Using Java 8 Lambda Expressions for Cleaner Code
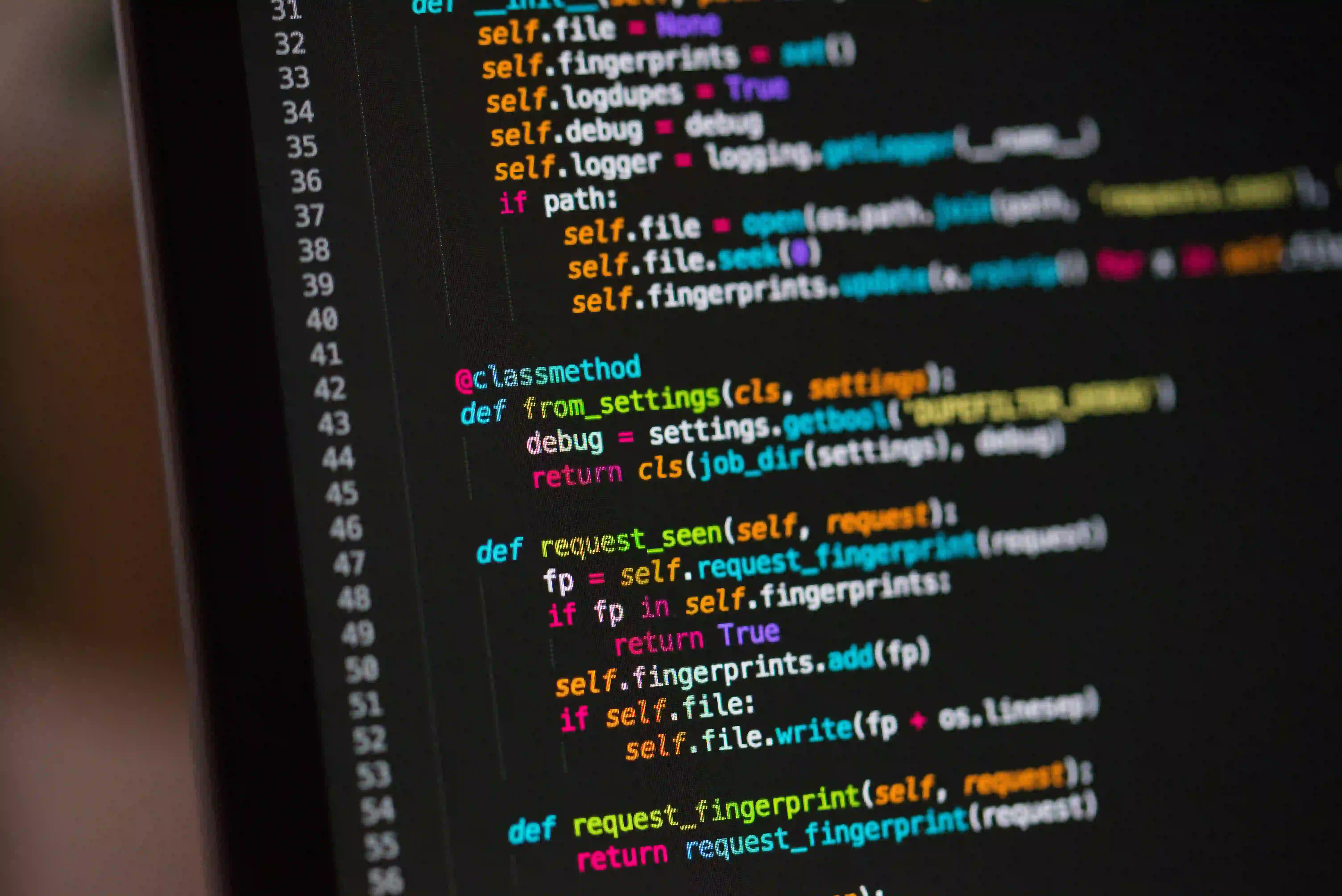
Lambda expressions are a powerful feature introduced in Java 8 that has revolutionized the way we write code. They allow us to treat functionality as a method argument, or code as data. This enables a more functional style of programming in Java, making our code more concise and readable.
