The Pitfalls of Optimistic Locking
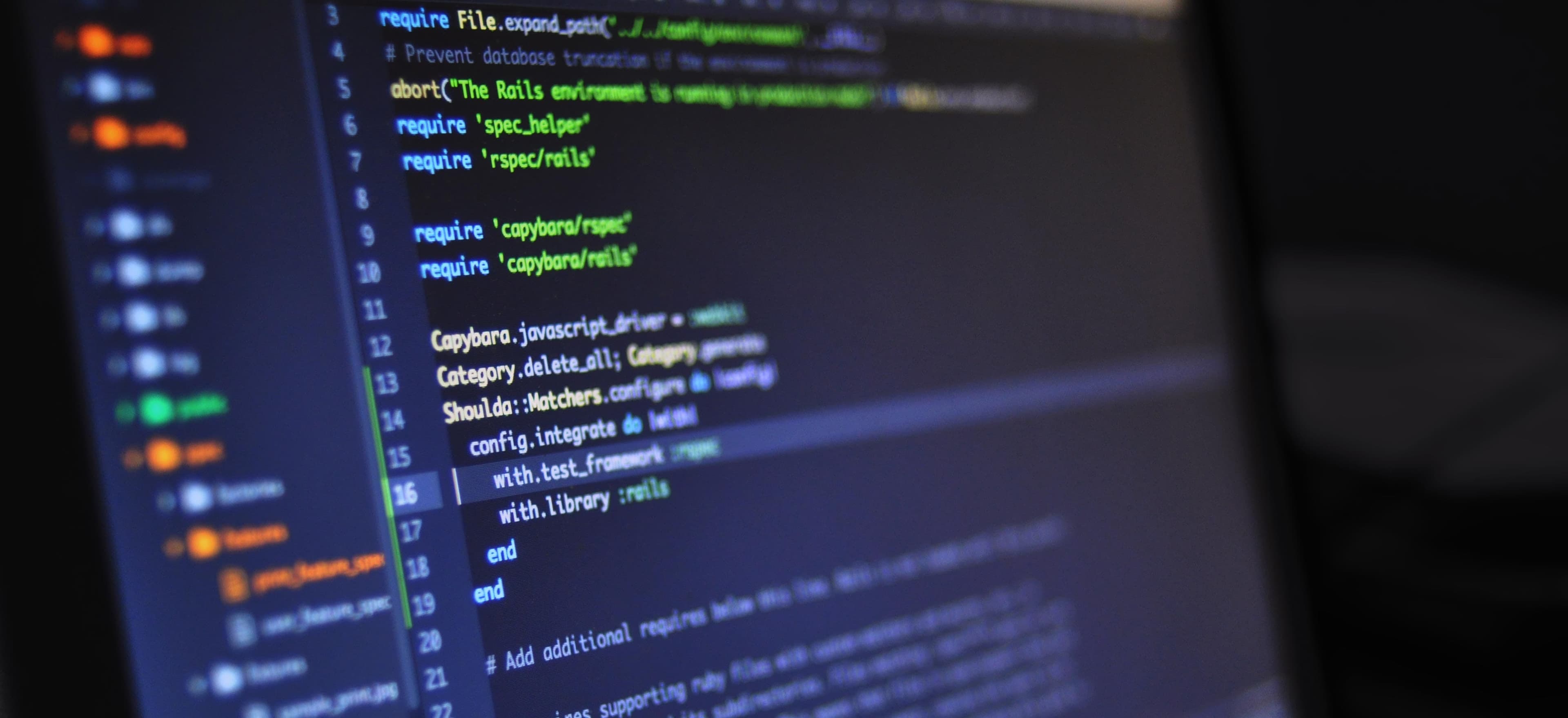
- Published on
The Pitfalls of Optimistic Locking in Java
Optimistic locking is a concurrency control mechanism that allows multiple transactions to access the same data without causing conflicts. In Java, optimistic locking is often used in applications that involve multiple users accessing and modifying shared data.
While optimistic locking can be an effective way to manage concurrent access to data, there are several pitfalls that developers should be aware of when using this approach. In this post, we'll explore some of the common pitfalls of optimistic locking in Java and discuss how to mitigate them.
Pitfall 1: Increased Risk of Data Conflicts
Optimistic locking relies on the assumption that conflicts between transactions are rare. However, in a highly concurrent environment, the risk of data conflicts increases. When multiple transactions attempt to update the same data simultaneously, conflicts can occur, leading to data corruption or inconsistencies.
To mitigate this risk, developers should carefully analyze the data access patterns in their application and consider using a combination of optimistic and pessimistic locking techniques where appropriate. Additionally, implementing proper error handling and conflict resolution mechanisms can help minimize the impact of data conflicts.
@Entity
public class Product {
@Version
private int version;
// other fields and methods
}
In the code above, the @Version
annotation is used in a JPA entity to enable optimistic locking. By including a version field in the entity and annotating it with @Version
, developers can leverage the built-in version-based conflict detection mechanism provided by JPA.
Pitfall 2: Potential Performance Overhead
Optimistic locking typically incurs a performance overhead compared to other locking mechanisms, such as pessimistic locking. This overhead stems from the need to check for conflicts and manage versioning information for each data modification operation.
Developers should be mindful of the potential performance impact of using optimistic locking and consider optimizing their data access and modification routines to minimize this overhead. Techniques such as batched updates, caching, and selective use of locking can help improve the performance of optimistic locking in Java applications.
@Transactional
public void updateProduct(Product product) {
entityManager.merge(product);
}
In the example above, the entityManager.merge()
method is used to update a Product
entity in a transactional context. Under the hood, the JPA provider will perform optimistic locking checks based on the entity's version information to detect and resolve conflicts.
Pitfall 3: Limited Support in Some Data Stores
Not all data stores provide built-in support for optimistic locking. While popular relational databases like MySQL and PostgreSQL offer mechanisms for version-based conflict detection, other types of data stores, such as NoSQL databases, may not natively support optimistic locking.
When working with data stores that lack native support for optimistic locking, developers may need to implement custom conflict detection and resolution logic. This can add complexity to the application code and require careful consideration of the data store's concurrency control capabilities.
Closing the Chapter
Optimistic locking is a powerful concurrency control technique that can help improve the scalability and performance of Java applications. However, it is important for developers to be mindful of the pitfalls associated with optimistic locking and take proactive measures to mitigate them.
By carefully analyzing data access patterns, optimizing performance, and considering the limitations of underlying data stores, developers can effectively leverage optimistic locking in Java applications while minimizing the risk of data conflicts and performance overhead.
In conclusion, while optimistic locking offers numerous benefits, it requires careful consideration and strategic implementation to avoid the potential pitfalls associated with concurrent data access and modification.
For more in-depth information on optimistic locking, check out the official Java documentation and Hibernate Optimistic Locking guide. Happy coding!