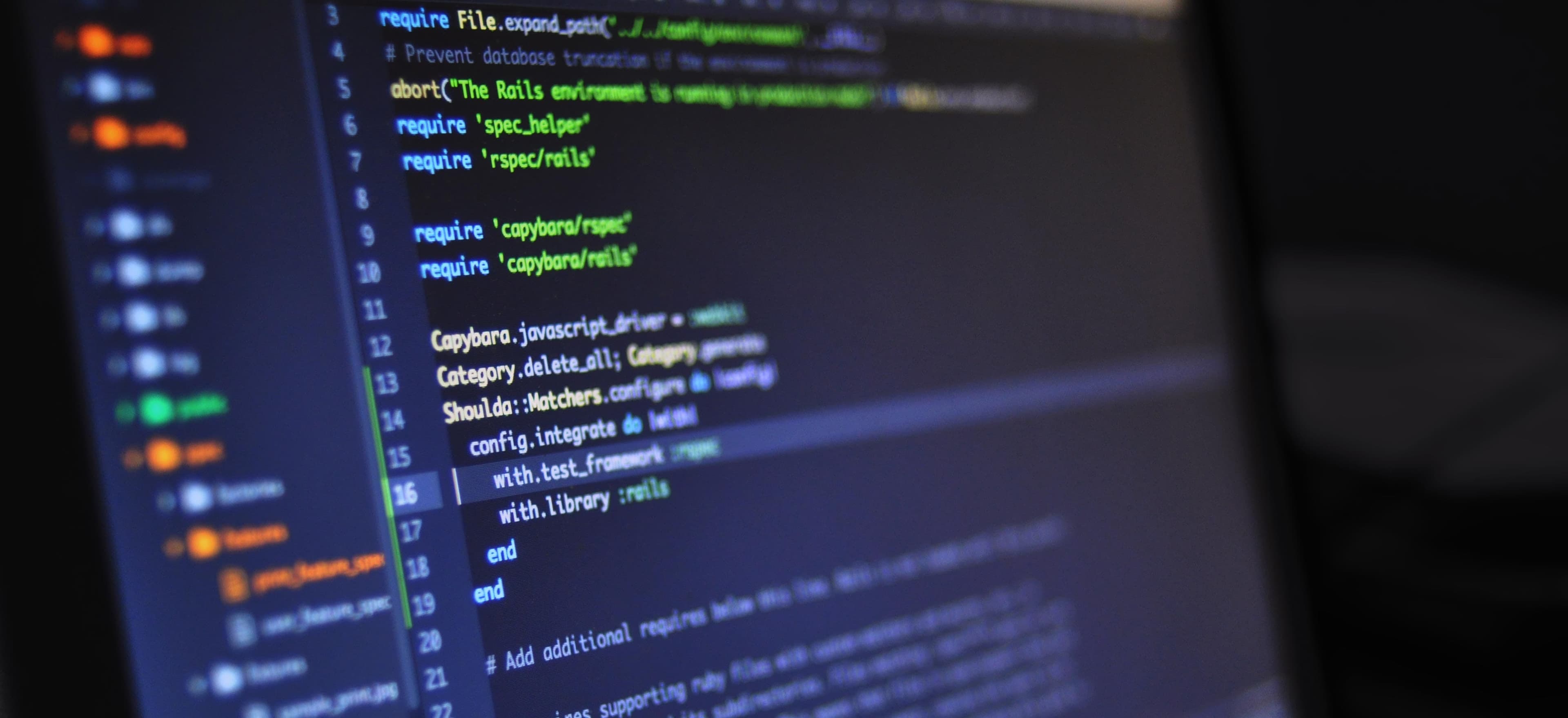
- Published on
The Challenge of Managing Dual Data Migrations
When dealing with complex enterprise systems, one of the biggest hurdles for developers and DevOps teams is managing dual data migrations. This process involves moving data from one type of database to another, which is a common occurrence when transitioning from legacy systems to modern, more efficient databases. In this article, we will explore the intricacies of dual data migrations, the challenges they present, and best practices for managing them effectively.
