Detecting Duplicate Nodes in Neo4j Using Cypher
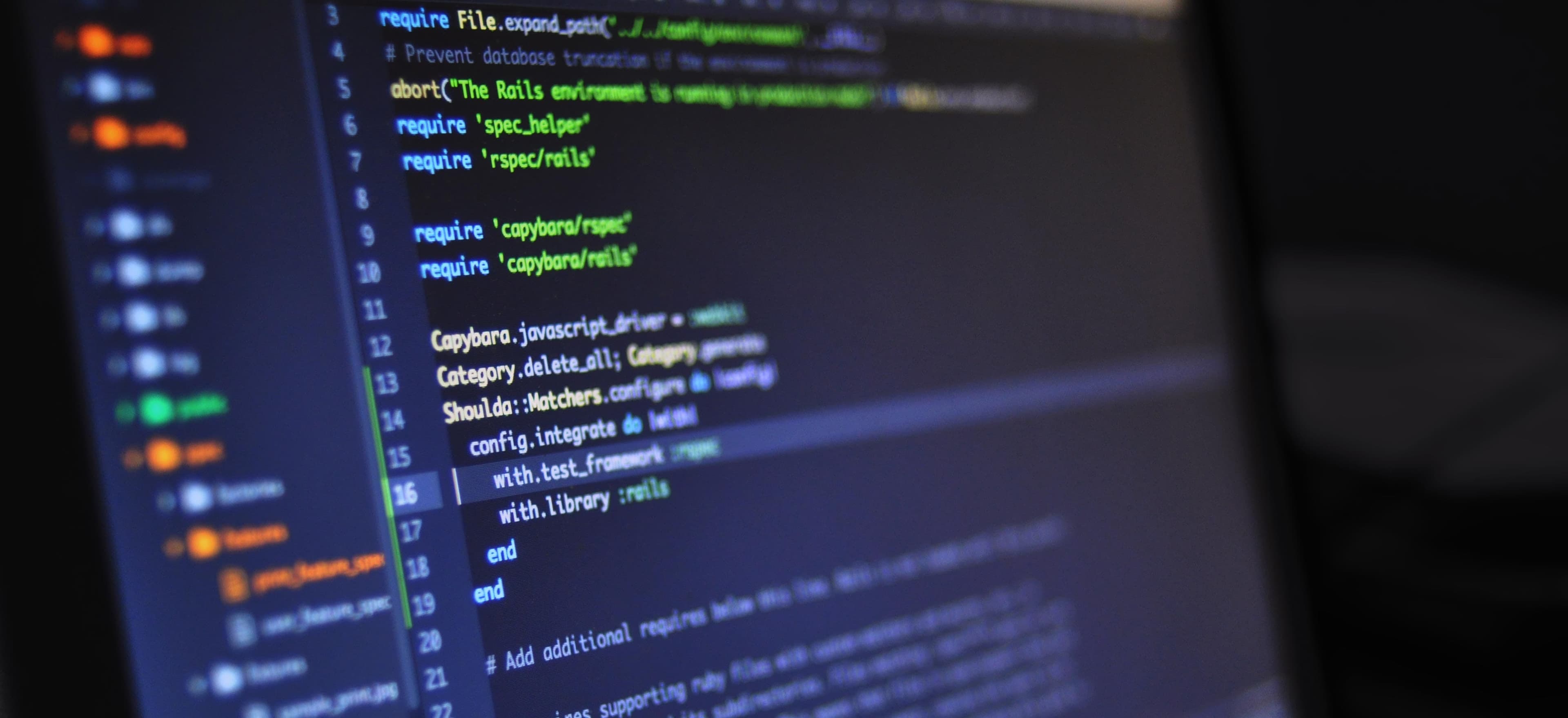
- Published on
Detecting Duplicate Nodes in Neo4j Using Cypher
When working with large datasets in a Neo4j graph database, it's not uncommon to come across duplicate nodes. These duplicate nodes can cause inconsistencies in the data and may need to be identified and resolved. In this blog post, we'll explore how to detect duplicate nodes in Neo4j using Cypher, the query language for Neo4j.
