Uncovering the Power of Eclipse Collections
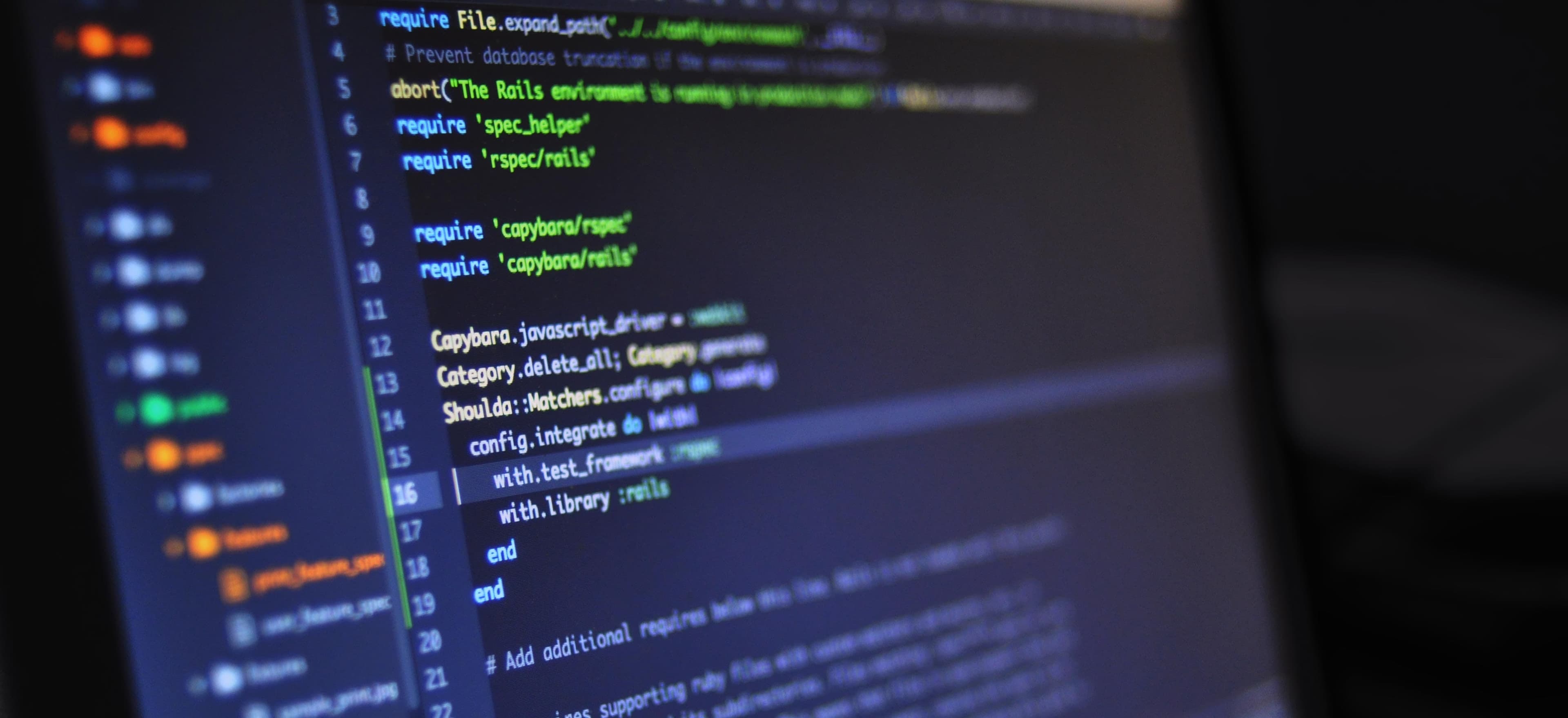
- Published on
Uncovering the Power of Eclipse Collections
Java developers know the power of collections when it comes to handling and organizing data. Eclipse Collections, formerly known as GS Collections, provides a rich and advanced set of collection types and operations to work with data more efficiently and elegantly than the standard Java Collections Framework. In this post, we will explore the benefits and capabilities of Eclipse Collections and understand why it's a valuable addition to a Java developer's toolkit.
What is Eclipse Collections?
