Error Handling in Java CLI with JewelCLI
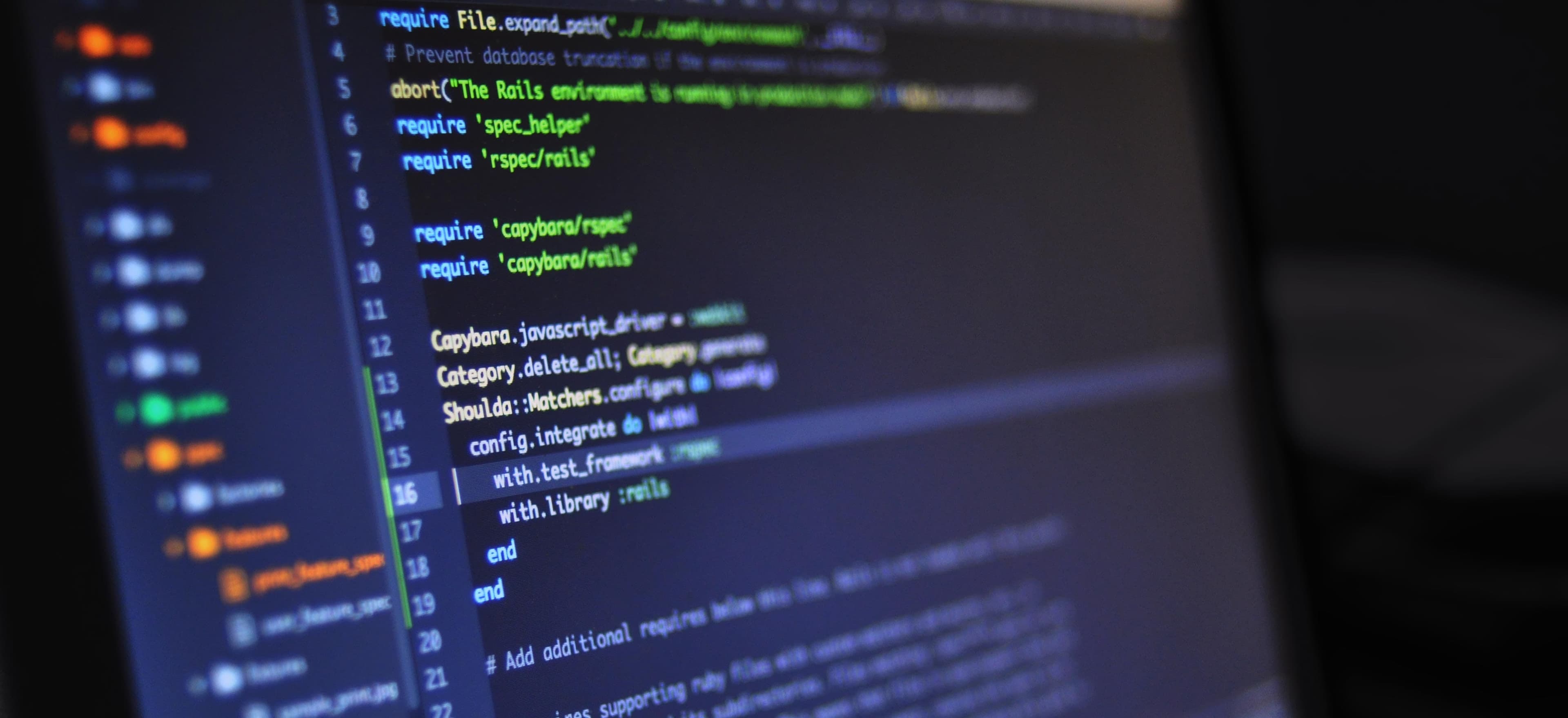
- Published on
Mastering Error Handling in Java CLI with JewelCLI
Creating a Command Line Interface (CLI) in Java can greatly benefit users, but it's essential to implement robust error handling to ensure a smooth experience. JewelCLI is a powerful Java library that simplifies CLI development, and in this guide, we'll explore how to effectively handle errors when building a Java CLI with JewelCLI.
Understanding Error Handling in Java CLI
Error handling is critical in CLI applications to provide users with clear feedback when something goes wrong. In Java, exceptions are commonly used to manage errors, allowing you to gracefully handle exceptional conditions. With JewelCLI, we can leverage its error handling features to enhance the user experience and make our CLI application more reliable.
Setting Up JewelCLI
Before diving into error handling, let's set up JewelCLI in a Java project. You can include JewelCLI in your Maven project by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>com.youdevise</groupId>
<artifactId>jewelcli</artifactId>
<version>0.7.7</version>
</dependency>
After adding the dependency, you can start incorporating JewelCLI into your Java CLI application.
Handling Errors with JewelCLI
JewelCLI simplifies error handling by providing mechanisms to define and handle errors within the CLI application. Below, we'll explore some techniques to effectively handle errors using JewelCLI.
1. Validation Errors
A common source of errors in CLI applications is user input validation. With JewelCLI, you can define custom validators for command-line options, allowing you to enforce specific constraints on user input. For example, to restrict a numeric input to a certain range, you can define a validator as follows:
public class RangeValidator implements OptionValidator<Integer> {
private int min;
private int max;
public RangeValidator(int min, int max) {
this.min = min;
this.max = max;
}
@Override
public void validate(String option, Integer value) throws InvalidOptionValueException {
if (value < min || value > max) {
throw new InvalidOptionValueException(option, String.valueOf(value), "Value must be between " + min + " and " + max);
}
}
}
In the above code snippet, we define a custom validator RangeValidator
that checks if the input value falls within the specified range. If the validation fails, an InvalidOptionValueException
is thrown, providing a clear error message.
2. Exception Handling
JewelCLI allows you to handle exceptions gracefully by providing an OptionException
that encapsulates the details of the error. You can catch and process these exceptions to provide meaningful feedback to the user. For example:
public static void main(String[] args) {
Cli.Builder<Runnable> builder = Cli.builder("mycli")
.withDescription("My CLI Application")
.withDefaultCommand(Help.class)
.withCommands(Help.class, SomeCommand.class);
try {
Cli<Runnable> cli = builder.build();
cli.parse(args).run();
} catch (OptionException e) {
System.err.println("Error: " + e.getMessage());
System.exit(1);
}
}
In the above example, we catch the OptionException
and print a meaningful error message to the standard error output before exiting the application with a non-zero status code.
3. Usage Help and Error Messages
JewelCLI provides built-in support for generating usage help messages and error messages, making it easier to communicate with users. By defining descriptive usage help and error messages, you can ensure that users understand how to interact with the CLI and receive informative feedback when errors occur.
@SomeCommand.Header("My Command")
public class SomeCommand implements Runnable {
@Option(help = "The input file")
private String inputFile;
@Option(help = "The output file")
private String outputFile;
@Override
public void run() {
if (inputFile == null || outputFile == null) {
throw new OptionException("Input and output files are required");
}
// Rest of the command execution logic
}
}
In the above code, we define a meaningful error message using OptionException
to inform users that both input and output files are required.
My Closing Thoughts on the Matter
Mastering error handling in Java CLI applications with JewelCLI is essential for delivering a seamless user experience. By leveraging the error handling mechanisms provided by JewelCLI, you can ensure that your CLI application communicates effectively with users, gracefully handles exceptions, and enforces input validation. This, in turn, fosters user confidence and trust in your CLI application.
In summary, JewelCLI offers a robust framework for error handling, allowing you to focus on building a feature-rich and user-friendly CLI application without compromising on error management.
Enhance your CLI applications with JewelCLI today and empower users with a seamless, error-resilient experience!