The Challenge of Duplicates in Graph Databases
In graph databases like Neo4j, each node is unique based on its internal identifier. However, duplicate nodes can still occur when data is imported or merged from various sources. These duplicates can lead to inaccuracies in query results and introduce inefficiencies in data processing.
Detecting and managing duplicate nodes is a critical part of data quality assurance and maintenance in Neo4j. Fortunately, Cypher provides powerful tools for identifying and resolving duplicate nodes within the graph database.
Using Cypher to Detect Duplicate Nodes
To begin detecting duplicate nodes in Neo4j, we can leverage Cypher's querying capabilities to compare nodes based on their properties. Let's consider a scenario where we have a Person
node with name
and email
properties. We want to find all the duplicate Person
nodes based on their email
property.
MATCH (p:Person)
WITH p.email AS email, collect(p) AS nodes
WHERE size(nodes) > 1
RETURN email, nodes
In this Cypher query, we first match all Person
nodes and then group them by their email
property using the collect
function. The WITH
clause allows us to pass the email
and the collected nodes to the next part of the query. We then filter the collected nodes to only include those with more than one occurrence, effectively identifying the duplicate Person
nodes based on their email
property.
Why This Approach Works
The MATCH
clause is used to find all Person
nodes in the graph, and the WITH
clause is utilized to process and prepare the data for further filtering. The collect
function is a crucial tool in grouping nodes based on their shared properties, allowing us to compare and identify duplicates efficiently.
By comparing the collected nodes' size to 1, we can easily filter out the unique nodes and focus on the duplicate instances. This approach provides a clear and concise method for detecting duplicate nodes in Neo4j, improving data quality and reliability.
Handling Large Datasets
When dealing with large datasets, the aforementioned approach may become resource-intensive due to the collection of all nodes. To optimize the process for large datasets, we can utilize the apoc.coll.toSet
function from the APOC library, which efficiently aggregates and filters duplicate values.
CALL apoc.cypher.run('
MATCH (p:Person)
RETURN p.email AS email, collect(p) AS nodes
') YIELD value
WITH apoc.coll.toSet(value.email) AS uniqueEmails, value.nodes AS nodes
UNWIND nodes AS node
MATCH (duplicate:Person {email: node.email}) WHERE NOT ID(duplicate) IN [id IN uniqueEmails | id]
RETURN duplicate
In this optimized query, we use the apoc.cypher.run
procedure to efficiently collect the Person
nodes and their respective emails. The apoc.coll.toSet
function then extracts unique email values, optimizing the identification of duplicate nodes. By excluding the IDs of unique nodes, we can accurately retrieve the duplicate Person
nodes based on their emails.
Why Optimization Matters
Large datasets require efficient query strategies to minimize resource consumption and maximize performance. The optimized approach leverages the APOC library's functions to streamline the identification of duplicate nodes, ensuring that the process remains scalable and responsive even with substantial data volumes.
Summary
Detecting duplicate nodes in Neo4j is crucial for maintaining data integrity and ensuring accurate query results. Cypher's querying capabilities, combined with APOC library functions, provide powerful tools for identifying and managing duplicate nodes within a Neo4j graph database. By leveraging the collect
and apoc.coll.toSet
functions, we can efficiently compare nodes based on their properties and optimize the detection process, even with large datasets.
Ensuring data quality through the identification and resolution of duplicate nodes is a fundamental aspect of managing Neo4j graph databases, and Cypher equips us with the necessary tools to address this challenge effectively.
By utilizing the approaches outlined in this post, you can streamline the process of detecting and resolving duplicate nodes in Neo4j, contributing to a more robust and reliable graph database environment.
Remember, maintaining a clean and consistent database is key to deriving valuable insights and ensuring the overall success of your Neo4j-powered applications.
For further insights into Neo4j and Cypher, you can explore the official Neo4j documentation and the rich array of resources available on the Neo4j website. Happy coding!
Now, go forth and optimize your graph database with confidence!