Eclipse Collections is an open-source Java library that aims to enhance the Java Collections Framework by providing a wide range of additional collection types, as well as functional programming-style operations for working with collections. It offers a more expressive and powerful way to work with collections, making code more readable, efficient, and less error-prone. Eclipse Collections also provides mutability control, enabling developers to choose between mutable and immutable collections based on their requirements.
Benefits of Eclipse Collections
Enhanced Performance
Eclipse Collections provides specialized collection types that are optimized for specific use cases, which can significantly improve performance compared to using standard Java collections. For example, it offers primitive collections (e.g., IntList
, LongSet
) that can store primitive data types directly without the overhead of auto-boxing, leading to better memory utilization and faster access times.
Fluent API and Functional Style
Eclipse Collections provides a fluent and concise API for working with collections, enabling developers to perform operations such as filtering, mapping, and aggregating in a functional programming style. This leads to more expressive and readable code, reducing the need for explicit iteration and conditional checks.
Immutability Support
Immutability is a key concept in functional programming and concurrent programming. Eclipse Collections promotes immutability by providing immutable collection types and methods to create immutable views of mutable collections. This helps in writing more robust and thread-safe code, as well as preventing unintentional modification of data.
Rich Set of Operations
Eclipse Collections offers a rich set of operations such as filtering, transforming, sorting, and grouping, which allows developers to manipulate and process collections more efficiently. These operations are designed to work seamlessly with the fluent API, enabling complex data transformations to be expressed in a clear and concise manner.
Getting Started with Eclipse Collections
Now, let's dive into some practical examples to understand how Eclipse Collections can be used to solve real-world problems and leverage its unique features.
Basic Operations
Let's start with a simple example of using Eclipse Collections to perform basic operations on a collection. Consider a scenario where we have a list of integers and we want to filter out the even numbers and then double the remaining ones.
MutableList<Integer> numbers = FastList.newListWith(1, 2, 3, 4, 5, 6);
MutableList<Integer> doubledOddNumbers = numbers.select(n -> n % 2 != 0).collect(n -> n * 2);
In this example, we create a MutableList
with some initial integers and then use the select
method to filter out the odd numbers. We then use the collect
method to double each remaining odd number. The resulting list doubledOddNumbers
will contain the doubled odd numbers from the original list.
Grouping and Aggregation
Eclipse Collections provides powerful operations for grouping and aggregation, which can be handy when dealing with complex data processing tasks. Let's consider a case where we have a list of employees and we want to group them by department and calculate the average salary for each department.
MutableList<Employee> employees = ... ; // assuming a list of employees
MutableMap<String, Double> averageSalaryByDepartment = employees.groupBy(Employee::getDepartment, Grouping::collectDouble, Employee::getSalary).collectValuesWith((department, salaries) -> salaries.average());
In this example, we use the groupBy
method to group the employees by their department. We then use the collectDouble
method to collect the salaries as a DoubleList
and calculate the average salary for each department using the average
method. The resulting map averageSalaryByDepartment
will contain the average salary for each department.
Immutable Collections
Immutability is a fundamental concept in functional programming, and Eclipse Collections makes it easy to work with immutable collections. Let's see an example of creating an immutable set of strings using Eclipse Collections.
ImmutableSet<String> immutableSet = Sets.immutable.with("apple", "banana", "orange");
In this example, we use the immutable.with
method to create an immutable set containing the specified strings. Once created, the immutableSet
cannot be modified, ensuring data integrity and thread safety.
Wrapping Up
Eclipse Collections offers a plethora of features and capabilities that can significantly improve the way Java developers work with collections. Its focus on performance, immutability, and fluent API design makes it a valuable addition to any Java developer's toolbox. By leveraging Eclipse Collections, developers can write more expressive, efficient, and robust code, thereby enhancing the quality and maintainability of their Java applications.
If you're interested in learning more about Eclipse Collections, you can explore the official documentation on Eclipse Collections GitHub.
In summary, Eclipse Collections unlocks the true potential of working with collections in Java, providing a powerful and elegant alternative to the standard Java Collections Framework. With its rich set of features and focus on performance and immutability, Eclipse Collections elevates the experience of working with collections and empowers developers to write cleaner, more efficient code.