Optimizing Memory Usage: Minimizing Object Creation
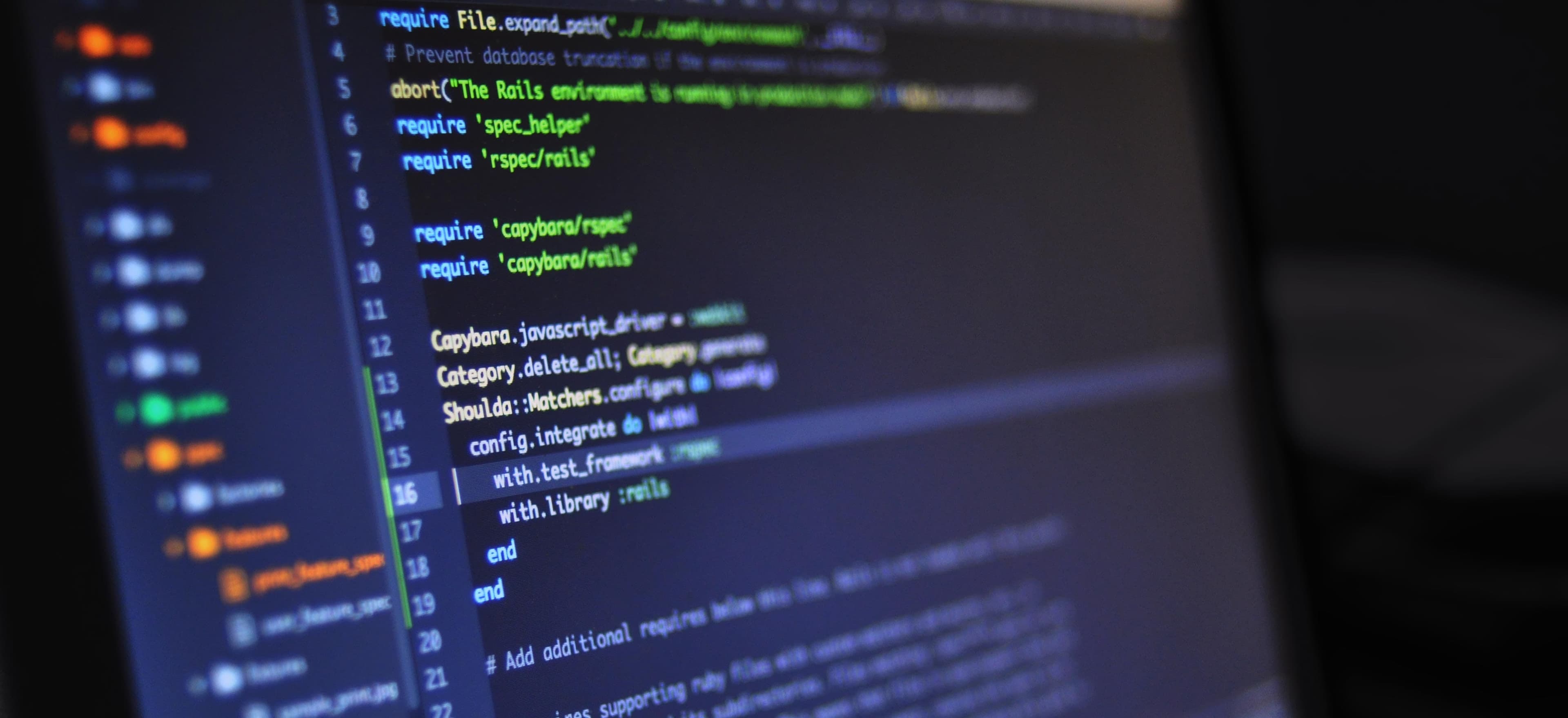
- Published on
Optimizing Memory Usage in Java: Minimizing Object Creation
In Java programming, memory management is a crucial aspect of optimizing the performance of an application. One common approach to improving memory usage is to minimize object creation. Excessive object creation can lead to increased memory consumption, garbage collection overhead, and impact the overall performance of the application. In this article, we will explore the importance of minimizing object creation in Java and discuss some best practices to optimize memory usage.
Understanding Object Creation Overhead
In Java, every object creation comes with an overhead cost. When an object is created, memory allocation, initialization, and garbage collection considerations are involved. This overhead can become significant when dealing with a large number of short-lived objects, such as in tight loops or frequently called methods.
Example 1: Object Creation Overhead
Let's consider a scenario where an object is frequently created within a loop:
List<String> stringList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
String newString = new String("example"); // Object creation
stringList.add(newString);
}
In this example, a new String
object is created in each iteration of the loop. This can lead to unnecessary memory allocation and garbage collection overhead.
Minimizing Object Creation
To minimize object creation and reduce memory overhead, several strategies and best practices can be employed.
1. Reusing Objects
Instead of creating new objects, consider reusing existing objects where possible. Object pooling is a technique where a pool of reusable objects is maintained, and objects are taken from the pool when needed and returned after use.
Example 2: Object Reuse using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.setLength(0); // Clear the StringBuilder
sb.append("example"); // Reuse the StringBuilder
String result = sb.toString(); // Use the StringBuilder content
// Further processing
}
In this example, the StringBuilder
is reused within the loop instead of creating new String
objects.
2. Immutable Objects and Flyweight Pattern
Consider using immutable objects and the flyweight pattern to minimize object creation. Immutable objects, once created, cannot be modified, which enables sharing and reusing the same instance across multiple contexts. The flyweight pattern focuses on sharing objects to support large numbers of fine-grained objects efficiently.
Example 3: Creating Immutable Objects
final class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
// Getters and other methods
}
In this example, the Point
class is immutable, allowing instances to be shared without the risk of modification.
3. Using Static Factory Methods
Static factory methods can be employed to control object creation and reuse. These methods encapsulate the object creation logic and can return pre-existing instances or cache instances for reuse.
Example 4: Static Factory Method for Object Creation
public class ObjectFactory {
private static Map<String, Object> objectCache = new HashMap<>();
public static Object createObject(String key) {
if (objectCache.containsKey(key)) {
return objectCache.get(key); // Reuse existing object
} else {
Object newObject = new Object(); // Create new object
objectCache.put(key, newObject); // Cache the new object
return newObject;
}
}
}
In this example, the ObjectFactory
uses a static factory method to control object creation and reuse by caching instances in a map.
4. Using Primitive Types and Arrays
Where applicable, consider using primitive types and arrays instead of objects and collections. Primitive types and arrays have lower memory overhead compared to their object counterparts.
Example 5: Using Primitive Types and Arrays
int[] intArray = new int[1000]; // Array of primitive ints
In this example, an array of primitive int
s is used instead of an array of Integer
objects.
In Conclusion, Here is What Matters
Minimizing object creation is an essential aspect of optimizing memory usage and improving the performance of Java applications. By reusing objects, using immutable objects, employing static factory methods, and using primitive types and arrays, developers can effectively reduce memory overhead and enhance the efficiency of their applications.
By understanding the overhead of object creation and applying the discussed best practices, developers can optimize memory usage and create efficient Java applications.
For further information on memory management and optimization in Java, Java Platform, Standard Edition HotSpot Virtual Machine Performance Enhancements provides detailed insights into performance enhancements in Java virtual machines.
In conclusion, prioritizing efficient memory usage through minimizing object creation is a fundamental practice for optimizing Java applications and ensuring high-performance execution.