Profile-based Logging in Spring Boot
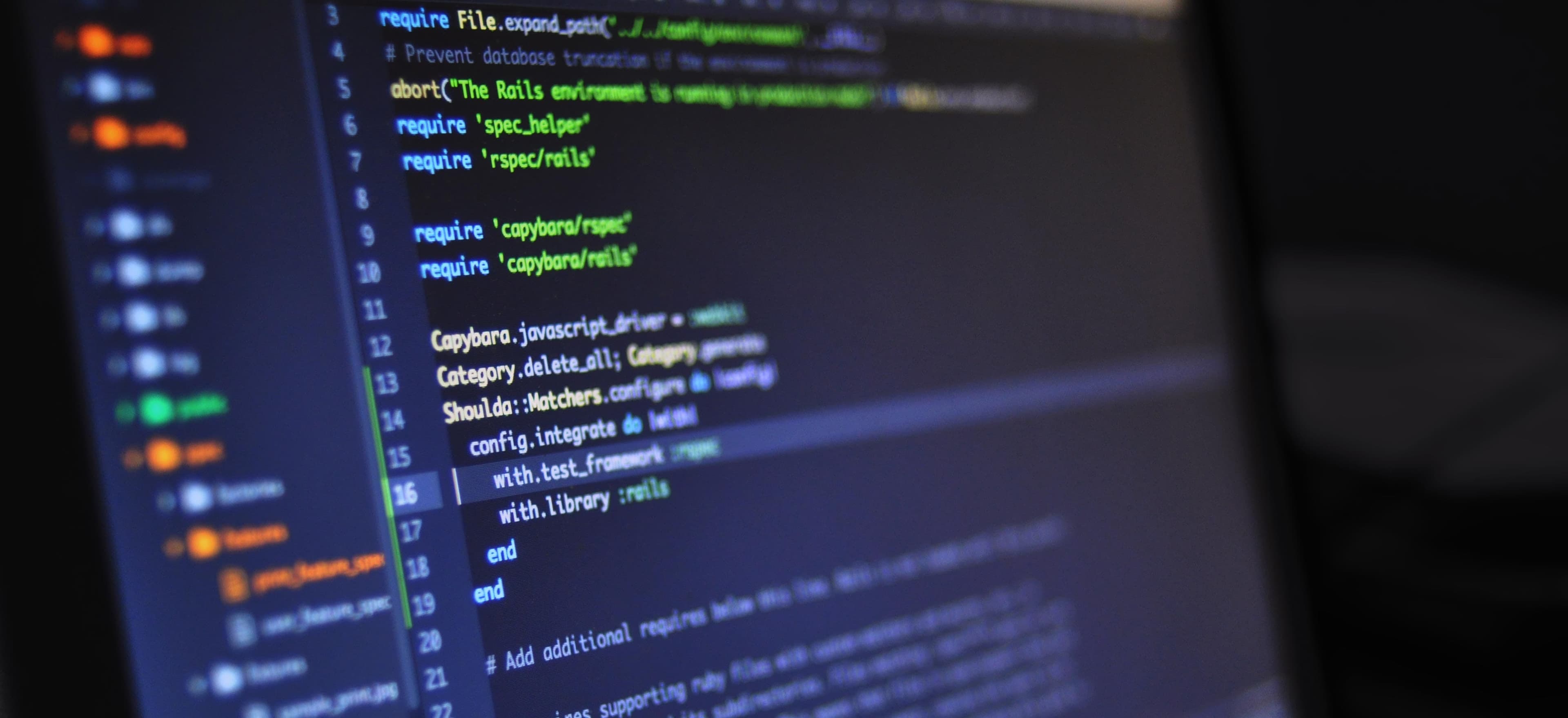
- Published on
Achieve Fine-grained Logging in Spring Boot with Profile-based Configuration
Logging is an essential aspect of application development, allowing us to track and understand the behavior of our code. Spring Boot provides a flexible and powerful way to configure logging, which can be further enhanced by leveraging profiles.
In this blog post, we'll dive into the concepts of profile-based logging in Spring Boot, exploring how to utilize different logging configurations for various environments or purposes. We'll walk through the implementation of profile-based logging, from setting up logback-spring.xml to utilizing it in a Spring Boot application.
