Handling LazyInitializationException in Hibernate
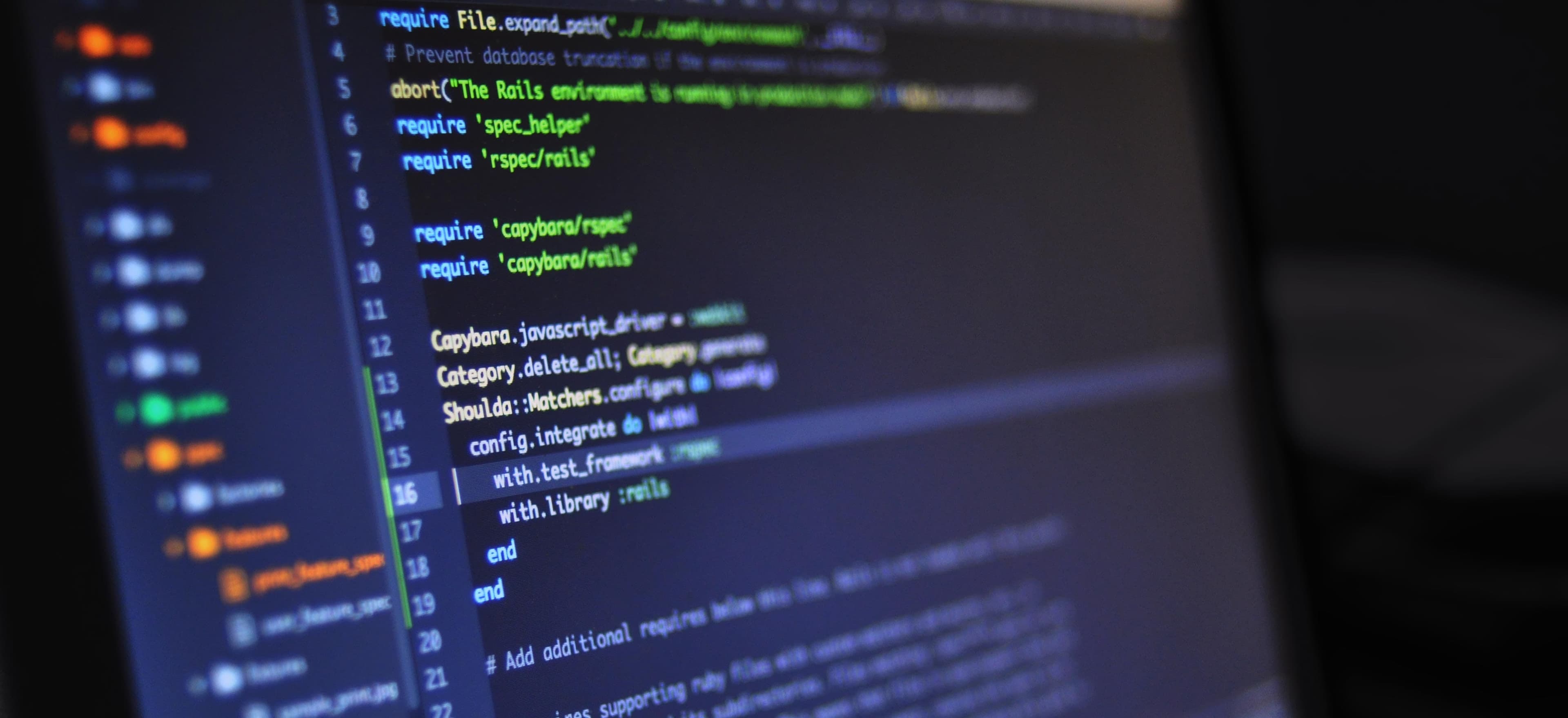
- Published on
Handling LazyInitializationException in Hibernate
Hibernate is a powerful, high-performance Java persistence framework widely used for managing relational databases in enterprise applications. It leverages the concept of lazy loading to optimize database operations. However, lazy loading can sometimes lead to a LazyInitializationException
when working with the retrieved entities outside the scope of a Hibernate session. In this blog post, we'll delve into strategies for effectively handling this exception to ensure smooth interactions with Hibernate-managed entities.