Understanding Dual Data Migrations
Dual data migrations, as the name suggests, involve migrating data from one database to another in a way that ensures both systems remain operational during the transition. This is particularly challenging because it requires not only moving the data itself but also maintaining data integrity, ensuring minimal downtime, and managing potential data loss or corruption.
The process typically involves extracting data from the source database, transforming it into a format compatible with the target database, and then loading it into the new system. This often requires careful planning, thorough testing, and the ability to rollback in case of unforeseen issues.
The Challenges
1. Data Consistency
Maintaining data consistency during a dual data migration is critical. Inconsistent data can lead to erroneous results, system instability, and ultimately, loss of trust in the new system. Achieving this consistency while the source database continues to receive and process new data adds another layer of complexity.
2. Downtime Minimization
Minimizing downtime is a major challenge during dual data migrations, especially in systems that require 24/7 availability. The migration process should be designed to allow the source system to remain operational as much as possible, even as the data is being migrated to the new database.
3. Rollback Strategy
Despite careful planning, unforeseen issues can arise during the migration. Having a robust rollback strategy in place is crucial to ensure that the system can revert to its previous state with minimal impact in case of data corruption or other migration-related issues.
Best Practices for Managing Dual Data Migrations
1. Thorough Planning
Before embarking on a dual data migration, it is essential to conduct a comprehensive analysis of the data schemas, dependencies, and potential pitfalls. Understanding the intricacies of the source and target databases is crucial for a successful migration.
2. Data Validation
Validating the data before, during, and after the migration is crucial. Implementing data validation checks and ensuring data integrity throughout the process can help identify and mitigate issues early on.
3. Incremental Data Migration
Instead of attempting to migrate the entire dataset at once, consider an incremental approach. This involves migrating data in smaller, manageable batches, allowing for better control and the ability to course-correct if issues arise.
4. Utilize Automated Testing
Implement automated testing for data consistency, integrity, and performance. This can help catch potential issues early and ensure that the migrated data meets the expected criteria.
5. Monitoring and Alerting
Continuous monitoring of the migration process is essential. Implement robust monitoring and alerting systems to detect anomalies, bottlenecks, or failures in real-time.
Sample Code for Incremental Data Migration in Java
public class DataMigrator {
public void migrateDataIncrementally() {
// Retrieve data from source database in manageable batches
List<DataBatch> dataBatches = sourceDatabase.retrieveDataInBatches();
for (DataBatch batch : dataBatches) {
// Transform the data to match the format of the target database
Data transformedData = transformData(batch);
// Load the transformed data into the target database
targetDatabase.loadData(transformedData);
}
}
private Data transformData(DataBatch batch) {
// Transformation logic to adapt data to the target database schema
// ...
}
}
In the sample Java code above, the DataMigrator
class demonstrates an incremental approach to data migration. By retrieving data in manageable batches and transforming it before loading it into the target database, the code exemplifies a strategy for minimizing downtime and controlling the migration process in a granular manner.
Closing Remarks
Dual data migrations are complex endeavors that demand meticulous planning, rigorous testing, and strategic execution. By understanding the challenges they present and adopting best practices such as thorough planning, data validation, incremental migration, automated testing, and monitoring, development teams can navigate the intricacies of dual data migrations more effectively.
Successfully managing dual data migrations not only ensures a smooth transition to a new database but also minimizes the impact on ongoing operations, data integrity, and overall system stability.
With careful consideration and a well-structured approach, the challenges of dual data migrations can be overcome, paving the way for a more efficient and reliable database infrastructure.
Remember, the key to successful dual data migrations lies in proactive planning, meticulous execution, and the flexibility to adapt to unforeseen circumstances.
Checkout our other articles