Understanding Future Failures in Scala: Common Pitfalls
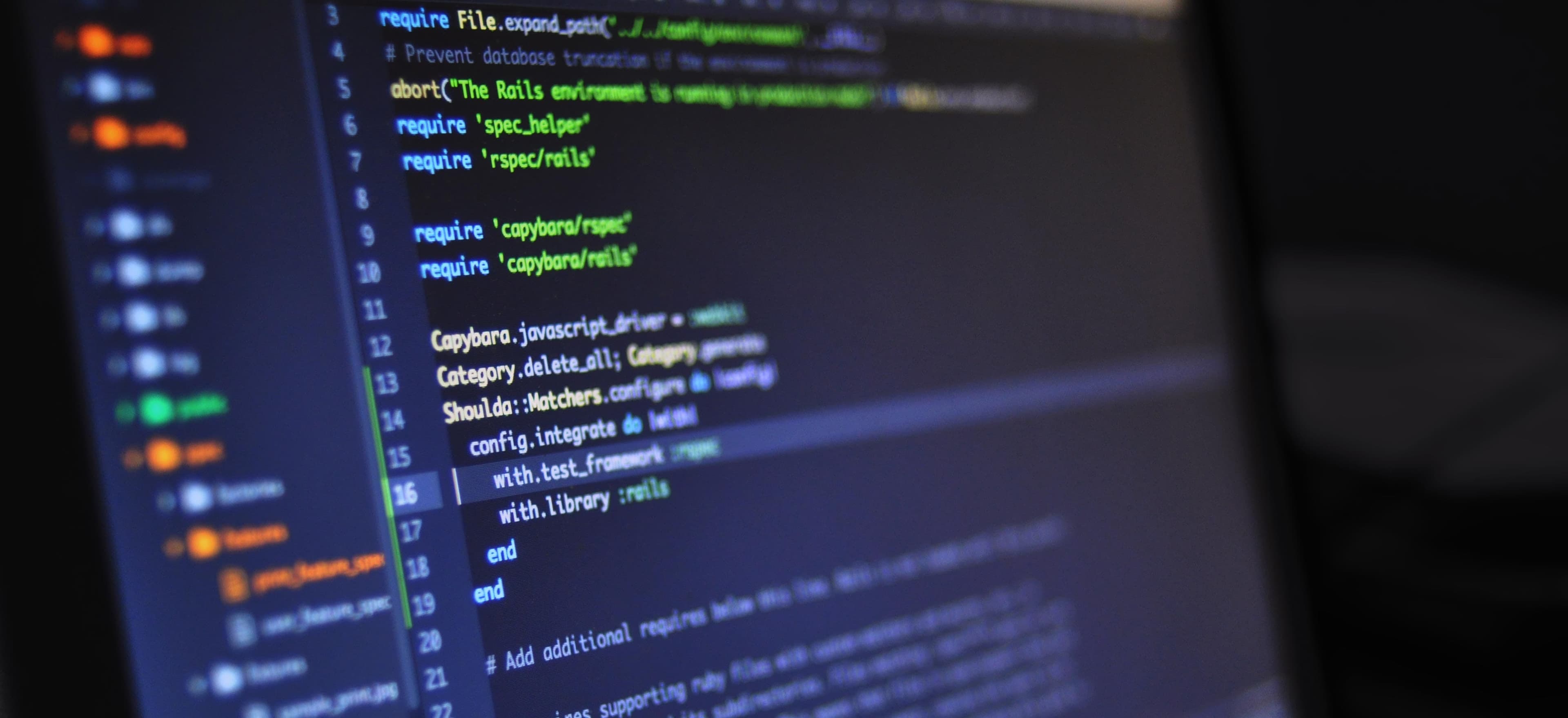
- Published on
Understanding Future Failures in Scala: Common Pitfalls
In the world of Scala, futures provide a powerful way to manage asynchronous programming. They give us the ability to write non-blocking code and improve the performance of our applications. However, as convenient as they are, futures can also introduce a host of potential pitfalls if not handled correctly. This article explores some common pitfalls associated with futures in Scala, discusses their implications, and offers practical solutions that can help you avoid these pitfalls in your own code.
What Are Futures?
In Scala, a Future
is a placeholder for a value that may not yet exist. It allows you to perform non-blocking operations and handle results at a later time. The main advantages of using futures include:
- Non-blocking behavior: The main thread can continue executing while waiting for the future's result.
- Composition: Futures can be combined and transformed easily using combinators like
map
,flatMap
, andfilter
. - Error Handling: Futures come with built-in mechanisms for error handling, simplifying the process of managing exceptions in asynchronous code.
Before diving deeper, make sure you're familiar with the basics of futures in Scala. If you need a refresher, check out the Scala Documentation on Futures.