Understanding Lazy Loading
In Hibernate, lazy loading is a mechanism where the associated entities or collections are not immediately loaded from the database when an entity is fetched. Instead, they are loaded only when accessed for the first time. This approach enhances performance by avoiding unnecessary data retrieval and is a key feature of Hibernate.
@Entity
public class Author {
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Book> books;
// Other fields and methods
}
In the above example, the books
collection is configured for lazy loading. When an Author
entity is retrieved, the books
collection will not be loaded until it is accessed.
Dealing with LazyInitializationException
The LazyInitializationException
is a runtime exception that occurs when an attempt is made to access a lazily loaded property or collection outside the scope of an active Hibernate session. This commonly happens in a different layer of the application, such as presentation or service layer, where the Hibernate session used for fetching the entity is already closed.
Option 1: Using Hibernate.initialize()
One way to resolve the LazyInitializationException
is by explicitly initializing the lazy property or collection within a transactional context using Hibernate.initialize()
. This approach ensures that the necessary data is loaded before the session is closed, preventing subsequent access issues.
@Transactional
public AuthorDTO getAuthorWithBooks(Long authorId) {
Author author = authorRepository.findById(authorId);
Hibernate.initialize(author.getBooks());
return convertToDTO(author);
}
In this example, Hibernate.initialize(author.getBooks())
forces the books collection to be loaded within the existing Hibernate session, effectively addressing the lazy initialization problem.
Option 2: Using Open Session in View Pattern
The Open Session in View (OSIV) pattern involves keeping the Hibernate session open until the view layer rendering is completed. This approach ensures that lazy loading can still function as expected in the presentation layer, as the session remains active during the entire request lifecycle.
To enable OSIV in a Spring Boot application, you can configure the OpenEntityManagerInViewIntercepto
r as follows:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addWebRequestInterceptor(openEntityManagerInViewInterceptor());
}
@Bean
public OpenEntityManagerInViewInterceptor openEntityManagerInViewInterceptor() {
OpenEntityManagerInViewInterceptor osivInterceptor = new OpenEntityManagerInViewInterceptor();
return osivInterceptor;
}
}
By adopting the OSIV pattern, you can mitigate LazyInitializationException
by maintaining an open session throughout the request-handling process.
Option 3: Using DTO Projections
Another effective strategy to avoid LazyInitializationException
is to employ Data Transfer Object (DTO) projections. Instead of directly exposing Hibernate-managed entities to the presentation layer, you can create DTOs containing only the necessary data, thus preemptively circumventing lazy loading issues.
public class AuthorDTO {
private String name;
private List<String> bookTitles;
// Getters and setters
}
By explicitly defining the data to be included in the DTOs and fetching only the required data in the service layer, you can sidestep lazy loading problems altogether.
Best Practices
-
Avoid Long-Running Sessions: Minimize the duration of Hibernate sessions to reduce the likelihood of running into
LazyInitializationException
. Acquire the necessary data within a session and avoid keeping sessions open for extended periods. -
Consider Eager Loading: Evaluate the possibility of using eager loading for specific associations where the benefits outweigh the performance implications. This approach can preemptively fetch related entities, eliminating lazy loading issues.
-
Opt for Fetch Joins: Utilize fetch joins in HQL or Criteria API queries to fetch associated entities eagerly in a single query. This can enhance performance and mitigate lazy loading exceptions.
-
Use Session-per-Request: Implement a session-per-request strategy to ensure that an active session exists throughout the duration of a request, effectively addressing lazy loading challenges in web applications.
The Last Word
Handling LazyInitializationException
in Hibernate necessitates a comprehensive understanding of lazy loading mechanisms and the adoption of appropriate strategies. Whether through explicit initialization, OSIV pattern, DTO projections, or adherence to best practices, mitigating lazy loading issues is fundamental to maintaining robust and resilient Hibernate-based applications. By incorporating these approaches into your development workflow, you can effectively manage lazy loading challenges and ensure seamless interactions with Hibernate-managed entities.
Remember, mastering the art of handling LazyInitializationException
is crucial for harnessing the full potential of Hibernate's performance optimizations while maintaining application integrity. With these strategies at your disposal, you are well-equipped to navigate and resolve LazyInitializationException
effectively in your Hibernate projects.