Ensuring Consistency in Data Across Microservices
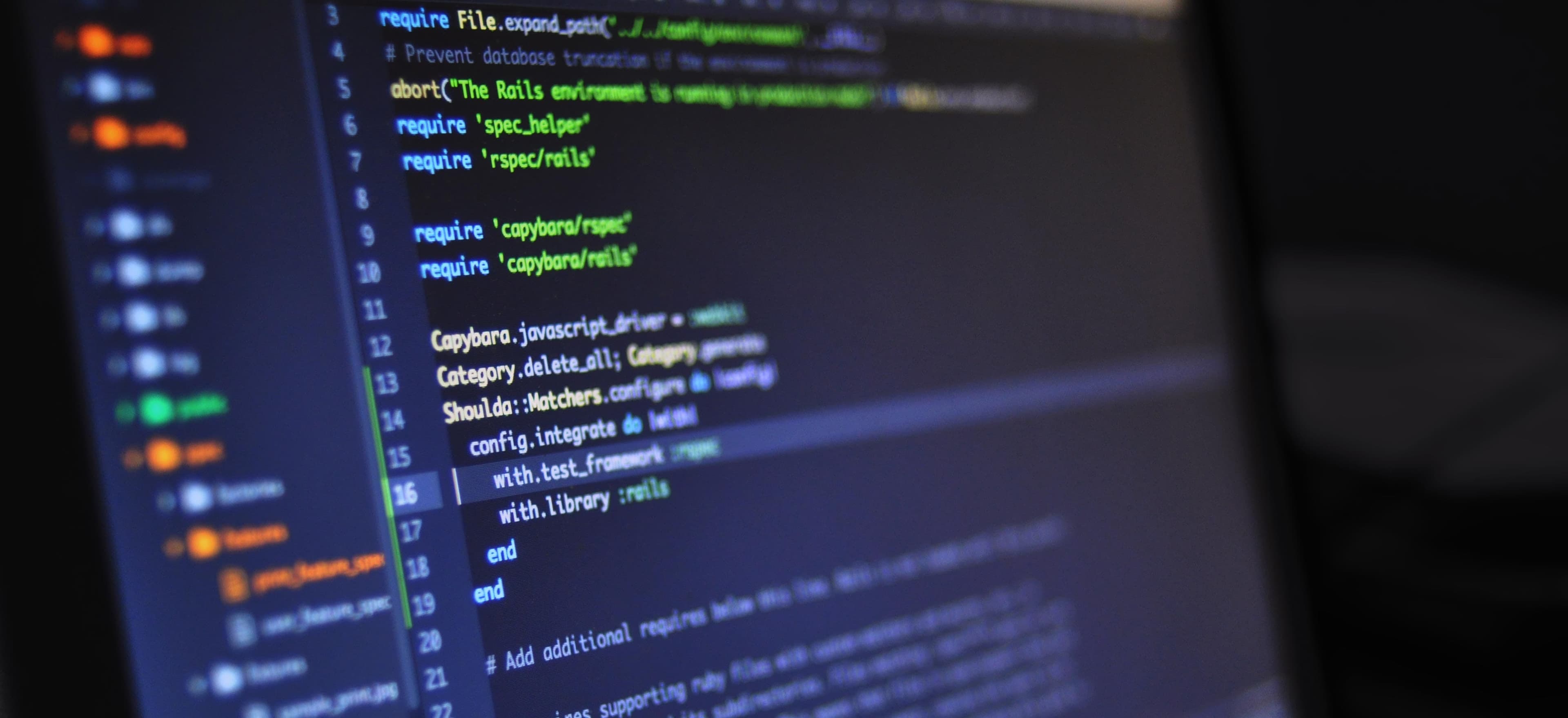
- Published on
Achieving Data Consistency in Microservices with Java
In a microservices architecture, where complex applications are composed of small, independent processes communicating with each other, maintaining data consistency is crucial. In this blog post, we will explore strategies to achieve data consistency in microservices using Java.
The Challenge of Data Consistency in Microservices
One of the main challenges in a microservices architecture is ensuring that data remains consistent across multiple services. Since each service has its database, it's possible for data to become inconsistent if not managed properly. For example, when an operation involves multiple services, a failure in one service could leave the data in an inconsistent state.
1. Synchronous Communication
Using synchronous communication between microservices can help ensure data consistency. When one microservice initiates an operation that involves other services, it can wait for the responses from those services before completing the operation. In Java, this can be achieved using frameworks like Spring Boot for building synchronous microservices.
// Example of synchronous communication using RestTemplate in Spring Boot
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.exchange(
"http://service2/api/resource",
HttpMethod.GET,
null,
String.class);
However, synchronous communication can introduce complexities and increase latency, especially in scenarios where multiple services need to be involved in a single operation.
2. Asynchronous Event-Driven Communication
Utilizing asynchronous event-driven communication can help decouple services and improve scalability. When one service performs an operation that affects another service, it can publish an event, allowing other services to consume and react to the event. In Java, frameworks like Kafka or RabbitMQ can be used for building event-driven microservices.
// Example of publishing an event using Kafka in Java
producer.send(new ProducerRecord<String, String>("topic", "message"));
By using asynchronous communication, services can continue to operate independently, reducing the risk of data consistency issues while also improving the overall system's responsiveness.
3. Distributed Transactions
In some cases, maintaining strong consistency across multiple services may require the use of distributed transactions. Java provides support for distributed transactions through Java Transaction API (JTA) and frameworks like Atomikos or Bitronix. With distributed transactions, it's possible to coordinate multiple operations across different services as a single atomic transaction.
// Example of using JTA with Atomikos for distributed transactions
UserTransaction userTransaction = new UserTransactionImp();
userTransaction.begin();
// Perform operations with multiple databases or services
userTransaction.commit();
However, it's important to note that distributed transactions can introduce complexities and performance overhead, and should be used judiciously.
4. Idempotency
Implementing idempotency in microservices can help mitigate the impact of inconsistent data. By designing operations to be idempotent, even if an operation is executed multiple times, the result will be the same as if it had been executed once. In Java, idempotent operations can be achieved by designing APIs and services with idempotent behavior.
// Example of idempotent API endpoint in Spring MVC
@RequestMapping(value = "/resource", method = RequestMethod.POST)
public ResponseEntity<String> createResource(@RequestBody Resource resource) {
// Check if the resource already exists
// If exists, return the existing resource
// If not, create a new resource
}
Designing idempotent operations not only helps in handling potential duplicates and retries but also improves the robustness of the system in the face of network issues or failures.
My Closing Thoughts on the Matter
Maintaining data consistency in a microservices architecture is a multifaceted challenge that requires careful consideration of trade-offs and the selection of appropriate strategies. By using synchronous communication, asynchronous event-driven communication, distributed transactions, and idempotency, Java developers can navigate the complexities of data consistency in microservices effectively.
By addressing data consistency concerns in a microservices architecture, developers can build resilient and reliable systems that deliver on the promises of scalability and agility inherent in microservices.
In conclusion, the ever-evolving landscape of microservices and data consistency warrants continual exploration and adaptation, and Java developers are well-equipped with a variety of tools and approaches to tackle these challenges effectively.
For further exploration, you can dive deeper into the concepts of synchronous and asynchronous communication in microservices, learn more about Java Transaction API (JTA), and discover best practices for implementing idempotent APIs.