Common Pitfalls with Futures
1. Ignoring Exceptions
One of the most common pitfalls is neglecting to handle exceptions thrown within future computations. When a future completes with a failure, the error must be properly managed; otherwise, it may lead to unexpected behavior in your application.
Example:
Here's a simple example that demonstrates this issue:
import scala.concurrent.{Future, ExecutionContext}
import scala.util.{Failure, Success}
implicit val ec = ExecutionContext.global
val future = Future {
throw new RuntimeException("Something went wrong!")
}
future.onComplete {
case Success(value) => println(s"Received value: $value")
case Failure(exception) => println(s"Caught exception: ${exception.getMessage}")
}
Why is this important?
Ignoring exceptions can cause your application to crash or enter an unpredictable state. Managing exceptions in futures ensures you can provide fallbacks or notification mechanisms in case of failure, keeping your system more robust.
2. Not Using ExecutionContext Properly
The ExecutionContext
defines the pool of threads that executes futures. By default, Scala provides a global execution context, but it can lead to bottlenecks in I/O-bound applications or when computations are CPU-intensive.
Example:
You may encounter performance issues if you rely solely on the default execution context. Consider defining your custom execution context:
import scala.concurrent.{Future, ExecutionContext}
import java.util.concurrent.Executors
val customThreadPool = Executors.newFixedThreadPool(10)
implicit val ec = ExecutionContext.fromExecutor(customThreadPool)
val future = Future {
// Perform some CPU-bound processing here
1 + 1
}
// Ensure to shut down the thread pool when done
customThreadPool.shutdown()
Why is this important?
Using an appropriate execution context helps you optimize resource usage and application scalability. Always assess your application's needs and choose an execution context accordingly.
3. Not Composing Futures Properly
Composition of futures is one of the core strengths of using futures in Scala, yet improper composition can lead to hard-to-maintain code and unintended behavior. For instance, nesting futures can lead to callback hell.
Example:
Instead of nesting, leverage combinators:
val futureA = Future { 1 }
val futureB = Future { 2 }
// Using flatMap for proper composition
val composedFuture = for {
a <- futureA
b <- futureB
} yield a + b
composedFuture.onComplete {
case Success(result) => println(s"Composed result: $result")
case Failure(exception) => println(s"Caught exception: ${exception.getMessage}")
}
Why is this important?
Properly composing futures using for
-comprehensions or combinators like flatMap
and map
enhances code readability and maintainability. It also helps prevent common issues such as missed failures in inner futures.
4. Blocking the Main Thread
Another frequent pitfall is blocking operations performed on the main thread while waiting for future results. This can negate the advantages of non-blocking I/O provided by futures.
Example:
Avoid using Await.result
unnecessarily:
import scala.concurrent.{Future, Await}
import scala.concurrent.duration._
val future = Future {
Thread.sleep(1000) // Simulating an expensive operation
"Future result"
}
// Blocking the main thread
val result = Await.result(future, 2.seconds)
println(result)
Why is this important?
Blocking defeats the purpose of futures. Instead, utilize callbacks provided by onComplete
, map
, or flatMap
to handle results without blocking.
5. Failing to Handle Cancellation
Futures may represent work that should be cancellable. Neglecting to manage cancellation can lead to resource leaks or lingering processes that still consume resources after their intended purpose is fulfilled.
Example:
Use Promise
for cancellable futures:
import scala.concurrent.{Future, Promise}
import scala.concurrent.ExecutionContext.Implicits.global
val promise = Promise[Int]()
val future = promise.future
// Start a computation and give the option to complete or fail
Future {
Thread.sleep(100) // Simulate some processing
promise.success(42) // completing the Future
}
// Cancelling the future
if (/* some condition */) {
promise.failure(new RuntimeException("Cancelled!"))
}
// Handling cancellation
future.onComplete {
case Success(value) => println(s"Received value: $value")
case Failure(exception) => println(s"Caught exception: ${exception.getMessage}")
}
Why is this important?
Handling future cancellation effectively helps free up resources and improve application responsiveness, especially in cases where users can interrupt long-running tasks.
Final Considerations
Futures in Scala are a powerful tool for managing asynchronous programming. However, to leverage their advantages fully, one must acknowledge and understand common pitfalls, such as ignoring exceptions, improperly managing execution contexts, inefficiently composing futures, blocking threads, and neglecting cancellation.
By avoiding these pitfalls, you will write more resilient, maintainable, and scalable Scala applications. Remember, the checks and balances you maintain in asynchronous coding are crucial to the stability of your entire project.
To enhance your understanding further, consider exploring additional resources such as the official Scala Futures documentation and experiment with various use cases in your projects.
Happy coding!