What are Lambda Expressions?
In simple terms, a lambda expression is an anonymous function that allows you to treat functionality as if it were an argument to a method. It is characterized by the ->
symbol, which separates the method parameters from the body of the lambda expression.
Why Use Lambda Expressions?
-
Concise Code: Lambda expressions enable us to write shorter and more readable code by removing the need for verbose anonymous inner classes.
-
Easier to Use with Collections: They make it easier to work with collections by providing a more functional and expressive way to iterate through, filter, and transform elements.
-
Enable Functional Programming: Lambda expressions bring a functional programming style to Java, allowing us to write more declarative and expressive code.
-
Parallel Processing: With the introduction of the Streams API, lambda expressions make it easier to perform operations in parallel, improving the performance of our code.
Syntax of Lambda Expressions
The syntax for a lambda expression is as follows:
(parameters) -> expression
or
(parameters) -> { statements; }
Where:
parameters
are the input parameters to the lambda expression.->
denotes the separation between parameters and body.expression
or{ statements; }
represent the body of the lambda.
Let's look at a simple example to illustrate the syntax of a lambda expression.
Example 1: Simple Lambda Expression
Suppose we have a functional interface MathOperation
which has a method int operation(int a, int b)
. We can use a lambda expression to implement this interface as follows:
interface MathOperation {
int operation(int a, int b);
}
public class LambdaExample {
public static void main(String[] args) {
MathOperation addition = (a, b) -> a + b;
System.out.println("Addition: " + addition.operation(10, 20));
}
}
In this example, (a, b) -> a + b
is a lambda expression that implements the operation
method of the MathOperation
interface. It takes two integer parameters a
and b
and returns their sum.
Functional Interfaces
In Java, a functional interface is an interface that contains only one abstract method. Lambda expressions can only be used with functional interfaces, and they provide the implementation of the single abstract method defined by the functional interface.
Example 2: Using Predefined Functional Interface
Java 8 provides a set of predefined functional interfaces in the java.util.function
package, such as Predicate
, Function
, Consumer
, and Supplier
.
Let's look at an example using the Predicate
functional interface:
import java.util.function.Predicate;
public class PredicateExample {
public static void main(String[] args) {
Predicate<Integer> isEven = num -> num % 2 == 0;
System.out.println("Is 10 even? " + isEven.test(10));
System.out.println("Is 15 even? " + isEven.test(15));
}
}
In this example, num -> num % 2 == 0
is a lambda expression that implements the test
method of the Predicate
functional interface. It checks if the given number is even.
Method References
In addition to lambda expressions, Java 8 introduces method references, which are a shorthand notation for a lambda expression to call a method. Method references can make your code even more concise and readable.
There are four types of method references:
- Static Method Reference:
ContainingClass::staticMethodName
- Instance Method Reference of a Particular Object:
object::instanceMethodName
- Instance Method Reference of an Arbitrary Object of a Particular Type:
ContainingType::methodName
- Constructor Reference:
ClassName::new
Example 3: Using Method References
Let's see an example of using a static method reference:
import java.util.function.Function;
public class MethodReferenceExample {
public static void main(String[] args) {
Function<String, Integer> parseInt = Integer::parseInt;
System.out.println(parseInt.apply("123")); // Output: 123
}
}
In this example, Integer::parseInt
is a static method reference that converts a String
to an Integer
.
Summary
Java 8 lambda expressions and method references have made the language more expressive and concise. They have simplified the way we write code, especially in scenarios where we want to pass behavior around or execute code in response to events.
By leveraging lambda expressions, you can write cleaner and more readable code, take advantage of functional programming paradigms, and work more effectively with collections and streams.
So, whether you are working with functional interfaces, method references, or the Streams API, Java 8's lambda expressions enable you to write cleaner and more expressive code. Embracing functional programming paradigms and leveraging lambda expressions will lead to a more powerful and concise codebase for your Java projects.
Start using lambda expressions today to elevate the quality of your Java code, and make the most of this powerful and expressive feature introduced in Java 8.