Understanding Profile-based Logging
In Spring Boot, profiles allow us to define sets of configurations that can be activated based on the environment or specific conditions. By harnessing profiles, we have the capability to customize logging behavior according to different deployment environments or specific use cases.
By defining specific logging configurations for different profiles, we can ensure that the application logs differently when running in development, testing, or production environments, or when targeting specific functionality such as performance monitoring or debugging.
Setting up Logback-spring.xml for Profile-based Logging
The first step in implementing profile-based logging is to set up the logging configuration file, logback-spring.xml, which Spring Boot uses for logging configuration.
Let's consider a scenario where we want to have different logging patterns for two profiles: "development" and "production".
Here's how you can set up the logback-spring.xml to achieve this:
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<springProfile name="development">
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="CONSOLE" />
</root>
</springProfile>
<springProfile name="production">
<appender name="FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<encoder>
<pattern>%d %p %C{1.} [%t] %m%n</pattern>
</encoder>
<rollingPolicy class="ch.qos.logback.core.rolling.SizeAndTimeBasedRollingPolicy">
<!-- Rolling policy configuration -->
</rollingPolicy>
</appender>
<root level="info">
<appender-ref ref="FILE" />
</root>
</springProfile>
</configuration>
In this setup, we define two different logging configurations based on the "development" and "production" profiles. For the "development" profile, we configure a console appender with a specific logging pattern and set the log level to debug. For the "production" profile, we configure a rolling file appender with a different logging pattern and set the log level to info.
By utilizing the springProfile
element in the logback-spring.xml, we can encapsulate profile-specific logging configurations, enabling Spring Boot to activate the relevant configuration based on the active profile.
Activating Profiles in Spring Boot
To configure and activate profiles in a Spring Boot application, we can utilize various methods such as environment variables, system properties, or configuration files.
Let's explore how we can activate the "development" and "production" profiles for our logging configurations.
Using application.properties or application.yml
In the application.properties
or application.yml
file, we can specify the active profiles using the spring.profiles.active
property.
For example, in application.properties
:
spring.profiles.active=development
In application.yml
:
spring:
profiles:
active: development
By setting the spring.profiles.active
property to the desired profile, we can activate the corresponding logging configuration defined in the logback-spring.xml for that profile.
Command-line Arguments
We can also activate profiles using command-line arguments when running the Spring Boot application.
For example, to activate the "production" profile:
java -jar -Dspring.profiles.active=production myapplication.jar
By passing the -Dspring.profiles.active
argument with the desired profile, we can activate the corresponding logging configuration for that profile during runtime.
Environment Variable
Setting an environment variable is another way to activate profiles.
For instance, in a Unix-based environment:
export SPRING_PROFILES_ACTIVE=production
By defining the environment variable SPRING_PROFILES_ACTIVE
with the desired profile, we can activate the corresponding logging configuration for that profile.
Leveraging Profile-based Logging in the Application
After setting up profile-based logging configurations and activating the desired profiles, we can utilize profile-specific logging in our application.
For instance, we could have a service class where we want to log certain information differently based on the active profile:
@Service
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
// ...
public void performAction() {
// Business logic
// Logging based on profile
if (logger.isDebugEnabled()) {
// Log detailed information for development profile
logger.debug("Debug information for development");
} else {
// Log summary information for production profile
logger.info("Performed action for production");
}
}
}
In the above example, we use the logger to differentiate the logging behavior based on the active profile. For the "development" profile, we log detailed debug information, while for the "production" profile, we log a summary at the info level.
By incorporating profile-specific logging in the application code, we can effectively tailor the logging output based on the active profile, promoting finer control over the application's logging behavior.
Wrapping Up
In this blog post, we've delved into the concept of profile-based logging in Spring Boot, learning how to configure profile-specific logging using logback-spring.xml and activate profiles via different methods. By leveraging profile-based logging, we can fine-tune the logging behavior of our Spring Boot applications, catering to the specific requirements of different environments and use cases.
Profile-based logging empowers us to achieve granular control over our application's logging, ensuring that the right information is captured at the right time, without cluttering the log output. As a result, we can effectively streamline our logging practices and enhance the overall observability and maintainability of our Spring Boot applications.